How to Convert Character to ASCII Numeric Value in Java
-
Cast
char
toint
to Convert a Character Into ASCII Value in Java -
getBytes(StandardCharsets.US_ASCII)
to Get the ASCII Value From a Character in Java -
String.chars()
to Convert Character to ASCII Values in Java 9+
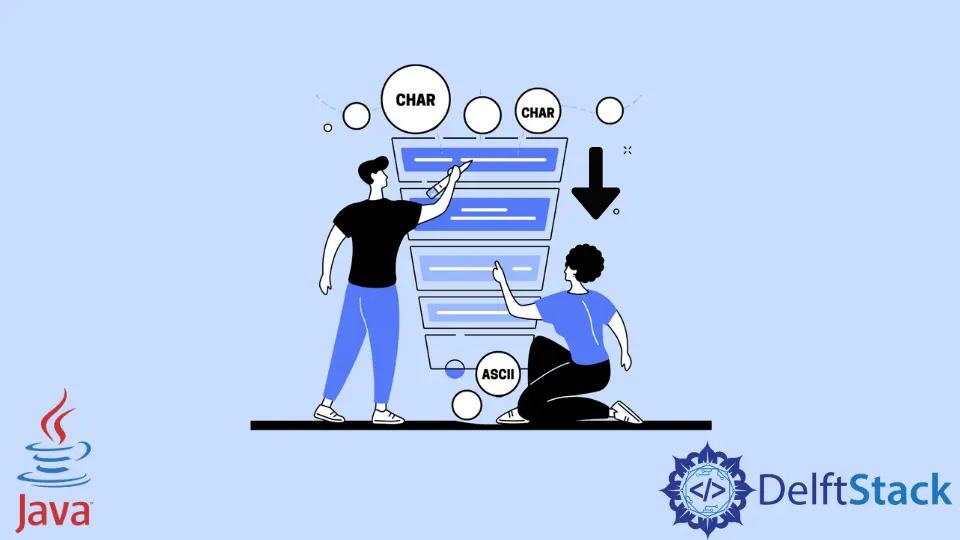
This article will discover the easiest and effective methods to convert a given character into an ASCII numeric value in Java with examples.
Cast char
to int
to Convert a Character Into ASCII Value in Java
One of the easiest ways to convert the character to an ASCII numeric value is to cast the character to int. As we are talking about a single character, we cannot use multiple characters.
Check out the example below. Notice we are using the character a
enclosed within single quotes, making its data type as char
.
public class Main {
public static void main(String[] args) {
int asciiValue = 'a';
System.out.println("ASCII Numeric Value: " + asciiValue);
}
}
Output:
ASCII Numeric Value: 97
getBytes(StandardCharsets.US_ASCII)
to Get the ASCII Value From a Character in Java
For this example to work, we will first get our character as a string and then convert that string to an array of bytes. StandardCharsets.US_ASCII
will ensure that we will get the right output value.
import java.nio.charset.StandardCharsets;
public class Main {
public static void main(String[] args) {
String s = "b";
byte[] bytes = s.getBytes(StandardCharsets.US_ASCII);
System.out.println("ASCII Numeric Value: " + bytes[0]);
}
}
Output:
ASCII Numeric Value: 98
This approach’s benefit is that we can directly get the ASCII value of multiple characters as the characters are in a string.
We have a string s
having 4 characters. We can get ASCII numeric values for all of these characters as we have done below.
import java.nio.charset.StandardCharsets;
public class Main {
public static void main(String[] args) {
String s = "ball";
byte[] bytes = s.getBytes(StandardCharsets.US_ASCII);
System.out.println("ASCII Numeric Value of b: " + bytes[0]);
System.out.println("ASCII Numeric Value of a: " + bytes[1]);
System.out.println("ASCII Numeric Value of l: " + bytes[2]);
System.out.println("ASCII Numeric Value of l: " + bytes[3]);
}
}
Output:
ASCII Numeric Value: 98
ASCII Numeric Value: 97
ASCII Numeric Value: 108
ASCII Numeric Value: 108
String.chars()
to Convert Character to ASCII Values in Java 9+
In this method, we will use an API added in Java 9. We can get an IntStream
using String.chars()
and then a Stream of Integer
objects using .boxed()
. It will numerically give us the ASCII values that we can then put into a List<Integer>
to loop through.
This example takes the string building
as input and prints the ASCII values of every character in the string.
import java.util.List;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args) {
String stringValue = "building";
List<Integer> listOfIntegers = stringValue.chars().boxed().collect(Collectors.toList());
for (int i : listOfIntegers) {
System.out.println("ASCII value " + i);
}
}
}
Output:
ASCII value 98
ASCII value 117
ASCII value 105
ASCII value 108
ASCII value 100
ASCII value 105
ASCII value 110
ASCII value 103
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn