Allergy Class in Java
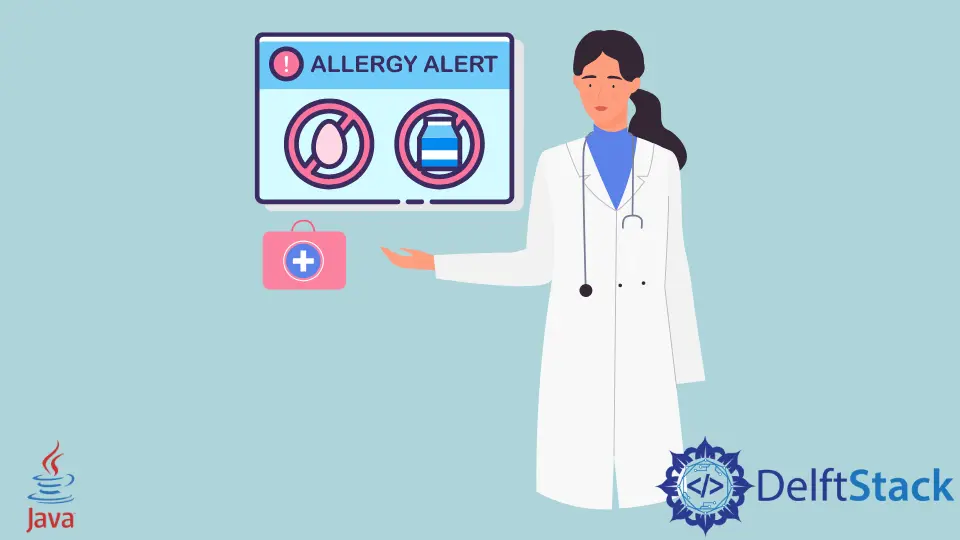
This tutorial demonstrates how to create a class named Allergy
, which checks if patients have an allergy or not in Java.
Create an Allergy Class in Java
An allergy
class means a class that can detect a patient’s allergy. We can create a class in Java for allergy, which will set and get the allergy name severity.
To support this system, we will need to create other classes to run the system based on the patient’s allergies or if the patient has an allergy or other diseases.
Let’s create a system to detect the patient’s allergy and see the steps.
- We need to create four classes.
- The first class is the
allergy
class which will set and gets the information about allergies. - The second class will be the
patient
class which will be used to set and get the patient’s name, age, and allergies they have. - The third class will be the
disease
class which will be used if the patient has another disease other than allergy. - The fourth and final class will be the
driver
class, which will be used to add the user info and symptoms and show the patient’s result.
Let’s try to implement the above system in Java.
First of all, the AllergyClass.java
:
package delftstack;
public class AllergyClass {
private String Allergy_Name;
private String Allergy_Severity;
private String Allergy_Symptoms;
public String GetAllergyName() {
return Allergy_Name;
}
public void SetAllergyName(String Allergy_Name) {
this.Allergy_Name = Allergy_Name;
}
public void SetAllergySymptom(String Allergy_Symptoms) {
this.Allergy_Symptoms = Allergy_Symptoms;
}
public String GetAllergySymptom() {
return Allergy_Symptoms;
}
public String GetSeverity() {
return Allergy_Severity;
}
public void SetSeverity(String Allergy_Severity) {
this.Allergy_Severity = Allergy_Severity;
}
}
The allergy
class will be used to set and get the allergy name, severity, and symptoms. Now the PatientClass.java
:
package delftstack;
import java.util.List;
public class PatientClass {
private String Patient_Name;
private int Patient_Age;
private List<AllergyClass> Allergy_List;
private List<DiseaseClass> Disease_List;
public String GetPatientName() {
return Patient_Name;
}
public void SetPatientName(String Patient_Name) {
this.Patient_Name = Patient_Name;
}
public int GetPatientAge() {
return Patient_Age;
}
public void SetPatientAge(int Patient_Age) {
this.Patient_Age = Patient_Age;
}
public List<AllergyClass> GetAllergyList() {
return Allergy_List;
}
public void SetAllergyList(List<AllergyClass> Allergy_List) {
this.Allergy_List = Allergy_List;
}
public List<DiseaseClass> GetDiseaseList() {
return Disease_List;
}
public void SetDiseaseList(List<DiseaseClass> Disease_List) {
this.Disease_List = Disease_List;
}
}
The patient
class will be used to set and get the patient’s name and age and assign them allergies or diseases. Now the DiseaseClass.java
:
package delftstack;
public class DiseaseClass {
private String Disease_Name;
private String Disease_Severity;
private String Disease_Symptoms;
public String GetDiseaseName() {
return Disease_Name;
}
public void SetDiseaseName(String Disease_Name) {
this.Disease_Name = Disease_Name;
}
public void SetDiseaseSymptom(String Disease_Symptoms) {
this.Disease_Symptoms = Disease_Symptoms;
}
public String GetDiseaseSymptom() {
return Disease_Symptoms;
}
public String GetSeverity() {
return Disease_Severity;
}
public void SetSeverity(String Disease_Severity) {
this.Disease_Severity = Disease_Severity;
}
}
The disease
class will perform the same operations as the allergy
class; this is an optional class in case the patient’s symptoms are not related to allergy. Now the main
class is Patient_Health_Info.java
:
package delftstack;
import java.util.ArrayList;
import java.util.List;
public class Patient_Health_Info {
public static void main(String[] args) {
// Create an instance of patient
PatientClass Demo_Patient = new PatientClass();
Demo_Patient.SetPatientName("Sheeraz");
Demo_Patient.SetPatientAge(28);
// Create an instance of Allergy
AllergyClass First_Allergy = new AllergyClass();
First_Allergy.SetAllergyName("Peanuts");
First_Allergy.SetAllergySymptom("Swelling/Chocking");
First_Allergy.SetSeverity("Severe");
// Create another instance of Allergy if a patient has multiple allergies
AllergyClass Second_Allergy = new AllergyClass();
Second_Allergy.SetAllergyName("Eggs");
Second_Allergy.SetAllergySymptom("Skin inflammation/Nasal congestion");
Second_Allergy.SetSeverity("Medium");
// Add Allergies to a list
List<AllergyClass> Allergy_List = new ArrayList<AllergyClass>();
Allergy_List.add(First_Allergy);
Allergy_List.add(Second_Allergy);
// Assign Allergies to the Patient.
Demo_Patient.SetAllergyList(Allergy_List);
String Patient_Diagnosis = PatientDiagnosis(Demo_Patient);
// Add Diseases to a list if present.
List<DiseaseClass> Disease_List = new ArrayList<DiseaseClass>();
// No diseases added
System.out.println("The Patient's Name is: " + Demo_Patient.GetPatientName());
System.out.println("The Patient's Age is: " + Demo_Patient.GetPatientAge());
System.out.println("The Patient has been diagnosed with :: " + Patient_Diagnosis);
if (Patient_Diagnosis == "Allergy") {
for (AllergyClass Patient_Allergy : Allergy_List) {
System.out.println("The Patient has a/an " + Patient_Allergy.GetAllergyName() + " allergy"
+ " with the symptoms of " + Patient_Allergy.GetAllergySymptom()
+ " and the allergy is " + Patient_Allergy.GetSeverity());
}
} else if (Patient_Diagnosis == "Disease") {
for (DiseaseClass Patient_Disease : Disease_List) {
System.out.println("The Patient has " + Patient_Disease.GetDiseaseName() + " Disease"
+ " with the symptoms of " + Patient_Disease.GetDiseaseSymptom()
+ " and the allergy is " + Patient_Disease.GetSeverity());
}
}
}
public static String PatientDiagnosis(PatientClass patient) {
if (patient.GetAllergyList().size() > 0) {
return "Allergy";
} else if (patient.GetDiseaseList().size() > 0) {
return "Disease";
}
return null;
}
}
This main
class will be used to insert and retrieve the info for a patient’s allergy or disease; it also determines if the patient has an allergy or any other disease. Let’s run the whole system and see the output.
The Patient's Name is: Sheeraz
The Patient's Age is: 28
The Patient has been diagnosed with :: Allergy
The Patient has a/an Peanuts allergy with the symptoms of Swelling/Chocking and the allergy is Severe
The Patient has a/an Eggs allergy with the symptoms of Skin inflammation/Nasal congestion and the allergy is Medium
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook