Java의 알레르기 클래스
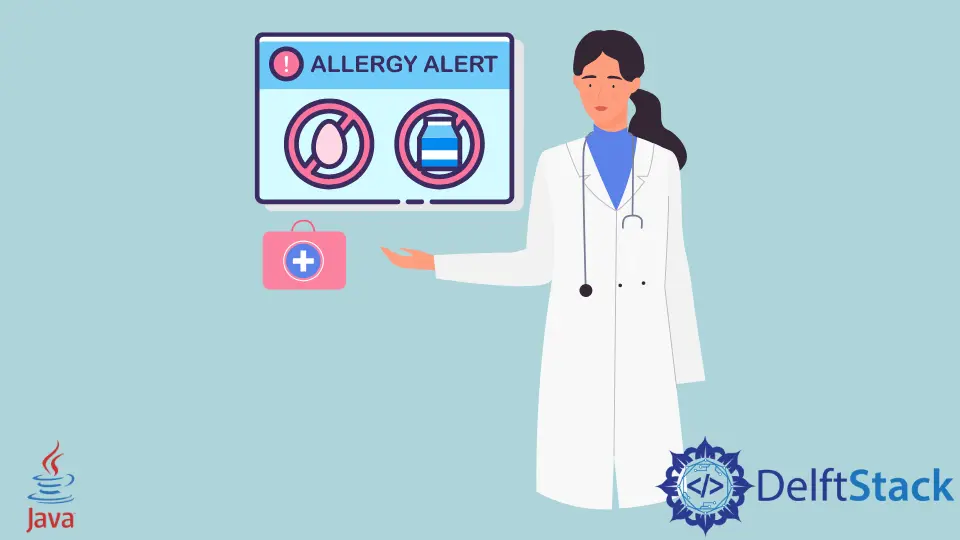
이 튜토리얼은 Java에서 환자에게 알레르기가 있는지 여부를 확인하는 Allergy
라는 클래스를 만드는 방법을 보여줍니다.
Java에서 알레르기 클래스 만들기
알레르기
등급은 환자의 알레르기를 감지할 수 있는 등급을 의미합니다. 알레르기 이름 심각도를 설정하고 가져오는 알레르기에 대한 클래스를 Java로 만들 수 있습니다.
이 시스템을 지원하려면 환자의 알레르기 또는 환자에게 알레르기 또는 기타 질병이 있는 경우 시스템을 실행하는 다른 클래스를 만들어야 합니다.
환자의 알레르기를 감지하고 단계를 볼 수 있는 시스템을 만들어 봅시다.
- 4개의 클래스를 생성해야 합니다.
- 첫 번째 클래스는 알레르기에 대한 정보를 설정하고 가져오는
알레르기
클래스입니다. - 두 번째 클래스는 환자의 이름, 나이, 알레르기를 설정하고 가져오는 데 사용되는
환자
클래스입니다. - 세 번째 클래스는 환자가 알레르기 이외의 다른 질병이 있는 경우 사용되는
질병
클래스입니다. - 네 번째이자 마지막 클래스는 사용자 정보와 증상을 추가하고 환자의 결과를 표시하는 데 사용되는
드라이버
클래스입니다.
위의 시스템을 Java로 구현해 봅시다.
먼저 AllergyClass.java
:
package delftstack;
public class AllergyClass {
private String Allergy_Name;
private String Allergy_Severity;
private String Allergy_Symptoms;
public String GetAllergyName() {
return Allergy_Name;
}
public void SetAllergyName(String Allergy_Name) {
this.Allergy_Name = Allergy_Name;
}
public void SetAllergySymptom(String Allergy_Symptoms) {
this.Allergy_Symptoms = Allergy_Symptoms;
}
public String GetAllergySymptom() {
return Allergy_Symptoms;
}
public String GetSeverity() {
return Allergy_Severity;
}
public void SetSeverity(String Allergy_Severity) {
this.Allergy_Severity = Allergy_Severity;
}
}
알레르기
클래스는 알레르기 이름, 심각도 및 증상을 설정하고 가져오는 데 사용됩니다. 이제 PatientClass.java
:
package delftstack;
import java.util.List;
public class PatientClass {
private String Patient_Name;
private int Patient_Age;
private List<AllergyClass> Allergy_List;
private List<DiseaseClass> Disease_List;
public String GetPatientName() {
return Patient_Name;
}
public void SetPatientName(String Patient_Name) {
this.Patient_Name = Patient_Name;
}
public int GetPatientAge() {
return Patient_Age;
}
public void SetPatientAge(int Patient_Age) {
this.Patient_Age = Patient_Age;
}
public List<AllergyClass> GetAllergyList() {
return Allergy_List;
}
public void SetAllergyList(List<AllergyClass> Allergy_List) {
this.Allergy_List = Allergy_List;
}
public List<DiseaseClass> GetDiseaseList() {
return Disease_List;
}
public void SetDiseaseList(List<DiseaseClass> Disease_List) {
this.Disease_List = Disease_List;
}
}
환자
클래스는 환자의 이름과 나이를 설정 및 가져오고 알레르기 또는 질병을 지정하는 데 사용됩니다. 이제 DiseaseClass.java
:
package delftstack;
public class DiseaseClass {
private String Disease_Name;
private String Disease_Severity;
private String Disease_Symptoms;
public String GetDiseaseName() {
return Disease_Name;
}
public void SetDiseaseName(String Disease_Name) {
this.Disease_Name = Disease_Name;
}
public void SetDiseaseSymptom(String Disease_Symptoms) {
this.Disease_Symptoms = Disease_Symptoms;
}
public String GetDiseaseSymptom() {
return Disease_Symptoms;
}
public String GetSeverity() {
return Disease_Severity;
}
public void SetSeverity(String Disease_Severity) {
this.Disease_Severity = Disease_Severity;
}
}
질병
클래스는 알레르기
클래스와 동일한 작업을 수행합니다. 이것은 환자의 증상이 알레르기와 관련이 없는 경우 선택 클래스입니다. 이제 main
클래스는 Patient_Health_Info.java
입니다.
package delftstack;
import java.util.ArrayList;
import java.util.List;
public class Patient_Health_Info {
public static void main(String[] args) {
// Create an instance of patient
PatientClass Demo_Patient = new PatientClass();
Demo_Patient.SetPatientName("Sheeraz");
Demo_Patient.SetPatientAge(28);
// Create an instance of Allergy
AllergyClass First_Allergy = new AllergyClass();
First_Allergy.SetAllergyName("Peanuts");
First_Allergy.SetAllergySymptom("Swelling/Chocking");
First_Allergy.SetSeverity("Severe");
// Create another instance of Allergy if a patient has multiple allergies
AllergyClass Second_Allergy = new AllergyClass();
Second_Allergy.SetAllergyName("Eggs");
Second_Allergy.SetAllergySymptom("Skin inflammation/Nasal congestion");
Second_Allergy.SetSeverity("Medium");
// Add Allergies to a list
List<AllergyClass> Allergy_List = new ArrayList<AllergyClass>();
Allergy_List.add(First_Allergy);
Allergy_List.add(Second_Allergy);
// Assign Allergies to the Patient.
Demo_Patient.SetAllergyList(Allergy_List);
String Patient_Diagnosis = PatientDiagnosis(Demo_Patient);
// Add Diseases to a list if present.
List<DiseaseClass> Disease_List = new ArrayList<DiseaseClass>();
// No diseases added
System.out.println("The Patient's Name is: " + Demo_Patient.GetPatientName());
System.out.println("The Patient's Age is: " + Demo_Patient.GetPatientAge());
System.out.println("The Patient has been diagnosed with :: " + Patient_Diagnosis);
if (Patient_Diagnosis == "Allergy") {
for (AllergyClass Patient_Allergy : Allergy_List) {
System.out.println("The Patient has a/an " + Patient_Allergy.GetAllergyName() + " allergy"
+ " with the symptoms of " + Patient_Allergy.GetAllergySymptom()
+ " and the allergy is " + Patient_Allergy.GetSeverity());
}
} else if (Patient_Diagnosis == "Disease") {
for (DiseaseClass Patient_Disease : Disease_List) {
System.out.println("The Patient has " + Patient_Disease.GetDiseaseName() + " Disease"
+ " with the symptoms of " + Patient_Disease.GetDiseaseSymptom()
+ " and the allergy is " + Patient_Disease.GetSeverity());
}
}
}
public static String PatientDiagnosis(PatientClass patient) {
if (patient.GetAllergyList().size() > 0) {
return "Allergy";
} else if (patient.GetDiseaseList().size() > 0) {
return "Disease";
}
return null;
}
}
이 main
클래스는 환자의 알레르기 또는 질병에 대한 정보를 삽입하고 검색하는 데 사용됩니다. 또한 환자에게 알레르기나 다른 질병이 있는지도 결정합니다. 전체 시스템을 실행하고 출력을 봅시다.
The Patient's Name is: Sheeraz
The Patient's Age is: 28
The Patient has been diagnosed with :: Allergy
The Patient has a/an Peanuts allergy with the symptoms of Swelling/Chocking and the allergy is Severe
The Patient has a/an Eggs allergy with the symptoms of Skin inflammation/Nasal congestion and the allergy is Medium
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook