How to Convert an ASCII Code to Char
- Use Casting to Convert ASCII to Char in Java
-
Use
Character.toString
to Convert ASCII to Char in Java -
Use
Character.toString
to Convert ASCII to Char in Java -
Use
Character.toChars()
to Convert ASCII to Char in Java
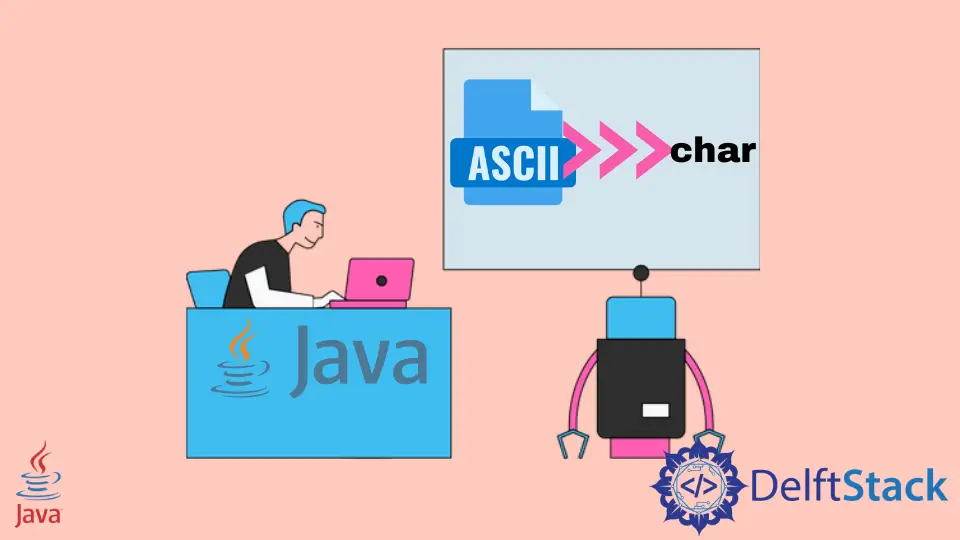
This article discusses how you can convert an ASCII code into its character using methods in Java. Additionally, we demonstrate how you can change upper-case alphabets into lower-case, and vice versa.
Use Casting to Convert ASCII to Char in Java
The most basic and easy way to extract the character from an ASCII Code is to cast the ASCII code to a char
directly; this will convert the asciiValue
of the int
type to a char
type.
public class Main {
public static void main(String[] args) {
int asciiValue = 97;
char convertedChar = (char) asciiValue;
System.out.println(convertedChar);
}
}
Output:
a
Use Character.toString
to Convert ASCII to Char in Java
The character class of Java provides us with a toString()
method, which is converted to a char
in a codePoint; in this case, we have an ASCII code. We can put the conversion method into a loop to get all the uppercase English alphabets. Note that the loop goes from 65 to 90, which are the codes corresponding to the uppercase alphabets.
This method’s benefit from the example we used above is that it can throw an exception if the int
value is not validated correctly.
public class Main {
public static void main(String[] args) {
int asciiValue = 65;
for (int i = asciiValue; i <= 90; i++) {
String convertedChar = Character.toString(i);
System.out.println(i + " => " + convertedChar);
}
}
}
Output:
65 => A
66 => B
67 => C
68 => D
69 => E
70 => F
71 => G
72 => H
73 => I
74 => J
75 => K
76 => L
77 => M
78 => N
79 => O
80 => P
81 => Q
82 => R
83 => S
84 => T
85 => U
86 => V
87 => W
88 => X
89 => Y
90 => Z
Use Character.toString
to Convert ASCII to Char in Java
To convert the ASCII codes to lower-case alphabets, we only need to change the loop range; it should start with 97 and end at 122.
public class Main {
public static void main(String[] args) {
int asciiValue = 97;
for (int i = asciiValue; i <= 122; i++) {
String convertedChar = Character.toString((char) i);
System.out.println(i + " => " + convertedChar);
}
}
}
Output:
97 => a
98 => b
99 => c
100 => d
101 => e
102 => f
103 => g
104 => h
105 => i
106 => j
107 => k
108 => l
109 => m
110 => n
111 => o
112 => p
113 => q
114 => r
115 => s
116 => t
117 => u
118 => v
119 => w
120 => x
121 => y
122 => z
Use Character.toChars()
to Convert ASCII to Char in Java
We can use another method of the character class in Java, which is toChars
; it takes a codePoint like the ASCII value and returns an array of char
.
public class Main {
public static void main(String[] args) {
int asciiValue = 255;
char[] convertedCharArray = Character.toChars(asciiValue);
System.out.println(convertedCharArray);
}
}
Output:
ÿ
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn