How to Convert Int to ASCII in Java
-
Convert Int to ASCII in Java Using Type Casting
(char)
-
Convert Int to ASCII in Java Using
Character.toString()
-
Convert Int to ASCII in Java Using
Character.forDigit()
-
Convert Int to ASCII in Java Using
String.valueOf()
- Conclusion
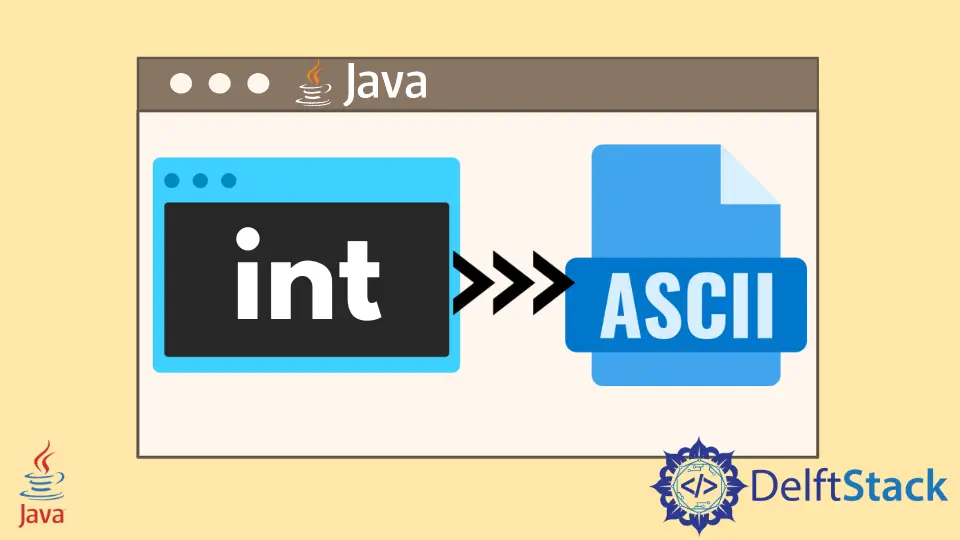
This tutorial delves into the process of converting an integer to its corresponding ASCII code in Java, providing a comprehensive understanding of the intricacies involved in this fundamental programming task.
In Java, the int
data type serves as a fundamental primitive type for storing numeric values, either signed or unsigned.
On the other hand, ASCII (American Standard Code for Information Interchange) is a widely-used character encoding standard that computers internally utilize. It assigns a unique numerical value to each keyboard key, allowing computers to process and display textual data.
Understanding how to access and display the ASCII code of an integer value in Java is crucial for various programming tasks. This tutorial presents multiple approaches involved in obtaining ASCII representations from integer values in Java.
Convert Int to ASCII in Java Using Type Casting (char)
The simplest approach to convert an integer to its ASCII code is through type casting to the char
data type. The char
data type is a 16-bit unsigned integer that represents a Unicode character.
Since ASCII is a subset of Unicode, each ASCII value corresponds to a valid char
in Java.
Below are the steps to convert an integer to its ASCII representation using type casting in Java:
-
Begin by obtaining the integer value that you want to convert to ASCII.
-
Use type casting to convert the integer to its ASCII character representation using
(char)
. -
Store the resulting ASCII character in a
char
variable.
Let’s illustrate this process with an example:
public class IntegerToAsciiExample {
public static void main(String[] args) {
int integerValue = 65;
char asciiChar = (char) integerValue;
System.out.println("ASCII Character: " + asciiChar);
}
}
Output:
ASCII Character: A
In this example, an integer value of 65
is chosen as an example. This integer value represents the ASCII code for a specific character.
To convert it to its corresponding ASCII character, the int
variable integerValue
is typecast to a char
, resulting in the ASCII character A
because the ASCII value for A
is 65
.
The resulting ASCII character is then stored in the char
variable asciiChar
. Finally, the program prints the ASCII character to the console using System.out.println
.
You can easily customize the integer value to convert any desired integer to its ASCII character. Simply replace the integerValue
in the example with the integer you want to convert.
Here’s a simple example demonstrating how to convert an integer to its ASCII representation:
public class SimpleTesting {
public static void main(String[] args) {
int integerValue = 97;
System.out.println("Integer Value: " + integerValue);
char asciiChar = (char) integerValue;
System.out.println("ASCII Character: " + asciiChar);
}
}
Output:
Integer Value: 97
ASCII Character: a
Here, we used an integer value of 97
and demonstrated how type casting to char
converted it into the corresponding ASCII character, which is a
.
Convert Int to ASCII in Java Using Character.toString()
Another effective way to convert an integer to its corresponding ASCII code is by utilizing the Character.toString()
method. This method in Java is primarily used to convert a char
type to a String
.
However, since char
represents characters using their ASCII values, we can use this method to convert an integer to its corresponding ASCII character and then convert it to a String
. This is especially useful when you want the result in string format.
The syntax of the Character.toString()
method in Java is:
public static String toString(char c)
The Character.toString()
method takes a char
as a parameter and returns a String
representation of that char
.
Here are the steps to convert an integer to its ASCII representation using Character.toString()
:
-
Begin by obtaining the integer value that you want to convert to ASCII.
-
Convert the integer to its corresponding ASCII character using
(char)
type casting. -
Use
Character.toString()
to convert the character to a string.
Let’s illustrate this process with an example:
public class IntegerToAsciiExample {
public static void main(String[] args) {
int integerValue = 65;
char asciiChar = (char) integerValue;
String asciiString = Character.toString(asciiChar);
System.out.println("ASCII Character: " + asciiString);
}
}
Output:
ASCII Character: A
As we can see, within the main
method of the IntegerToAsciiExample
class, an integer value of 65
is used as an example. Firstly, the integer is typecast to a char
using (char) integerValue
, converting it to the corresponding ASCII character.
This ASCII character is then converted to a string using Character.toString()
. Finally, the program prints the ASCII character in string format to the console using System.out.println
.
In this case, the output is "ASCII Character: A"
as the ASCII code 65
corresponds to the character A
.
Here’s another example:
public class SimpleTesting {
public static void main(String[] args) {
int integerValue = 97;
System.out.println("Integer Value: " + integerValue);
String asciiString = Character.toString((char) integerValue);
System.out.println("ASCII Character: " + asciiString);
}
}
Output:
Integer Value: 97
ASCII Character: a
In this example, we use the Character.toString()
method to directly convert the integer to its ASCII representation in string format. The output showcases the initial integer value and the corresponding ASCII character, demonstrating the effectiveness of this approach.
Convert Int to ASCII in Java Using Character.forDigit()
Converting an integer to its corresponding ASCII code can also be done using the forDigit()
method of the Character
class. The Character.forDigit()
method in Java is designed to convert a numerical digit to its corresponding character representation.
This method allows for the conversion of a digit (0-9) to its corresponding ASCII character. By adjusting the parameters accordingly, we can also convert integers to their ASCII representation.
Here’s how we use Character.forDigit()
for conversion:
public static char forDigit(int digit, int radix)
The Character.forDigit()
method converts the specified digit into its ASCII representation based on the given radix (typically 10 for decimal representation). The digit
parameter represents the digit to be converted, and the radix
parameter defines the base for representing the digit.
Here are the steps to convert an integer to its ASCII representation using Character.forDigit()
:
-
Begin by obtaining the integer value that you want to convert to ASCII.
-
Use the
Character.forDigit()
method to convert the integer to its corresponding ASCII character.
Let’s illustrate this process with an example:
public class IntegerToAsciiExample {
public static void main(String[] args) {
int integerValue = 10;
char asciiChar = Character.forDigit(integerValue, 16);
System.out.println("ASCII Character: " + asciiChar);
}
}
Output:
ASCII Character: a
In the main
method, an integer variable integerValue
is initialized with the value 10
.
Next, the Character.forDigit()
method is called with integerValue
and the radix 16
. This method returns the ASCII character representation of the input integer using the specified radix.
In this case, the radix 16
corresponds to hexadecimal representation.
The resulting ASCII character is stored in the asciiChar
variable. Finally, the program prints out the ASCII character for the integer value 10
in hexadecimal.
Here’s another example demonstrating how to use the forDigit()
method to obtain ASCII values for different integers:
public class SimpleTesting {
public static void main(String[] args) {
int integerValue = 97; // Example integer value
System.out.println("Integer Value: " + integerValue);
// Get ASCII value for 5 in decimal (base 10)
char ch1 = Character.forDigit(5, 10);
System.out.println("ASCII Value (5 in decimal): " + ch1);
// Get ASCII value for 15 in hexadecimal (base 16)
char ch2 = Character.forDigit(15, 16);
System.out.println("ASCII Value (15 in hexadecimal): " + ch2);
}
}
Output:
Integer Value: 97
ASCII Value (5 in decimal): 5
ASCII Value (15 in hexadecimal): f
In this example, we use the Character.forDigit()
method to obtain ASCII values for different integers in various bases (decimal and hexadecimal). The output showcases the initial integer value and its corresponding ASCII values based on the specified radix.
Convert Int to ASCII in Java Using String.valueOf()
The String.valueOf()
method in Java can be used to convert various data types to strings, including integers. Although its primary purpose is to convert numerical and non-numerical data to string representations, we can utilize it to obtain the ASCII representation of an integer.
In our case, we use it to convert a char
(obtained through type casting) representing the ASCII character back to a string.
Here’s how we use String.valueOf()
for conversion:
String asciiString = String.valueOf((char) integerValue);
In this line, integerValue
is the integer we want to convert to ASCII, which is first typecasted to a char
. Then, we use String.valueOf()
to convert this char
to its string representation.
Here are the steps to convert an integer to its ASCII representation using String.valueOf()
:
-
Start by obtaining the integer value that you want to convert to ASCII.
-
Utilize the
String.valueOf()
method to convert the integer to its string representation.
Let’s illustrate this process with an example:
public class IntegerToAsciiExample {
public static void main(String[] args) {
int integerValue = 65;
String asciiString = String.valueOf((char) integerValue);
System.out.println("ASCII Character: " + asciiString); //
}
}
Output:
ASCII Character: A
Initially, an integer variable, integerValue
, is assigned the value 65
, representing the ASCII code for the character A
. The String.valueOf()
method is then employed, wherein the integer value is first typecasted to a char
to align with the ASCII representation.
Subsequently, the String.valueOf()
method is used to convert the char
to its string representation, effectively obtaining the ASCII character. Finally, the resultant ASCII character is printed to the console using System.out.println()
.
Below is another example demonstrating how to use the String.valueOf()
method to obtain ASCII values for different integers:
public class SimpleTesting {
public static void main(String[] args) {
int integerValue = 97;
System.out.println("Integer Value: " + integerValue);
String asciiString = String.valueOf((char) integerValue);
System.out.println("ASCII Character: " + asciiString);
}
}
Output:
Integer Value: 97
ASCII Character: a
In this example, we use String.valueOf()
to obtain the ASCII value corresponding to the integer 97
. The output showcases the initial integer value and its corresponding ASCII character.
Conclusion
We have discussed the different methods to convert an integer to its ASCII representation in Java.
The most straightforward method involves type casting the integer to the char
data type, given that char
is a 16-bit unsigned integer that directly corresponds to Unicode characters, including the ASCII subset. This method provides a simple and efficient way to convert integers to their respective ASCII characters.
Another effective approach is utilizing the Character.toString()
method, originally designed to convert a char
to a String
. By leveraging this method and typecasting the integer to a char
first, we can conveniently convert integers to ASCII characters and obtain the result in string format.
Furthermore, the Character.forDigit()
method offers an alternative means of converting an integer to its ASCII representation by allowing conversion from numerical digits to their corresponding characters based on a specified radix. This method proves useful when dealing with various bases and provides flexibility in obtaining ASCII characters for different integers.
Lastly, the String.valueOf()
method can also be employed to obtain the ASCII representation of an integer. By typecasting the integer to a char
and utilizing String.valueOf()
, we can efficiently convert integers to their corresponding ASCII characters and represent the result as a string.
Related Article - Java Integer
- How to Handle Integer Overflow and Underflow in Java
- How to Reverse an Integer in Java
- How to Convert Int to Binary in Java
- The Max Value of an Integer in Java
- Minimum and Maximum Value of Integer in Java
- How to Convert Integer to Int in Java