Comment lire un fichier XML en Java
- Exemple de fichier XML
-
Lire un fichier XML à l’aide de
DocumentBuilderFactory
en Java - Lire le fichier XML dans Java POJO
-
Lire un fichier XML en utilisant
jdom2
en Java -
Lire un fichier XML en utilisant
XPath
en Java -
Lire un fichier XML en utilisant
DOM4J
en Java
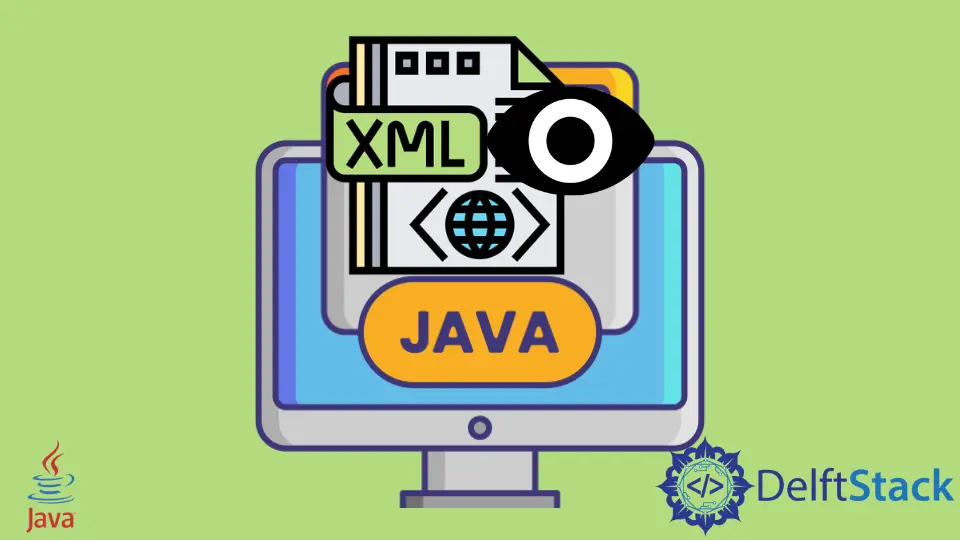
Dans cet article, nous allons apprendre à analyser un fichier XML dans une application Java en utilisant certaines bibliothèques telles que DOM Parser
, XML Parser
, jdom2
, dom4j
, etc. XML signifie Extensible Markup Language
, qui est utilisé pour transmettre des données dans une application.
Exemple de fichier XML
Ceci est un exemple de fichier XML qui sera utilisé pour lire dans cet article.
<?xml version="1.0"?>
<company>
<employee id="1001">
<firstname>Tony</firstname>
<lastname>Black</lastname>
<salary>100000</salary>
</employee>
<employee id="2001">
<firstname>Amy</firstname>
<lastname>Green</lastname>
<salary>200000</salary>
</employee>
</company>
Lire un fichier XML à l’aide de DocumentBuilderFactory
en Java
Nous utilisons DocumentBuilder
pour créer une instance pour le constructeur puis analysons le fichier XML en utilisant la méthode parse()
. La méthode getElementsByTagName()
récupère chaque nœud du XML, puis chaque nœud enfant du nœud est itéré en utilisant la boucle for
. Voir l’exemple ci-dessous.
import java.io.File;
import java.io.IOException;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.xml.sax.SAXException;
public class SimpleTesting {
public static void main(String[] args) throws ParserConfigurationException, SAXException {
try {
File file = new File("company.xml");
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
DocumentBuilder db = dbf.newDocumentBuilder();
Document document = db.parse(file);
document.getDocumentElement().normalize();
System.out.println("Root Element :" + document.getDocumentElement().getNodeName());
NodeList nList = document.getElementsByTagName("employee");
System.out.println("----------------------------");
for (int temp = 0; temp < nList.getLength(); temp++) {
Node nNode = nList.item(temp);
System.out.println("\nCurrent Element :" + nNode.getNodeName());
if (nNode.getNodeType() == Node.ELEMENT_NODE) {
Element eElement = (Element) nNode;
System.out.println("Employee id : " + eElement.getAttribute("id"));
System.out.println("First Name : "
+ eElement.getElementsByTagName("firstname").item(0).getTextContent());
System.out.println(
"Last Name : " + eElement.getElementsByTagName("lastname").item(0).getTextContent());
System.out.println(
"Salary : " + eElement.getElementsByTagName("salary").item(0).getTextContent());
}
}
} catch (IOException e) {
System.out.println(e);
}
}
}
Production:
Root Element :company
----------------------------
Current Element :employee
Employee id : 1001
First Name : Tony
Last Name : Black
Salary : 100000
Current Element :employee
Employee id : 2001
First Name : Amy
Last Name : Green
Salary : 200000
Lire le fichier XML dans Java POJO
Si nous voulons obtenir des données XML dans un type compatible Java, nous pouvons utiliser Java POJO pour lire les données. Ici, nous utilisons le type Employé de type ArrayList
pour ajouter chaque nœud à l’aide de la méthode add()
puis nous utilisons la boucle for
pour itérer chaque objet. Voir l’exemple ci-dessous.
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.xml.sax.SAXException;
public class Main {
public static void main(String[] args)
throws ParserConfigurationException, SAXException, IOException {
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
Document document = builder.parse(new File("employee.xml"));
List<Employee> employees = new ArrayList<>();
NodeList nodeList = document.getDocumentElement().getChildNodes();
for (int i = 0; i < nodeList.getLength(); i++) {
Node node = nodeList.item(i);
if (node.getNodeType() == Node.ELEMENT_NODE) {
Element elem = (Element) node;
String firstname =
elem.getElementsByTagName("firstname").item(0).getChildNodes().item(0).getNodeValue();
String lastname =
elem.getElementsByTagName("lastname").item(0).getChildNodes().item(0).getNodeValue();
Double salary = Double.parseDouble(
elem.getElementsByTagName("salary").item(0).getChildNodes().item(0).getNodeValue());
employees.add(new Employee(firstname, lastname, salary));
}
}
for (Employee empl : employees) System.out.println(empl.toString());
}
}
class Employee {
private String Firstname;
private String Lastname;
private double salary;
public Employee(String Firstname, String Lastname, double salary) {
this.Firstname = Firstname;
this.Lastname = Lastname;
this.salary = salary;
}
@Override
public String toString() {
return "[" + Firstname + ", " + Lastname + ", " + salary + "]";
}
}
Production:
[Tony, Black, 100000.0]
[Amy, Green, 200000.0]
Lire un fichier XML en utilisant jdom2
en Java
Jdom2 est une bibliothèque qui prend en charge l’analyse DOM à l’aide de classes Java. Nous avons utilisé la classe SAXBuilder
et la méthode build()
pour récupérer les données dans Document
puis en utilisant la méthode getRootElement()
, obtenir les éléments. Voir l’exemple ci-dessous.
import java.io.File;
import java.io.IOException;
import java.util.List;
import javax.xml.parsers.ParserConfigurationException;
import org.jdom2.Document;
import org.jdom2.Element;
import org.jdom2.JDOMException;
import org.jdom2.input.SAXBuilder;
import org.xml.sax.SAXException;
public class Main {
public static void main(String[] args)
throws ParserConfigurationException, SAXException, IOException {
try {
File inputFile = new File("/employee.xml");
SAXBuilder saxBuilder = new SAXBuilder();
Document document = saxBuilder.build(inputFile);
System.out.println("Root element :" + document.getRootElement().getName());
Element classElement = document.getRootElement();
List<Element> studentList = classElement.getChildren();
System.out.println("----------------------------");
for (int temp = 0; temp < studentList.size(); temp++) {
Element student = studentList.get(temp);
System.out.println("\nCurrent Element :" + student.getName());
System.out.println("First Name : " + student.getChild("firstname").getText());
System.out.println("Last Name : " + student.getChild("lastname").getText());
System.out.println("Salary : " + student.getChild("salary").getText());
}
} catch (JDOMException e) {
e.printStackTrace();
} catch (IOException ioe) {
ioe.printStackTrace();
}
}
}
Production:
Root element :company
----------------------------
Current Element :employee
First Name : Tony
Last Name : Black
Salary : 100000
Current Element :employee
First Name : Amy
Last Name : Green
Salary : 200000
Lire un fichier XML en utilisant XPath
en Java
Ici, nous utilisons la bibliothèque XPath
pour analyser les fichiers XML en Java. La classe XPathFactory
est utilisée pour compiler tous les nœuds dans NodeList
puis itérer chaque enfant à travers la boucle for. Voir l’exemple ci-dessous.
import java.io.File;
import java.io.IOException;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import javax.xml.xpath.XPath;
import javax.xml.xpath.XPathConstants;
import javax.xml.xpath.XPathExpressionException;
import javax.xml.xpath.XPathFactory;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.xml.sax.SAXException;
public class Main {
public static void main(String[] args)
throws ParserConfigurationException, SAXException, IOException {
try {
File inputFile = new File("/employee.xml");
DocumentBuilderFactory dbFactory = DocumentBuilderFactory.newInstance();
DocumentBuilder dBuilder;
dBuilder = dbFactory.newDocumentBuilder();
Document doc = dBuilder.parse(inputFile);
doc.getDocumentElement().normalize();
XPath xPath = XPathFactory.newInstance().newXPath();
String expression = "/company/employee";
NodeList nodeList =
(NodeList) xPath.compile(expression).evaluate(doc, XPathConstants.NODESET);
for (int i = 0; i < nodeList.getLength(); i++) {
Node nNode = nodeList.item(i);
System.out.println("\nCurrent Element :" + nNode.getNodeName());
if (nNode.getNodeType() == Node.ELEMENT_NODE) {
Element eElement = (Element) nNode;
System.out.println("First Name : "
+ eElement.getElementsByTagName("firstname").item(0).getTextContent());
System.out.println(
"Last Name : " + eElement.getElementsByTagName("lastname").item(0).getTextContent());
System.out.println(
"Salary : " + eElement.getElementsByTagName("salary").item(0).getTextContent());
}
}
} catch (ParserConfigurationException e) {
System.out.println(e);
} catch (SAXException e) {
System.out.println(e);
} catch (IOException e) {
System.out.println(e);
} catch (XPathExpressionException e) {
System.out.println(e);
}
}
}
Production:
Current Element :employee
First Name : Tony
Last Name : Black
Salary : 100000
Current Element :employee
First Name : Amy
Last Name : Green
Salary : 200000
Lire un fichier XML en utilisant DOM4J
en Java
Le Dom4j
est une autre bibliothèque qui permet d’analyser les fichiers XML en Java. La méthode read()
de la classe SAXReader est utilisée pour lire les noeuds dans le document. Voir l’exemple ci-dessous.
import java.io.File;
import java.io.IOException;
import java.util.List;
import javax.xml.parsers.ParserConfigurationException;
import org.dom4j.Document;
import org.dom4j.DocumentException;
import org.dom4j.Element;
import org.dom4j.Node;
import org.dom4j.io.SAXReader;
import org.xml.sax.SAXException;
public class Main {
public static void main(String[] args)
throws ParserConfigurationException, SAXException, IOException {
try {
File inputFile = new File("employee.xml");
SAXReader reader = new SAXReader();
Document document = reader.read(inputFile);
System.out.println("Root element :" + document.getRootElement().getName());
Element classElement = document.getRootElement();
List<Node> nodes = document.selectNodes("company/employee");
System.out.println("----------------------------");
for (Node node : nodes) {
System.out.println("\nCurrent Element :" + node.getName());
System.out.println("First Name : " + node.selectSingleNode("firstname").getText());
System.out.println("Last Name : " + node.selectSingleNode("lastname").getText());
System.out.println("Salary : " + node.selectSingleNode("salary").getText());
}
} catch (DocumentException e) {
e.printStackTrace();
}
}
}
Production:
Root element :company
----------------------------
Current Element :employee
First Name : Tony
Last Name : Black
Salary : 100000
Current Element :employee
First Name : Amy
Last Name : Green
Salary : 200000