How to Read XML File in Java
- Sample XML File
-
Read XML File Using
DocumentBuilderFactory
in Java - Read XML File in Java POJO
-
Read XML File Using
jdom2
in Java -
Read XML File Using
XPath
in Java -
Read XML File Using
DOM4J
in Java
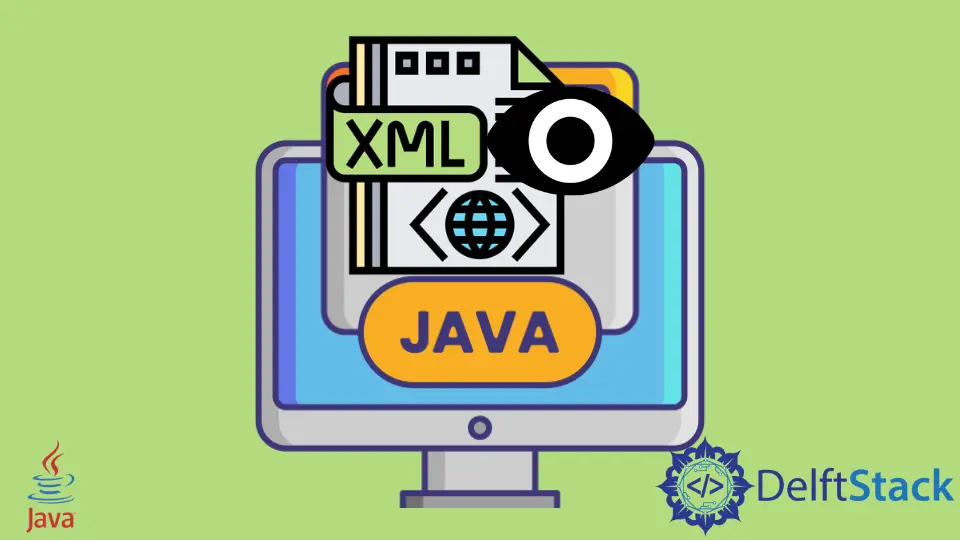
In this article, we will learn how to parse XML file into Java application by using some libraries such as DOM Parser
, XML Parser
, jdom2
, dom4j
, etc. XML stands for Extensible Markup Language
, which is used to pass data in an application.
Sample XML File
This is a sample XML file that will be used to read in this article.
<?xml version="1.0"?>
<company>
<employee id="1001">
<firstname>Tony</firstname>
<lastname>Black</lastname>
<salary>100000</salary>
</employee>
<employee id="2001">
<firstname>Amy</firstname>
<lastname>Green</lastname>
<salary>200000</salary>
</employee>
</company>
Read XML File Using DocumentBuilderFactory
in Java
We use DocumentBuilder
to create an instance for the builder and then parse the XML file using the parse()
method. The getElementsByTagName()
method fetches each node of the XML, and then each child node of the node is iterated by using the for
loop. See the example below.
import java.io.File;
import java.io.IOException;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.xml.sax.SAXException;
public class SimpleTesting {
public static void main(String[] args) throws ParserConfigurationException, SAXException {
try {
File file = new File("company.xml");
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
DocumentBuilder db = dbf.newDocumentBuilder();
Document document = db.parse(file);
document.getDocumentElement().normalize();
System.out.println("Root Element :" + document.getDocumentElement().getNodeName());
NodeList nList = document.getElementsByTagName("employee");
System.out.println("----------------------------");
for (int temp = 0; temp < nList.getLength(); temp++) {
Node nNode = nList.item(temp);
System.out.println("\nCurrent Element :" + nNode.getNodeName());
if (nNode.getNodeType() == Node.ELEMENT_NODE) {
Element eElement = (Element) nNode;
System.out.println("Employee id : " + eElement.getAttribute("id"));
System.out.println("First Name : "
+ eElement.getElementsByTagName("firstname").item(0).getTextContent());
System.out.println(
"Last Name : " + eElement.getElementsByTagName("lastname").item(0).getTextContent());
System.out.println(
"Salary : " + eElement.getElementsByTagName("salary").item(0).getTextContent());
}
}
} catch (IOException e) {
System.out.println(e);
}
}
}
Output:
Root Element :company
----------------------------
Current Element :employee
Employee id : 1001
First Name : Tony
Last Name : Black
Salary : 100000
Current Element :employee
Employee id : 2001
First Name : Amy
Last Name : Green
Salary : 200000
Read XML File in Java POJO
In case we want to get XML data into Java-compatible type, we can use Java POJO to read data. Here, we use the ArrayList
type Employee to add each node using the add()
method and then use the for
loop to iterate each object. See the below example.
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.xml.sax.SAXException;
public class Main {
public static void main(String[] args)
throws ParserConfigurationException, SAXException, IOException {
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
Document document = builder.parse(new File("employee.xml"));
List<Employee> employees = new ArrayList<>();
NodeList nodeList = document.getDocumentElement().getChildNodes();
for (int i = 0; i < nodeList.getLength(); i++) {
Node node = nodeList.item(i);
if (node.getNodeType() == Node.ELEMENT_NODE) {
Element elem = (Element) node;
String firstname =
elem.getElementsByTagName("firstname").item(0).getChildNodes().item(0).getNodeValue();
String lastname =
elem.getElementsByTagName("lastname").item(0).getChildNodes().item(0).getNodeValue();
Double salary = Double.parseDouble(
elem.getElementsByTagName("salary").item(0).getChildNodes().item(0).getNodeValue());
employees.add(new Employee(firstname, lastname, salary));
}
}
for (Employee empl : employees) System.out.println(empl.toString());
}
}
class Employee {
private String Firstname;
private String Lastname;
private double salary;
public Employee(String Firstname, String Lastname, double salary) {
this.Firstname = Firstname;
this.Lastname = Lastname;
this.salary = salary;
}
@Override
public String toString() {
return "[" + Firstname + ", " + Lastname + ", " + salary + "]";
}
}
Output:
[Tony, Black, 100000.0]
[Amy, Green, 200000.0]
Read XML File Using jdom2
in Java
The jdom2 is a library that provides support of DOM parsing using Java classes. We used SAXBuilder
class and build()
method to get data into Document
and then by using the getRootElement()
method, get the elements. See the example below.
import java.io.File;
import java.io.IOException;
import java.util.List;
import javax.xml.parsers.ParserConfigurationException;
import org.jdom2.Document;
import org.jdom2.Element;
import org.jdom2.JDOMException;
import org.jdom2.input.SAXBuilder;
import org.xml.sax.SAXException;
public class Main {
public static void main(String[] args)
throws ParserConfigurationException, SAXException, IOException {
try {
File inputFile = new File("/employee.xml");
SAXBuilder saxBuilder = new SAXBuilder();
Document document = saxBuilder.build(inputFile);
System.out.println("Root element :" + document.getRootElement().getName());
Element classElement = document.getRootElement();
List<Element> studentList = classElement.getChildren();
System.out.println("----------------------------");
for (int temp = 0; temp < studentList.size(); temp++) {
Element student = studentList.get(temp);
System.out.println("\nCurrent Element :" + student.getName());
System.out.println("First Name : " + student.getChild("firstname").getText());
System.out.println("Last Name : " + student.getChild("lastname").getText());
System.out.println("Salary : " + student.getChild("salary").getText());
}
} catch (JDOMException e) {
e.printStackTrace();
} catch (IOException ioe) {
ioe.printStackTrace();
}
}
}
Output:
Root element :company
----------------------------
Current Element :employee
First Name : Tony
Last Name : Black
Salary : 100000
Current Element :employee
First Name : Amy
Last Name : Green
Salary : 200000
Read XML File Using XPath
in Java
Here, we use the XPath
library to parse XML files in Java. The XPathFactory
class is used to compile all the nodes into NodeList
and then iterate each child through for loop. See the example below.
import java.io.File;
import java.io.IOException;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import javax.xml.xpath.XPath;
import javax.xml.xpath.XPathConstants;
import javax.xml.xpath.XPathExpressionException;
import javax.xml.xpath.XPathFactory;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.xml.sax.SAXException;
public class Main {
public static void main(String[] args)
throws ParserConfigurationException, SAXException, IOException {
try {
File inputFile = new File("/employee.xml");
DocumentBuilderFactory dbFactory = DocumentBuilderFactory.newInstance();
DocumentBuilder dBuilder;
dBuilder = dbFactory.newDocumentBuilder();
Document doc = dBuilder.parse(inputFile);
doc.getDocumentElement().normalize();
XPath xPath = XPathFactory.newInstance().newXPath();
String expression = "/company/employee";
NodeList nodeList =
(NodeList) xPath.compile(expression).evaluate(doc, XPathConstants.NODESET);
for (int i = 0; i < nodeList.getLength(); i++) {
Node nNode = nodeList.item(i);
System.out.println("\nCurrent Element :" + nNode.getNodeName());
if (nNode.getNodeType() == Node.ELEMENT_NODE) {
Element eElement = (Element) nNode;
System.out.println("First Name : "
+ eElement.getElementsByTagName("firstname").item(0).getTextContent());
System.out.println(
"Last Name : " + eElement.getElementsByTagName("lastname").item(0).getTextContent());
System.out.println(
"Salary : " + eElement.getElementsByTagName("salary").item(0).getTextContent());
}
}
} catch (ParserConfigurationException e) {
System.out.println(e);
} catch (SAXException e) {
System.out.println(e);
} catch (IOException e) {
System.out.println(e);
} catch (XPathExpressionException e) {
System.out.println(e);
}
}
}
Output:
Current Element :employee
First Name : Tony
Last Name : Black
Salary : 100000
Current Element :employee
First Name : Amy
Last Name : Green
Salary : 200000
Read XML File Using DOM4J
in Java
The Dom4j
is another library that can parse XML files in Java. The read()
method of SAXReader class is used to read nodes into the document. See the example below.
import java.io.File;
import java.io.IOException;
import java.util.List;
import javax.xml.parsers.ParserConfigurationException;
import org.dom4j.Document;
import org.dom4j.DocumentException;
import org.dom4j.Element;
import org.dom4j.Node;
import org.dom4j.io.SAXReader;
import org.xml.sax.SAXException;
public class Main {
public static void main(String[] args)
throws ParserConfigurationException, SAXException, IOException {
try {
File inputFile = new File("employee.xml");
SAXReader reader = new SAXReader();
Document document = reader.read(inputFile);
System.out.println("Root element :" + document.getRootElement().getName());
Element classElement = document.getRootElement();
List<Node> nodes = document.selectNodes("company/employee");
System.out.println("----------------------------");
for (Node node : nodes) {
System.out.println("\nCurrent Element :" + node.getName());
System.out.println("First Name : " + node.selectSingleNode("firstname").getText());
System.out.println("Last Name : " + node.selectSingleNode("lastname").getText());
System.out.println("Salary : " + node.selectSingleNode("salary").getText());
}
} catch (DocumentException e) {
e.printStackTrace();
}
}
}
Output:
Root element :company
----------------------------
Current Element :employee
First Name : Tony
Last Name : Black
Salary : 100000
Current Element :employee
First Name : Amy
Last Name : Green
Salary : 200000