How to Access a Variable From Another Class in Java
-
Accessing Variables in Another Class Using
public
Access Modifier -
Accessing Variables in Another Class Using
getter
andsetter
Methods -
Accessing Variables in Another Class Using
protected
Access Modifier -
Accessing Variables in Another Class Using
Package-Private
Access Modifier -
Accessing Variables in Another Class Using the
Instance
Methods - Conclusion
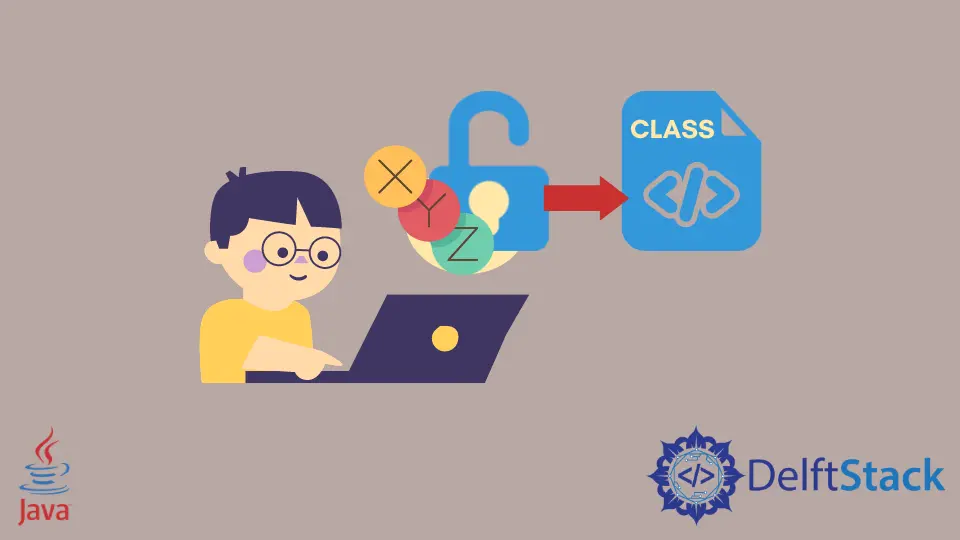
This tutorial introduces how to call a variable from another class in Java. We’ve included some example programs you can follow to execute this project.
A variable
is defined as the name used for holding a value of any type during program execution.
In Java, a variable
can be static
, local
, or instance
.
If a variable is static
, we can access it by using the class name. If a variable is an instance
, we must use a class object to access the variable.
Accessing variables in another class is essential for building modular and organized Java programs.
In object-oriented programming, classes serve as blueprints for objects, and each class encapsulates its own data and behavior. However, real-world applications often require collaboration among different classes to achieve complex functionalities.
By accessing variables in another class, we enable the exchange of information and promote code reusability. This allows developers to compartmentalize code logic, making it more manageable and easier to maintain.
Moreover, it facilitates the creation of relationships between classes, fostering a modular architecture that aligns with the principles of encapsulation and abstraction.
Overall, accessing variables in another class enhances the flexibility and scalability of a Java program, promoting a more efficient and collaborative development process.
Accessing Variables in Another Class Using public
Access Modifier
The public
access modifier makes variables accessible from outside the class, allowing other classes to read or modify them. This promotes code flexibility, as it enables collaboration between different components of a program.
Unlike other access modifiers, such as private
or protected
, public
variables are visible and usable by any class, simplifying communication and promoting code reusability. It establishes a well-defined interface for interactions between classes, making the code more transparent and easier to understand.
Using the public
access modifier is a deliberate and explicit choice, signaling that certain variables are intentionally made accessible to the broader scope of the program for specific purposes.
However, it is essential to use this approach judiciously, as it breaks encapsulation and might lead to unintended consequences.
Let’s illustrate this concept with a practical example:
// Class Student
public class Student {
public String name; // Public variable
public int age; // Public variable
}
In the above code, the Student
class has two public
variables: name
and age
.
public
variables, such as name
and age
, are declared with the public
access modifier, indicating that they can be accessed directly from any class.
To illustrate this, let’s consider the creation of another class, named Main
, where these public
variables are accessed directly.
This approach simplifies the interaction between classes, allowing seamless access to specific variables without the need for additional methods or complex mechanisms.
// Class Main
public class Main {
public static void main(String[] args) {
// Creating an instance of the Student class
Student student = new Student();
// Accessing public variables directly
student.name = "John Doe";
student.age = 21;
// Displaying the values of public variables
System.out.println("Student Name: " + student.name);
System.out.println("Student Age: " + student.age);
}
}
In this class, we create an instance of the Student
class and directly access its public
variables: name
and age
.
When you run the Main
class, it will output:
Student Name: John Doe
Student Age: 21
This output demonstrates how we can access the public
variables directly from another class.
While this approach is more straightforward, it comes with the trade-off of reduced encapsulation and increased potential for unintended modifications.
Accessing Variables in Another Class Using getter
and setter
Methods
Accessing variables in another class using getter
and setter
methods is crucial for maintaining a controlled and secure interaction with the internal state of a class.
getters
allow the retrieval of variable values, while setters
enable the modification of these values. This encapsulation ensures that the internal details of a class, like variable names and implementations, remain hidden, promoting a more robust and maintainable code structure.
By using getter
and setter
methods, developers have the flexibility to implement additional logic, such as validation or calculations, when reading or modifying variables. This approach provides a clear and standardized way for other classes to access and update the state of an object, enhancing code readability and reducing the risk of unintended changes.
It also aligns with the principles of information hiding and abstraction, making the code more modular and adaptable. Overall, utilizing getter
and setter
methods establishes a secure and well-defined interface for interacting with variables in another class.
Let’s explore this concept with a practical example:
// Class Student
public class Student {
private String name;
private int age;
// Getter for 'name' variable
public String getName() {
return name;
}
// Setter for 'name' variable
public void setName(String name) {
this.name = name;
}
// Getter for 'age' variable
public int getAge() {
return age;
}
// Setter for 'age' variable
public void setAge(int age) {
if (age > 0) { // Adding a simple validation
this.age = age;
} else {
System.out.println("Invalid age!");
}
}
}
In the above code, we have a Student
class with private variables name
and age
. To provide access to these variables, we’ve defined getter
and setter
methods for each.
getter
methods like getName()
and getAge()
retrieve the values of private variables, allowing external classes to access this information without exposing the underlying implementation.
On the other hand, setter
methods such as setName(String name)
and setAge(int age)
enable the modification of these variables while enforcing specific rules or validations.
For instance, the setAge
method includes a simple validation to ensure that the age is positive.
In each setter
method, you’ll notice the use of the this
keyword, which refers to the current instance of the class. This is necessary to distinguish between the class variable and the method parameter when they share the same name.
Now, let’s see how we can use these methods in another class:
// Class Main
public class Main {
public static void main(String[] args) {
// Creating an instance of the Student class
Student student = new Student();
// Using setter methods to set values
student.setName("John Doe");
student.setAge(21);
// Using getter methods to retrieve values
String studentName = student.getName();
int studentAge = student.getAge();
// Displaying the retrieved values
System.out.println("Student Name: " + studentName);
System.out.println("Student Age: " + studentAge);
}
}
When you run the Main
class, it will output:
Student Name: John Doe
Student Age: 21
This demonstrates how the getter
and setter
methods facilitate controlled access to the private variables of the Student
class. Additionally, the setter
method for age
includes a simple validation, showcasing the flexibility of using methods to encapsulate logic related to variable manipulation.
Employing getter
and setter
methods in Java is a powerful way to ensure proper encapsulation and controlled access to class variables. It not only enhances code readability but also provides an avenue for implementing additional logic or validations when interacting with the internal state of objects.
Accessing Variables in Another Class Using protected
Access Modifier
The protected
access modifier in Java allows a variable to be accessed by classes within the same package or by subclasses, even if they are in different packages. This level of access is useful in scenarios where you want to expose variables to specific classes or their subclasses while maintaining a certain level of encapsulation.
Unlike public
access, which allows unrestricted access from any class, protected
access restricts visibility to a more limited scope, ensuring that variables are not exposed to classes outside the specified package or inheritance hierarchy.
Using the protected
access modifier promotes a more modular and organized code structure while still allowing for collaboration between specific classes.
Let’s dive into a practical example to illustrate how the protected
access modifier works.
// Class Animal
public class Animal {
protected String name; // Protected variable
// Constructor to initialize the protected variable
public Animal(String name) {
this.name = name;
}
}
In the code snippet above, we have a class Animal
with a protected
variable name
.
In the Animal
class, the use of the protected
keyword declares the variable name
with this modifier. This means that the variable is accessible by subclasses, providing a controlled level of visibility within the class hierarchy.
The class further includes a constructor that takes a parameter, name
, and utilizes it to initialize the protected
variable. This combination of protected
access and constructor initialization is a concise and effective way to manage the accessibility of variables within a class and its subclasses.
Now, let’s create a subclass that accesses the protected
variable.
// Class Dog (subclass of Animal)
public class Dog extends Animal {
private String breed; // Private variable specific to Dog
// Constructor to initialize variables in the subclass
public Dog(String name, String breed) {
super(name); // Call to the superclass constructor to initialize the protected variable
this.breed = breed;
}
// Method to display information about the Dog
public void displayInfo() {
System.out.println("Name: " + name); // Accessing protected variable from the superclass
System.out.println("Breed: " + breed); // Accessing private variable from the subclass
}
}
In the Dog
class, we extend the Animal
class, and the protected
variable name
is accessible due to the protected
modifier.
Now, let’s use these classes in a main class to showcase the output:
// Class Main
public class Main {
public static void main(String[] args) {
// Creating an instance of Dog
Dog myDog = new Dog("Buddy", "Golden Retriever");
// Calling the displayInfo method to output information
myDog.displayInfo();
}
}
When you run the Main
class, it will output:
Name: Buddy
Breed: Golden Retriever
This output demonstrates how the protected
access modifier allows the Dog
subclass to access the name
variable from the Animal
superclass.
The protected
variable facilitates controlled sharing of information between related classes while maintaining a level of encapsulation. This concept is particularly useful in class hierarchies where certain data needs to be shared among classes that are closely related.
Accessing Variables in Another Class Using Package-Private
Access Modifier
The package-private
, or default, access modifier is an often-overlooked option that provides a middle ground between public
and private
access.
The package-private
access modifier limits the visibility of a variable to the classes within the same package.
While not as restrictive as private
access, it ensures a degree of encapsulation, preventing the variable from being accessed by classes outside the package.
This can be particularly useful when you want to share information among related classes without exposing it to the entire application.
Let’s delve into a practical example to demonstrate the concept:
// Class Circle in package 'shapes'
package shapes;
class Circle {
double radius; // Package-private variable
// Constructor to initialize the package-private variable
Circle(double radius) {
this.radius = radius;
}
}
In the code snippet above, we have a class Circle
within a package named shapes
. The radius
variable is declared without an access modifier, making it package-private
by default.
In the given code snippet, the radius
variable is designated as package-private
by not having an explicit access modifier. This implies that it is accessible only within the same package.
The class also features a constructor taking a radius
parameter, responsible for initializing this package-private
variable. This design ensures that the radius
variable is confined to its package, limiting access to other classes outside the package and promoting a controlled and modular code structure.
Now, let’s create another class in the same package to access the package-private
variable.
// Class ShapeUtils in package 'shapes'
package shapes;
public class ShapeUtils {
// Method to calculate and return the area of a Circle
public static double calculateCircleArea(Circle circle) {
return Math.PI * Math.pow(circle.radius, 2);
}
}
In the ShapeUtils
class, we have a public method, calculateCircleArea
, that takes a Circle
object as a parameter. Notice that this class can access the package-private radius
variable from the Circle
class because they belong to the same package.
Now, let’s use these classes in a main class to showcase the output:
// Class Main in package 'shapes'
package shapes;
public class Main {
public static void main(String[] args) {
// Creating an instance of Circle
Circle myCircle = new Circle(5.0);
// Calculating and displaying the area of the Circle
double area = ShapeUtils.calculateCircleArea(myCircle);
System.out.println("Area of the Circle: " + area);
}
}
When you run the Main
class, it will output:
Area of the Circle: 78.53981633974483
This output demonstrates how the package-private
access modifier allows the ShapeUtils
class to access the radius
variable from the Circle
class within the same package.
The package-private
modifier strikes a balance between encapsulation and flexibility, making it a useful tool for managing class member visibility in a package-centric manner.
Accessing Variables in Another Class Using the Instance
Methods
Instance
methods act as intermediaries that provide a standardized way to retrieve or modify variables, allowing for additional logic, validation, or side effects.
Unlike direct variable access or other methods, using instance
methods abstracts the internal details of the class, preventing unintended modifications and promoting a clear, well-defined interface for external classes. This approach aligns with the principles of object-oriented programming, where each class encapsulates its data and behavior, and external classes interact with it through well-defined methods.
Instance
methods enhance code readability, maintainability, and flexibility, making it easier to manage and evolve a Java program.
Let’s dive into a practical example to illustrate the concept:
// Class Book
public class Book {
private String title; // Private variable
private String author; // Private variable
// Constructor to initialize private variables
public Book(String title, String author) {
this.title = title;
this.author = author;
}
// Instance method to get the title
public String getTitle() {
return title;
}
// Instance method to get the author
public String getAuthor() {
return author;
}
}
In the code snippet above, we have a class Book
with private variables title
and author
. The class provides instance methods getTitle
and getAuthor
to access these private variables.
In the Book
class, the variables title
and author
are intentionally marked as private, encapsulating the internal state of the class.
The constructor of the class initializes these private variables with values provided as parameters. To facilitate controlled access to these private variables, the class employs instance methods, namely getTitle
and getAuthor
.
These methods follow the standard naming convention for getter methods, allowing external classes to retrieve the values of private variables in a controlled manner.
This combination of private variables and instance methods adheres to the principles of encapsulation, providing a secure and well-defined way for external classes to interact with the internal state of the Book
class.
Now, let’s create another class that uses these instance methods to access the Book
class variables.
// Class Library
public class Library {
// Instance method to display information about a book
public void displayBookInfo(Book book) {
System.out.println("Title: " + book.getTitle());
System.out.println("Author: " + book.getAuthor());
}
}
In the Library
class, we have an instance method, displayBookInfo
, that takes a Book
object as a parameter and utilizes the getTitle
and getAuthor
methods to access the private variables.
Now, let’s use these classes in a main class to showcase the output:
// Class Main
public class Main {
public static void main(String[] args) {
// Creating an instance of Book
Book myBook = new Book("The Great Gatsby", "F. Scott Fitzgerald");
// Creating an instance of Library
Library myLibrary = new Library();
// Using the Library instance method to display book information
myLibrary.displayBookInfo(myBook);
}
}
When you run the Main
class, it will output:
Title: The Great Gatsby
Author: F. Scott Fitzgerald
This output demonstrates how the instance methods getTitle
and getAuthor
provide controlled access to the private variables of the Book
class.
By using methods to encapsulate the logic of variable access, the code becomes more modular, easier to maintain, and less prone to unintended modifications. Instance
methods play a crucial role in adhering to the principles of encapsulation and abstraction in Java programming.
Conclusion
The importance of accessing variables in another class lies in employing the most suitable method based on the specific requirements of the code.
public
access modifiers facilitate broad access when needed, while getter and setter methods offer controlled interaction, ensuring secure and standardized variable access.
The protected
access modifier strikes a balance, allowing access within a specific scope, often among closely related classes or subclasses.
package-private
access provides a more restricted visibility, confined to the same package.
Lastly, instance
methods contribute to encapsulation, promoting controlled and organized access to variables.
The choice among these methods depends on the desired level of visibility and control, emphasizing the flexibility and adaptability of Java programming practices.
Related Article - Java Variable
- Java Synchronised Variable
- Difference Between Static and Final Variables in Java
- How to Set JAVA_HOME Variable in Java
- How to Create Counters in Java
- How to Increase an Array Size in Java