Java의 친구 클래스
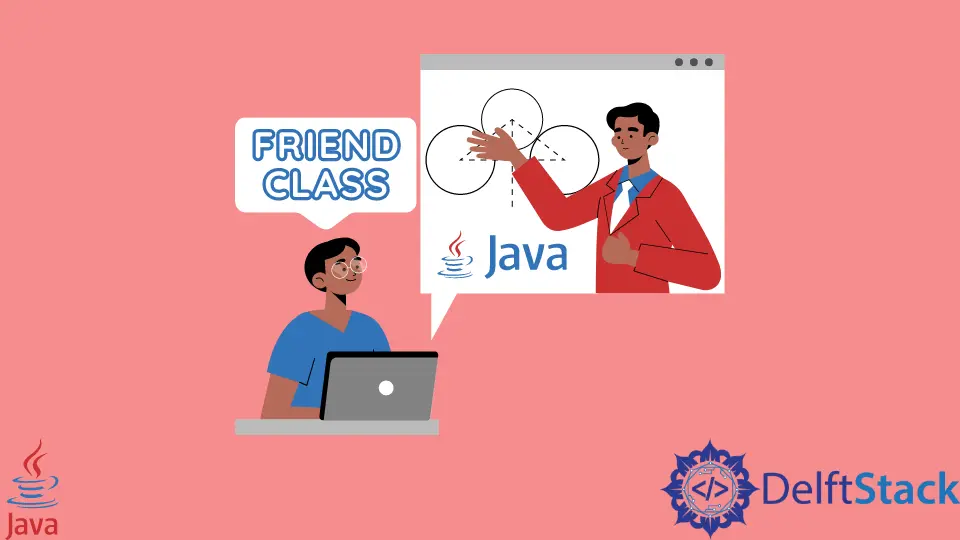
Friend 클래스는 클래스의 비공개 멤버에 액세스하는 데 사용되는 C++의 기능입니다. Java는 friend
키워드를 지원하지 않지만 기능을 구현할 수 있습니다.
이 자습서는 Java에서 친구 클래스를 만드는 방법을 보여줍니다.
Java의 친구 클래스
friend 개념은 Java에서도 구현할 수 있습니다. 예를 들어 회사의 다른 부서에서 온 두 명의 동료가 있습니다.
두 동료는 서로를 알지 못하지만 어떤 일을 위해 협력해야 합니다. 친구 패턴에 따라 직원 한 명을 Jack
으로, 다른 한 명을 Michelle
로 설정해 보겠습니다.
이 예제를 구현하려면 두 개의 패키지를 만들고 두 클래스를 모두 구현해야 합니다.
부서(패키지) Delftstack1
의 Jack
클래스:
package Delftstack1;
import Delftstack2.Michelle;
public final class Jack {
static {
// Declare classes in the Delftstack2 package as 'friends'
Michelle.setInstance(new Michelle_Implement());
}
// Constructor is Only accessible by 'friend' classes.
Jack() {}
// This Method is Only accessible by 'friend' classes.
void HelloDelftstack() {
System.out.println("Hello! I am Jack from Delftstack");
}
static final class Michelle_Implement extends Michelle {
protected Jack createJack() {
return new Jack();
}
protected void sayHello(Jack jack) {
jack.HelloDelftstack();
}
}
}
부서(패키지) Delftstack2
의 클래스 Michelle
:
package Delftstack2;
import Delftstack1.Jack;
public abstract class Michelle {
private static Michelle instance;
static Michelle getInstance() {
Michelle a = instance;
if (a != null) {
return a;
}
return createInstance();
}
private static Michelle createInstance() {
try {
Class.forName(Jack.class.getName(), true, Jack.class.getClassLoader());
} catch (ClassNotFoundException e) {
throw new IllegalStateException(e);
}
return instance;
}
public static void setInstance(Michelle michelle) {
if (instance != null) {
throw new IllegalStateException("Michelle instance already set");
}
instance = michelle;
}
protected abstract Jack createJack();
protected abstract void sayHello(Jack jack);
}
기본 메서드를 구현하는 클래스:
package Delftstack2;
import Delftstack1.Jack;
public final class Friend_Class {
public static void main(String[] args) {
Michelle michelle = Michelle.getInstance();
Jack jack = michelle.createJack();
michelle.sayHello(jack);
}
}
위의 코드는 서로 다른 패키지에 있는 두 개의 클래스를 사용하여 Java에서 friend 클래스 기능을 구현합니다. 클래스 Michelle
은 클래스 Jack
멤버에 액세스하는 친구 클래스 역할을 합니다.
출력:
Hello! I am Jack from Delftstack
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook