Java 中将字节数组转换为整数
Siddharth Swami
2023年10月12日
Java
Java Array
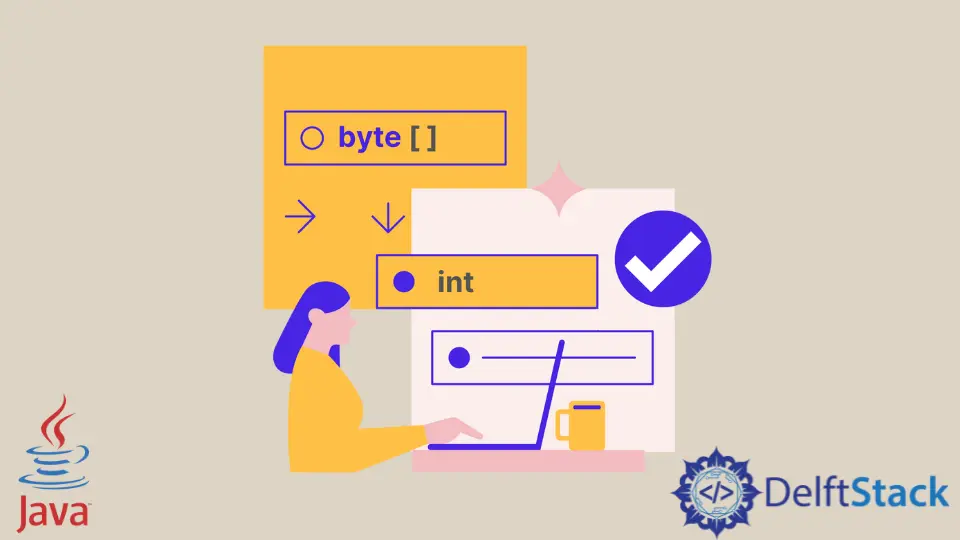
字节属于 Java 中的原始数据类型。Java 中的字节数组是包含字节的数组,并存储二进制数据的集合。整数数据类型表示 Java 中的整数。字节和整数紧密相连,因为二进制数据可以转换为整数形式。
在本文中,我们将在 Java 中将字节数组转换为整数类型。
Java 中 java.nio
包中的 ByteBuffer
类为我们提供了一种将字节数组转换为整数的方法。
请参考下面的代码。
import java.nio.ByteBuffer;
public class Test {
public static void main(String[] args) {
byte[] bytes = {0x00, 0x01, 0x03, 0x10};
System.out.println(ByteBuffer.wrap(bytes).getInt() + " ");
}
}
输出:
66320
在上面的代码中,我们创建了一个给定长度的字节数组的 Bytebuffer
,然后,我们从中读取接下来的四个字节作为整数类型。ByteBuffer
方法将字节数组包装到一个缓冲区中,getInt()
函数将类中接下来的四个字节作为整数读取。
下面我们有另一个 ByteBuffer
类的例子。在下面的代码中,我们只分配了两个内存。这些方法执行的功能与前一个相同。这里它分别转换每个字节,因为我们可以看到它给出了两个不同的输出。
请参考下面的代码。
import java.nio.*;
class Byte_Array_To_Int {
static void byte_To_Int(byte ar[]) {
ByteBuffer wrapped = ByteBuffer.wrap(ar); // big-endian by default
short num = wrapped.getShort();
ByteBuffer dbuf = ByteBuffer.allocate(2); // allocates the memory by 2.
dbuf.putShort(num);
byte[] bytes = dbuf.array();
for (int i = 0; i < bytes.length; i++) { // loop for printing the elements.
System.out.println(bytes[i]);
}
}
public static void main(String[] args) {
byte[] b = {0x03, 0x04}; // byte type array.
byte_To_Int(b);
}
输出:
3
4
ByteBuffer.wrap()
将数组包装到缓冲区中。默认情况下,它以 big-endian 0x00 类型的形式存储数据。请注意,在输出中,我们有两个不同的整数。如前所述,此代码获取数组中存在的每个元素并相应地对其进行转换。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe