Come leggere file XML in Java
- File XML di esempio
-
Leggere il file XML utilizzando
DocumentBuilderFactory
in Java - Leggi il file XML in Java POJO
-
Leggere il file XML utilizzando
jdom2
in Java -
Leggere il file XML utilizzando
XPath
in Java -
Leggere il file XML utilizzando
DOM4J
in Java
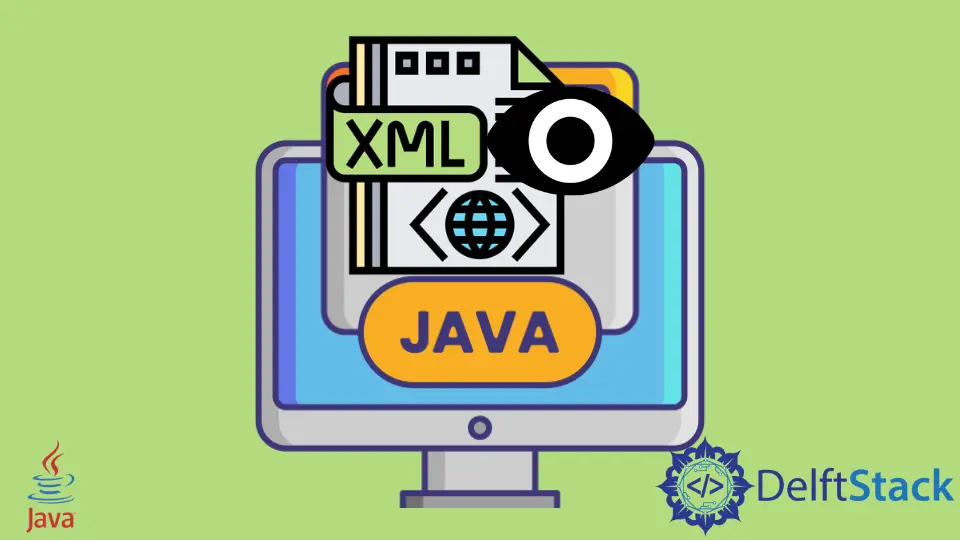
In questo articolo impareremo come analizzare il file XML in un’applicazione Java utilizzando alcune librerie come DOM Parser
, XML Parser
, jdom2
, dom4j
, ecc. XML sta per Extensible Markup Language
, che viene utilizzato per passare i dati in un’applicazione.
File XML di esempio
Questo è un file XML di esempio che verrà utilizzato per leggere in questo articolo.
<?xml version="1.0"?>
<company>
<employee id="1001">
<firstname>Tony</firstname>
<lastname>Black</lastname>
<salary>100000</salary>
</employee>
<employee id="2001">
<firstname>Amy</firstname>
<lastname>Green</lastname>
<salary>200000</salary>
</employee>
</company>
Leggere il file XML utilizzando DocumentBuilderFactory
in Java
Usiamo DocumentBuilder
per creare un’istanza per il builder e poi analizzare il file XML usando il metodo parse()
. Il metodo getElementsByTagName()
recupera ogni nodo dell’XML, quindi ogni nodo figlio del nodo viene iterato utilizzando il cicli for
. Vedi l’esempio sotto.
import java.io.File;
import java.io.IOException;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.xml.sax.SAXException;
public class SimpleTesting {
public static void main(String[] args) throws ParserConfigurationException, SAXException {
try {
File file = new File("company.xml");
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
DocumentBuilder db = dbf.newDocumentBuilder();
Document document = db.parse(file);
document.getDocumentElement().normalize();
System.out.println("Root Element :" + document.getDocumentElement().getNodeName());
NodeList nList = document.getElementsByTagName("employee");
System.out.println("----------------------------");
for (int temp = 0; temp < nList.getLength(); temp++) {
Node nNode = nList.item(temp);
System.out.println("\nCurrent Element :" + nNode.getNodeName());
if (nNode.getNodeType() == Node.ELEMENT_NODE) {
Element eElement = (Element) nNode;
System.out.println("Employee id : " + eElement.getAttribute("id"));
System.out.println("First Name : "
+ eElement.getElementsByTagName("firstname").item(0).getTextContent());
System.out.println(
"Last Name : " + eElement.getElementsByTagName("lastname").item(0).getTextContent());
System.out.println(
"Salary : " + eElement.getElementsByTagName("salary").item(0).getTextContent());
}
}
} catch (IOException e) {
System.out.println(e);
}
}
}
Produzione:
Root Element :company
----------------------------
Current Element :employee
Employee id : 1001
First Name : Tony
Last Name : Black
Salary : 100000
Current Element :employee
Employee id : 2001
First Name : Amy
Last Name : Green
Salary : 200000
Leggi il file XML in Java POJO
Nel caso in cui desideriamo ottenere dati XML in un tipo compatibile con Java, possiamo utilizzare Java POJO per leggere i dati. Qui, usiamo il tipo Employee ArrayList
per aggiungere ogni nodo usando il metodo add()
e poi usiamo il cicli for
per iterare ogni oggetto. Vedi l’esempio sotto.
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.xml.sax.SAXException;
public class Main {
public static void main(String[] args)
throws ParserConfigurationException, SAXException, IOException {
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
Document document = builder.parse(new File("employee.xml"));
List<Employee> employees = new ArrayList<>();
NodeList nodeList = document.getDocumentElement().getChildNodes();
for (int i = 0; i < nodeList.getLength(); i++) {
Node node = nodeList.item(i);
if (node.getNodeType() == Node.ELEMENT_NODE) {
Element elem = (Element) node;
String firstname =
elem.getElementsByTagName("firstname").item(0).getChildNodes().item(0).getNodeValue();
String lastname =
elem.getElementsByTagName("lastname").item(0).getChildNodes().item(0).getNodeValue();
Double salary = Double.parseDouble(
elem.getElementsByTagName("salary").item(0).getChildNodes().item(0).getNodeValue());
employees.add(new Employee(firstname, lastname, salary));
}
}
for (Employee empl : employees) System.out.println(empl.toString());
}
}
class Employee {
private String Firstname;
private String Lastname;
private double salary;
public Employee(String Firstname, String Lastname, double salary) {
this.Firstname = Firstname;
this.Lastname = Lastname;
this.salary = salary;
}
@Override
public String toString() {
return "[" + Firstname + ", " + Lastname + ", " + salary + "]";
}
}
Produzione:
[Tony, Black, 100000.0]
[Amy, Green, 200000.0]
Leggere il file XML utilizzando jdom2
in Java
Jdom2 è una libreria che fornisce supporto per l’analisi DOM utilizzando classi Java. Abbiamo usato la classe SAXBuilder
e il metodo build()
per ottenere i dati in Document
e quindi utilizzando il metodo getRootElement()
, ottenere gli elementi. Vedi l’esempio sotto.
import java.io.File;
import java.io.IOException;
import java.util.List;
import javax.xml.parsers.ParserConfigurationException;
import org.jdom2.Document;
import org.jdom2.Element;
import org.jdom2.JDOMException;
import org.jdom2.input.SAXBuilder;
import org.xml.sax.SAXException;
public class Main {
public static void main(String[] args)
throws ParserConfigurationException, SAXException, IOException {
try {
File inputFile = new File("/employee.xml");
SAXBuilder saxBuilder = new SAXBuilder();
Document document = saxBuilder.build(inputFile);
System.out.println("Root element :" + document.getRootElement().getName());
Element classElement = document.getRootElement();
List<Element> studentList = classElement.getChildren();
System.out.println("----------------------------");
for (int temp = 0; temp < studentList.size(); temp++) {
Element student = studentList.get(temp);
System.out.println("\nCurrent Element :" + student.getName());
System.out.println("First Name : " + student.getChild("firstname").getText());
System.out.println("Last Name : " + student.getChild("lastname").getText());
System.out.println("Salary : " + student.getChild("salary").getText());
}
} catch (JDOMException e) {
e.printStackTrace();
} catch (IOException ioe) {
ioe.printStackTrace();
}
}
}
Produzione:
Root element :company
----------------------------
Current Element :employee
First Name : Tony
Last Name : Black
Salary : 100000
Current Element :employee
First Name : Amy
Last Name : Green
Salary : 200000
Leggere il file XML utilizzando XPath
in Java
Qui, usiamo la libreria XPath
per analizzare i file XML in Java. La classe XPathFactory
viene utilizzata per compilare tutti i nodi in NodeList
e quindi iterare ogni figlio attraverso il bucle for. Vedi l’esempio sotto.
import java.io.File;
import java.io.IOException;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import javax.xml.xpath.XPath;
import javax.xml.xpath.XPathConstants;
import javax.xml.xpath.XPathExpressionException;
import javax.xml.xpath.XPathFactory;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.xml.sax.SAXException;
public class Main {
public static void main(String[] args)
throws ParserConfigurationException, SAXException, IOException {
try {
File inputFile = new File("/employee.xml");
DocumentBuilderFactory dbFactory = DocumentBuilderFactory.newInstance();
DocumentBuilder dBuilder;
dBuilder = dbFactory.newDocumentBuilder();
Document doc = dBuilder.parse(inputFile);
doc.getDocumentElement().normalize();
XPath xPath = XPathFactory.newInstance().newXPath();
String expression = "/company/employee";
NodeList nodeList =
(NodeList) xPath.compile(expression).evaluate(doc, XPathConstants.NODESET);
for (int i = 0; i < nodeList.getLength(); i++) {
Node nNode = nodeList.item(i);
System.out.println("\nCurrent Element :" + nNode.getNodeName());
if (nNode.getNodeType() == Node.ELEMENT_NODE) {
Element eElement = (Element) nNode;
System.out.println("First Name : "
+ eElement.getElementsByTagName("firstname").item(0).getTextContent());
System.out.println(
"Last Name : " + eElement.getElementsByTagName("lastname").item(0).getTextContent());
System.out.println(
"Salary : " + eElement.getElementsByTagName("salary").item(0).getTextContent());
}
}
} catch (ParserConfigurationException e) {
System.out.println(e);
} catch (SAXException e) {
System.out.println(e);
} catch (IOException e) {
System.out.println(e);
} catch (XPathExpressionException e) {
System.out.println(e);
}
}
}
Produzione:
Current Element :employee
First Name : Tony
Last Name : Black
Salary : 100000
Current Element :employee
First Name : Amy
Last Name : Green
Salary : 200000
Leggere il file XML utilizzando DOM4J
in Java
Dom4j
è un’altra libreria in grado di analizzare i file XML in Java. Il metodo read()
della classe SAXReader viene utilizzato per leggere i nodi nel documento. Vedi l’esempio sotto.
import java.io.File;
import java.io.IOException;
import java.util.List;
import javax.xml.parsers.ParserConfigurationException;
import org.dom4j.Document;
import org.dom4j.DocumentException;
import org.dom4j.Element;
import org.dom4j.Node;
import org.dom4j.io.SAXReader;
import org.xml.sax.SAXException;
public class Main {
public static void main(String[] args)
throws ParserConfigurationException, SAXException, IOException {
try {
File inputFile = new File("employee.xml");
SAXReader reader = new SAXReader();
Document document = reader.read(inputFile);
System.out.println("Root element :" + document.getRootElement().getName());
Element classElement = document.getRootElement();
List<Node> nodes = document.selectNodes("company/employee");
System.out.println("----------------------------");
for (Node node : nodes) {
System.out.println("\nCurrent Element :" + node.getName());
System.out.println("First Name : " + node.selectSingleNode("firstname").getText());
System.out.println("Last Name : " + node.selectSingleNode("lastname").getText());
System.out.println("Salary : " + node.selectSingleNode("salary").getText());
}
} catch (DocumentException e) {
e.printStackTrace();
}
}
}
Produzione:
Root element :company
----------------------------
Current Element :employee
First Name : Tony
Last Name : Black
Salary : 100000
Current Element :employee
First Name : Amy
Last Name : Green
Salary : 200000