How to Convert Byte Array to Integer in Java
-
Convert Byte Array to Int in Java Using the
ByteBuffer
Class -
Convert Byte Array to Int in Java Using the
DataInputStream
Class - Convert Byte Array to Int in Java by Manually Shifting Bytes
-
Convert Byte Array to Int in Java Using the
BigInteger
Class - Convert Byte Array to Int in Java Using the Guava library
- Conclusion
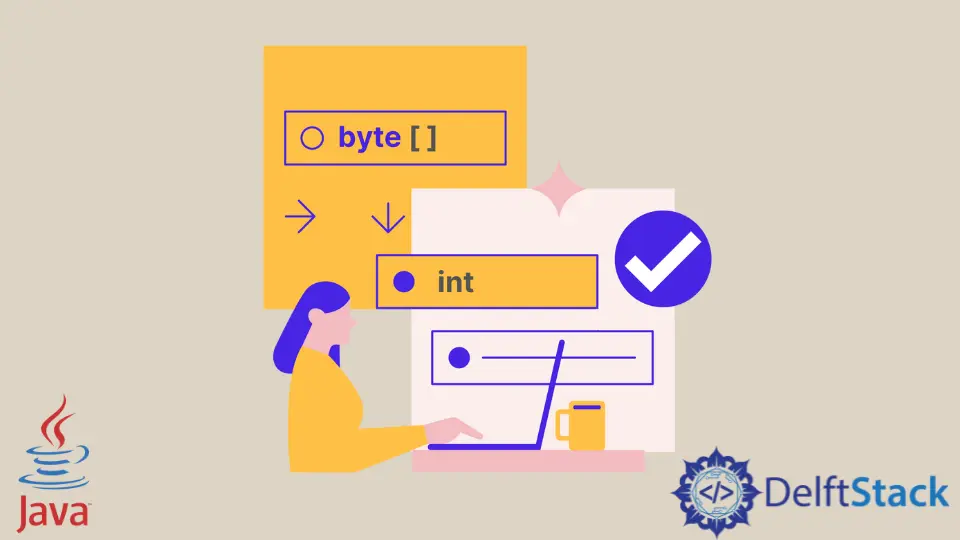
Converting a byte array to an integer in Java is a common task in programming, especially when dealing with network communication, file I/O, or other scenarios where data is represented in byte form.
Java provides several methods to achieve this conversion, allowing developers to efficiently handle the transformation of raw byte data into meaningful integer values. In this article, we’ll explore various approaches to convert a byte array to an int in Java.
Convert Byte Array to Int in Java Using the ByteBuffer
Class
One of the most straightforward and convenient ways to convert a byte array to an integer is by using the ByteBuffer
class. ByteBuffer
is part of the java.nio
package introduced in Java 1.4, which provides support for non-blocking I/O operations and working with buffers.
A ByteBuffer
is a container for handling sequences of bytes and provides methods for reading and writing various data types, including integers. To convert a byte array to an integer, we can use the wrap
method to create a ByteBuffer
instance and then call the getInt
method to interpret the bytes as an integer.
Let’s see how we can convert a byte array to an integer using ByteBuffer
:
import java.nio.ByteBuffer;
public class ByteArrayToInt {
public static void main(String[] args) {
byte[] byteArray = {0x00, 0x01, 0x03, 0x10};
int intValue = ByteBuffer.wrap(byteArray).getInt();
System.out.println("Converted Integer Value: " + intValue);
}
}
In this example, we declare a byte array named byteArray
containing four hexadecimal values. The ByteBuffer.wrap(byteArray)
method is employed to create a ByteBuffer
instance that wraps the provided byte array.
Subsequently, the getInt()
method is invoked on the ByteBuffer
instance, extracting and interpreting the next four bytes as an integer. The result is then printed to the console.
Output:
Converted Integer Value: 66320
As we can see, the output shows the integer value obtained by converting the byte array {0x00, 0x01, 0x03, 0x10}
using the ByteBuffer
class.
Note: The byte order (endianness) used by
ByteBuffer
depends on the system’s native byte order. If a specific byte order is required, you can use methods likeorder(ByteOrder)
on theByteBuffer
instance.
Let’s explore another example where we allocate memory explicitly and convert each byte separately.
import java.nio.ByteBuffer;
public class ByteArrayToIntSeparate {
public static void main(String[] args) {
byte[] byteArray = {0x03, 0x04};
ByteBuffer wrapped = ByteBuffer.wrap(byteArray);
short num = wrapped.getShort();
ByteBuffer resultBuffer = ByteBuffer.allocate(2);
resultBuffer.putShort(num);
for (byte convertedByte : resultBuffer.array()) {
System.out.println(convertedByte);
}
}
}
Here, we declare a byte array named byteArray
with two hexadecimal values. The ByteBuffer.wrap(byteArray)
method is used to wrap the byte array, and the getShort()
method reads the next two bytes as a short.
We then allocate memory for the result using ByteBuffer.allocate(2)
and put the short value into the new buffer using putShort()
. Finally, we iterate through the resulting byte array and print each converted byte.
Output:
3
4
This output signifies the conversion of each byte separately from the byte array {0x03, 0x04}
using the ByteBuffer
class. The values 3
and 4
represent the individual bytes converted to integers.
Convert Byte Array to Int in Java Using the DataInputStream
Class
While ByteBuffer
is a powerful tool for byte array manipulation, another method involves using the DataInputStream
class in Java. This class provides methods to read primitive data types from an input stream, and we can leverage it to convert a byte array to an integer.
The DataInputStream
class allows us to read primitive data types from an input stream. To convert a byte array to an integer, we can create a ByteArrayInputStream
with our byte array and then wrap it with a DataInputStream
.
The readInt()
method of DataInputStream
is then used to interpret the bytes as an integer.
import java.io.ByteArrayInputStream;
import java.io.DataInputStream;
public class ByteArrayToIntWithDataInputStream {
public static void main(String[] args) {
byte[] byteArray = {0x00, 0x01, 0x03, 0x10};
ByteArrayInputStream byteArrayInputStream = new ByteArrayInputStream(byteArray);
DataInputStream dataInputStream = new DataInputStream(byteArrayInputStream);
try {
int intValue = dataInputStream.readInt();
System.out.println("Converted Integer Value: " + intValue);
} catch (Exception e) {
e.printStackTrace();
}
}
}
In this example, we declare a byte array named byteArray
with the same hexadecimal values as the previous example. We then create a ByteArrayInputStream
and a DataInputStream
to handle the conversion.
Inside a try
block, we call readInt()
on the DataInputStream
, interpreting the next four bytes as an integer. Any exceptions during this process are caught and printed. The resulting integer value is then output to the console.
Output:
Converted Integer Value: 66320
This output represents the integer value obtained by converting the byte array {0x00, 0x01, 0x03, 0x10}
using the DataInputStream
class.
Now, let’s explore another example using DataInputStream
to convert each byte separately.
import java.io.ByteArrayInputStream;
import java.io.DataInputStream;
public class ByteArrayToIntSeparateWithDataInputStream {
public static void main(String[] args) {
byte[] byteArray = {0x03, 0x04};
ByteArrayInputStream byteArrayInputStream = new ByteArrayInputStream(byteArray);
DataInputStream dataInputStream = new DataInputStream(byteArrayInputStream);
try {
short num = dataInputStream.readShort();
System.out.println(num);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Here, we declare a byte array named byteArray
with two hexadecimal values. Similar to the previous example, we create a ByteArrayInputStream
and a DataInputStream
for the conversion.
Inside a try
block, we call readShort()
on the DataInputStream
, interpreting the next two bytes as a short. Any exceptions during this process are caught and printed.
The resulting short value is then output to the console.
Output:
772
This output signifies the conversion of each byte separately from the byte array {0x03, 0x04}
using the DataInputStream
class. The value 772
represents the short value obtained from interpreting the two bytes individually.
Convert Byte Array to Int in Java by Manually Shifting Bytes
An alternative approach to converting a byte array to an integer involves manually shifting the bytes. This method requires careful bit manipulation to reconstruct the integer from individual bytes.
While it may be less concise than using ByteBuffer
or DataInputStream
, it provides a clear understanding of the bit-level operations involved. The process of manually shifting bytes to convert a byte array to an integer involves:
- Iterating through the byte array.
- Shifting each byte to its appropriate position.
- Combining them to form the final integer value.
Let’s see an implementation of manually shifting bytes in Java.
public class ByteArrayToIntManually {
public static void main(String[] args) {
byte[] byteArray = {0x00, 0x01, 0x03, 0x10};
int intValue = 0;
for (int i = 0; i < 4; i++) {
intValue = (intValue << 8) | (byteArray[i] & 0xFF);
}
System.out.println("Converted Integer Value: " + intValue);
}
}
In this example, we begin by declaring a byte array named byteArray
with some hexadecimal values. The variable intValue
is initialized to 0, and a loop iterates through the byte array.
Inside the loop, we shift the existing bits of intValue
to the left by 8 positions and use the bitwise OR (|
) operator to combine the next byte from the array, ensuring that only the lower 8 bits of the byte are considered (byteArray[i] & 0xFF
). This process is repeated for each byte in the array, resulting in the reconstruction of the integer value.
Output:
Converted Integer Value: 66320
This output displays the integer value obtained by manually shifting bytes from the byte array {0x00, 0x01, 0x03, 0x10}
. The manual bit manipulation within the loop effectively combines the bytes to form the final integer result.
While this method may be more explicit, it offers insights into the binary operations involved in the conversion process.
Convert Byte Array to Int in Java Using the BigInteger
Class
Yet another method for converting a byte array to an integer involves the use of the BigInteger
class, typically known for handling arbitrary-precision integers. In this context, BigInteger
provides a convenient mechanism for interpreting byte arrays as integers.
The BigInteger
class allows for the representation and manipulation of integers of arbitrary precision. To convert a byte array to an integer, we instantiate a BigInteger
object by passing the byte array to its constructor.
Once created, the intValue()
method facilitates the extraction of the integer value.
Let’s see a code example that demonstrates the usage of BigInteger
for byte array to int conversion:
import java.math.BigInteger;
public class ByteArrayToIntWithBigInteger {
public static void main(String[] args) {
byte[] byteArray = {0x00, 0x01, 0x03, 0x10};
BigInteger bigIntValue = new BigInteger(byteArray);
int intValue = bigIntValue.intValue();
System.out.println("Converted Integer Value: " + intValue);
}
}
In this example, a BigInteger
object, bigIntValue
, is created by passing the byte array to its constructor. The intValue()
method is then applied to obtain the integer representation.
The output of the code is as follows:
Converted Integer Value: 66320
This output signifies the integer value obtained by converting the byte array {0x00, 0x01, 0x03, 0x10}
using the BigInteger
class. The simplicity and ease of use of BigInteger
make it a viable option for byte array to int conversions, especially in scenarios involving arbitrary-precision integers.
Convert Byte Array to Int in Java Using the Guava library
In addition to the core Java libraries, the Guava library provides utilities that simplify converting a byte array to an integer. Guava offers a convenient method through its UnsignedBytes
class, which provides static methods for dealing with bytes in an unsigned manner.
Guava’s UnsignedBytes
class includes a method called toInt()
, which takes a byte array as a parameter and converts it into an integer. This method considers the bytes in the array as unsigned values, providing a seamless way to handle byte array to integer conversions without the need for manual bit manipulation.
import com.google.common.primitives.UnsignedBytes;
public class ByteArrayToIntWithGuava {
public static void main(String[] args) {
byte[] byteArray = {0x00, 0x01, 0x03, 0x10};
int intValue = 0;
for (byte b : byteArray) {
intValue = (intValue << 8) | (UnsignedBytes.toInt(b) & 0xFF);
}
System.out.println("Converted Integer Value: " + intValue);
}
}
In this example, we utilize the Guava library to convert a byte array to an integer. The UnsignedBytes.toInt()
method is employed, taking the byteArray
as an argument.
The UnsignedBytes.toInt()
method treats each byte in the array as an unsigned value and performs the conversion. The resulting integer value is then printed to the console.
Output:
Converted Integer Value: 66320
The output represents the integer value obtained by converting the byte array {0x00, 0x01, 0x03, 0x10}
using Guava’s UnsignedBytes
class.
Guava’s UnsignedBytes
class simplifies the byte-to-integer conversion process, offering an elegant and concise solution for handling unsigned byte values. This method is particularly beneficial when working with applications that involve unsigned byte representations, streamlining the code, and making it more readable.
Conclusion
Converting a byte array to an integer in Java can be approached through various methods, each with its advantages and use cases. Whether using core Java functionalities like ByteBuffer
and DataInputStream
, employing manual bit manipulation, or leveraging external libraries like Guava, you have a range of tools at your disposal.
Choosing the appropriate method depends on factors such as performance requirements, code readability, and the specific nature of the application. By understanding these different approaches, you can make informed decisions based on your project’s needs.