How to Return Array in Java
- Return an Array of Different Data Types From a Function in Java
- Return an Array From a Class Object in Java
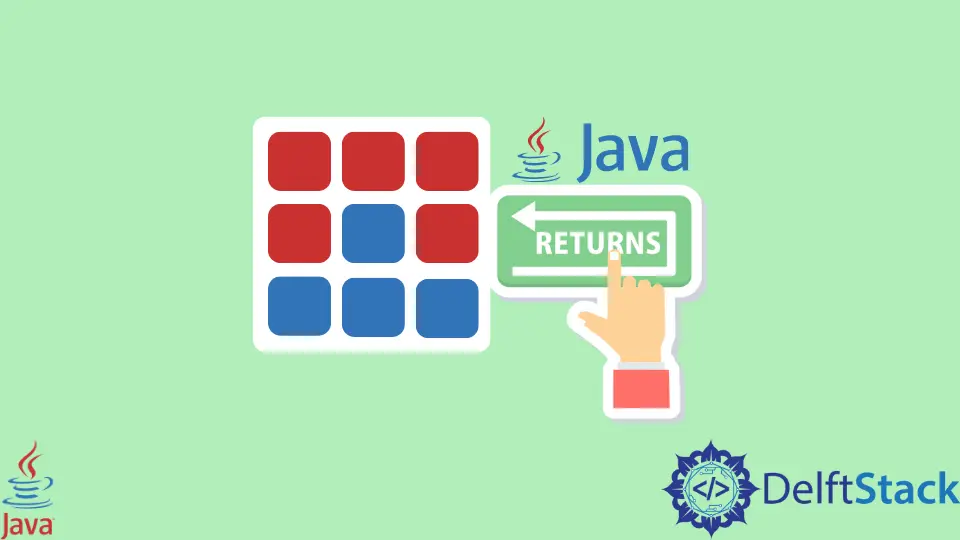
This article introduces how we can return an array in Java from a function or a class object.
Return an Array of Different Data Types From a Function in Java
We can initialize an array with the elements to return them from the function. In the following example, we have four functions with different return types like int
, double
, String
, and boolean
. We initialize a new array to return it with the function. To create a new array, we use the new
keyword and the data type of the array.
new int[]
creates a new array of integers with the items and the same goes for other data types. Now we have to receive the returned value in a String
to print it in the output. To do this, we use Arrays.toString()
that takes an array as the only argument and converts the array to a string.
import java.util.Arrays;
public class ReturnAnArray {
public static void main(String[] args) {
String intArrayAsString = Arrays.toString(returnArrayInt());
String doubleArrayAsString = Arrays.toString(returnArrayDouble());
String arrayAsString = Arrays.toString(returnArrayString());
String booleanAsString = Arrays.toString(returnArrayBoolean());
System.out.println("Returned Integer Array: " + intArrayAsString);
System.out.println("Returned Double Array: " + doubleArrayAsString);
System.out.println("Returned String Array: " + arrayAsString);
System.out.println("Returned Boolean Array: " + booleanAsString);
}
static int[] returnArrayInt() {
return new int[] {1, 3, 6, 8, 10};
}
static double[] returnArrayDouble() {
return new double[] {1.0, 2.4, 5.7};
}
static String[] returnArrayString() {
return new String[] {"One", "Two", "Three", "Four"};
}
static boolean[] returnArrayBoolean() {
return new boolean[] {true, false, true, false};
}
}
Output:
Returned Integer Array: [1, 3, 6, 8, 10]
Returned Double Array: [1.0, 2.4, 5.7]
Returned String Array: [One, Two, Three, Four]
Returned Boolean Array: [true, false, true, false]
Return an Array From a Class Object in Java
To return an array from a class, we need a class ArrayReturningClass
and a function inside it createNewArray
, that returns an array, the return type in our case is int
. In createNewArray
, we create a new array and initialize it with some integer values. At last, we return it using return newArray
.
We create an object of the ArrayReturningClass
class and access the createNewArray()
function. We now have the returned array in returnedArray
that we can convert into a String
using Arrays.toString()
.
import java.util.Arrays;
public class ReturnAnArray {
public static void main(String[] args) {
ArrayReturningClass arrayReturningClass = new ArrayReturningClass();
int[] returnedArray = arrayReturningClass.createNewArray();
String intArrayAsString = Arrays.toString(returnedArray);
System.out.println(intArrayAsString);
}
}
class ArrayReturningClass {
public int[] createNewArray() {
int[] newArray = {10, 20, 40, 50};
return newArray;
}
}
Output:
[10, 20, 40, 50]
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn