Friend Class in Java
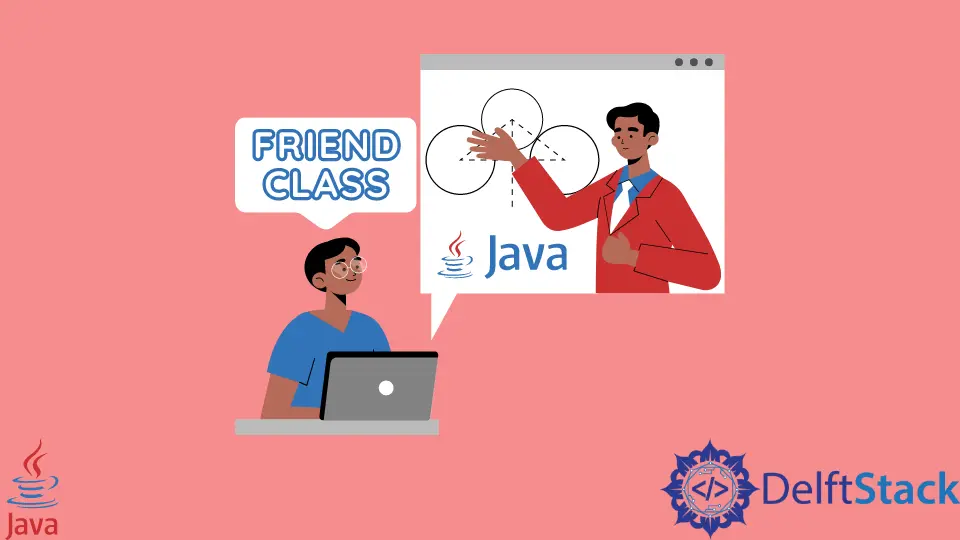
Friend class is the functionality of C++, which is used to access the non-public members of a class. Java doesn’t support the friend
keyword, but we can achieve the functionality.
This tutorial demonstrates how to create a friend class in Java.
Friend Class in Java
The friend concept can also be implemented in Java. For example, two colleagues from different departments of a company.
Both colleagues don’t know each other, but they need to cooperate for some work. Let’s set one employee as Jack
and the other as Michelle
based on a friend pattern.
We need to create two packages and implement both classes to implement this example.
The class Jack
in Department(package) Delftstack1
:
package Delftstack1;
import Delftstack2.Michelle;
public final class Jack {
static {
// Declare classes in the Delftstack2 package as 'friends'
Michelle.setInstance(new Michelle_Implement());
}
// Constructor is Only accessible by 'friend' classes.
Jack() {}
// This Method is Only accessible by 'friend' classes.
void HelloDelftstack() {
System.out.println("Hello! I am Jack from Delftstack");
}
static final class Michelle_Implement extends Michelle {
protected Jack createJack() {
return new Jack();
}
protected void sayHello(Jack jack) {
jack.HelloDelftstack();
}
}
}
The class Michelle
in Department(package) Delftstack2
:
package Delftstack2;
import Delftstack1.Jack;
public abstract class Michelle {
private static Michelle instance;
static Michelle getInstance() {
Michelle a = instance;
if (a != null) {
return a;
}
return createInstance();
}
private static Michelle createInstance() {
try {
Class.forName(Jack.class.getName(), true, Jack.class.getClassLoader());
} catch (ClassNotFoundException e) {
throw new IllegalStateException(e);
}
return instance;
}
public static void setInstance(Michelle michelle) {
if (instance != null) {
throw new IllegalStateException("Michelle instance already set");
}
instance = michelle;
}
protected abstract Jack createJack();
protected abstract void sayHello(Jack jack);
}
The class to implement the main method:
package Delftstack2;
import Delftstack1.Jack;
public final class Friend_Class {
public static void main(String[] args) {
Michelle michelle = Michelle.getInstance();
Jack jack = michelle.createJack();
michelle.sayHello(jack);
}
}
The code above implements the friend class functionality in Java with two classes in different packages. Class Michelle
acts as a friend class that accesses the class Jack
members.
Output:
Hello! I am Jack from Delftstack
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook