How to Increase an Array Size in Java
- Increase an Array Size by Creating Another New Array in Java
-
Increase the Array Size Using the
Arrays.copyOf()
Method in Java -
Increase the Array Size Using the
ArrayList
Array in Java - Increase Array Size in Java
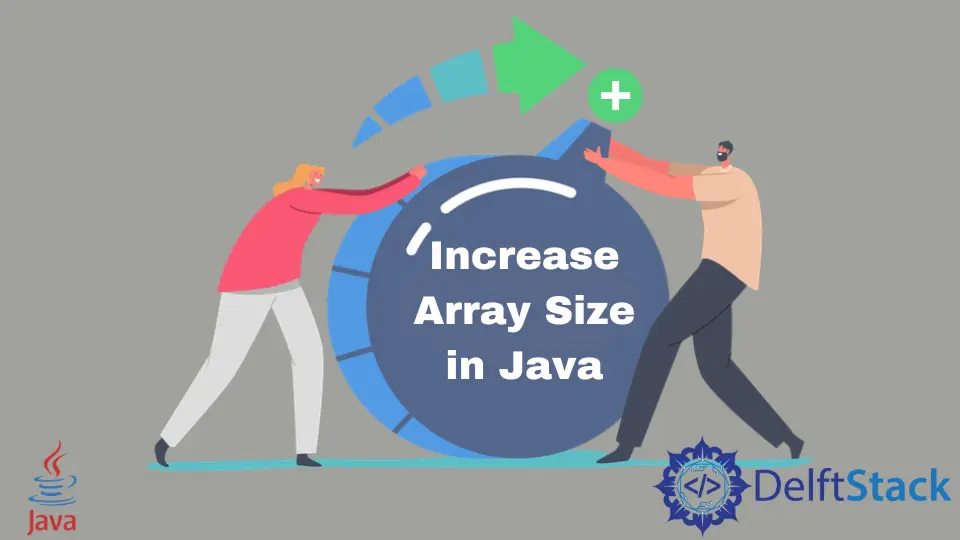
This tutorial introduces how to increase an array size in Java. You also have some example codes to help you understand the topic.
An array in Java is a finite collection of data of the same type. Arrays are of fixed size, and their length cannot be modified after their creation. It is because arrays are stored in memory as contiguous blocks of data
.
The user must predefine their size to ensure that the amount of memory required is available contiguously. In this guide, you will know the different ways to tackle the fixed-length issue of arrays.
Let’s first see what happens when we try to add elements to an array with no extra space. We usually add items to an array by accessing its index. If we try to add an item to an already-full array using the next index, Java will return an ArrayIndexOutOfBoundsException
.
The following code is an example of such a scenario.
public class Main {
public static void main(String[] args) {
int[] arr = new int[3];
arr[0] = 5;
arr[1] = 10;
arr[2] = 15;
arr[3] = 20; // Index 3 is out of bounds
}
}
Output:
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: Index 3 out of bounds for length 3
at abc.Demo.main(Demo.java:13)
Now let’s try to understand how to overcome this problem.
Increase an Array Size by Creating Another New Array in Java
A simple solution to the fixed size of the array is to create another array with a larger size.
We can use a counter variable to keep track of the number of elements inserted into the array. Whenever this number becomes equal to the array’s length (indicating that the array is full), we can create a new array that is one size larger than the original array.
Next, we will transfer all the elements from the original array to the new array and still have one extra space for a new element. We can repeat this process if we receive a new element and don’t have enough space for it.
Consider the following code where we create an array of length 5
and try to insert 7
elements in it. Whenever the array becomes full, we increase its size by using our increaseSize()
method.
public class Main {
public static int[] increaseSize(int[] arr) {
int[] newArr = new int[arr.length + 1]; // Creating a new array with space for an extra element
for (int i = 0; i < arr.length; i++) {
newArr[i] = arr[i]; // Copying the elements to the new array
}
return newArr;
}
public static void main(String[] args) {
int[] arr = new int[5];
int counter = 0;
for (int i = 0; i <= 6; i++) {
if (counter == arr.length) {
arr = increaseSize(arr);
}
arr[i] = i * 2;
counter += 1;
}
for (int i = 0; i < arr.length; i++) {
System.out.print(arr[i] + " ");
}
}
}
Output:
0 2 4 6 8 10 12
Increase the Array Size Using the Arrays.copyOf()
Method in Java
Java has a built-in copyOf()
method that can create a new array of a larger size and copy our old array elements into the new one.
The copyOf()
function belongs to the Arrays
class. The syntax of this method is shown below. It returns an array of the mentioned length that has all the elements of the original array.
copyOf(originalArray, newLength)
The following code is similar to the one mentioned above. The only difference is that we are using the copyOf
method instead of the increaseSize()
method.
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
int[] arr = new int[5];
int counter = 0;
for (int i = 0; i <= 6; i++) {
if (counter == arr.length) {
int[] newArr = Arrays.copyOf(arr, arr.length + 1);
arr = newArr;
}
arr[i] = i * 2;
counter += 1;
}
for (int i = 0; i < arr.length; i++) {
System.out.print(arr[i] + " ");
}
}
}
Output:
0 2 4 6 8 10 12
Increase the Array Size Using the ArrayList
Array in Java
An ArrayList
is a dynamic array found in the java.util
package and implements the List
interface. Unlike a normal array, an ArrayList
has a variable length.
Java automatically handles the size of this dynamic array, and we don’t need to worry about running out of space in the array. Its default size is 10
elements, but it will grow in size if more elements are added.
The following table below explains some of the key differences between an array and an ArrayList
.
Array | ArrayList |
---|---|
Arrays are not resizable and will always have a fixed length after creation. | ArrayList is a variable size array, and its size will increase dynamically if additional space is required. |
Arrays can contain both primitive data types and objects. | ArrayList can only store objects and not primitive data types. Primitive data types are converted into the corresponding objects before adding them to the list. (int to Integer, double to Double) |
Performance of arrays is better than ArrayList as the elements can be added, removed, or fetched using indices in constant time. |
ArrayLists are slightly slower than arrays as they need to be resized if we need additional space. |
An element can be accessed by using its index. | There are dedicated methods like get() , set() , or add() to access and modify elements in the ArrayList . We cannot directly use indices in square brackets to access elements. |
The following code explains how to use ArrayLists
and perform basic operations like adding an element, modifying an element, and printing the list values.
import java.util.ArrayList;
public class Main {
public static void main(String[] args) { // Creating a new ArrayList to hold Integer values
ArrayList<Integer> arrList = new ArrayList<Integer>();
// Adding elements 5, 10, 15 and 20 to the ArrayList
arrList.add(5);
arrList.add(10);
arrList.add(15);
arrList.add(20);
// Printing the ArrayList
System.out.println(arrList);
// Adding an element 7 at index 2. This will shift the rest of the elements one place to the
// right
arrList.add(2, 7);
System.out.println(arrList);
// Fetching the element at index 3
System.out.println(arrList.get(3));
// Changing the element present at index 1 to 17
arrList.set(1, 17);
System.out.println(arrList);
}
}
Output:
[5, 10, 15, 20]
[5, 10, 7, 15, 20]
15
[5, 17, 7, 15, 20]
The scenario where we were trying to add 7
elements to an array of length 5
can easily be solved by using ArrayLists
. Remember that we cannot use primitive data types like int
in ArrayLists
, so we use the wrapper class, Integer
.
import java.util.ArrayList;
public class Main {
public static void main(String[] args) {
ArrayList<Integer> arrList = new ArrayList<Integer>();
for (int i = 0; i <= 6; i++) {
arrList.add(i * 2);
}
for (int i = 0; i < arrList.size(); i++) {
System.out.print(arrList.get(i) + " ");
}
}
}
Output:
0 2 4 6 8 10 12
Increase Array Size in Java
An array is a basic yet extremely important and useful data structure. A limitation of arrays is that they are of fixed size, and their size cannot increase dynamically.
In this tutorial, we learned how to increase the size of an array. We can create a new array of a larger size and copy the contents of the old array to the new one. The best and simplest solution is by using ArrayLists
as they can dynamically increase their size.
We hope this guide was very helpful and you learned something new in Java.
Related Article - Java Variable
- Java Synchronised Variable
- Difference Between Static and Final Variables in Java
- How to Set JAVA_HOME Variable in Java
- How to Create Counters in Java
- How to Access a Variable From Another Class in Java