Inner Class and Static Nested Class in Java
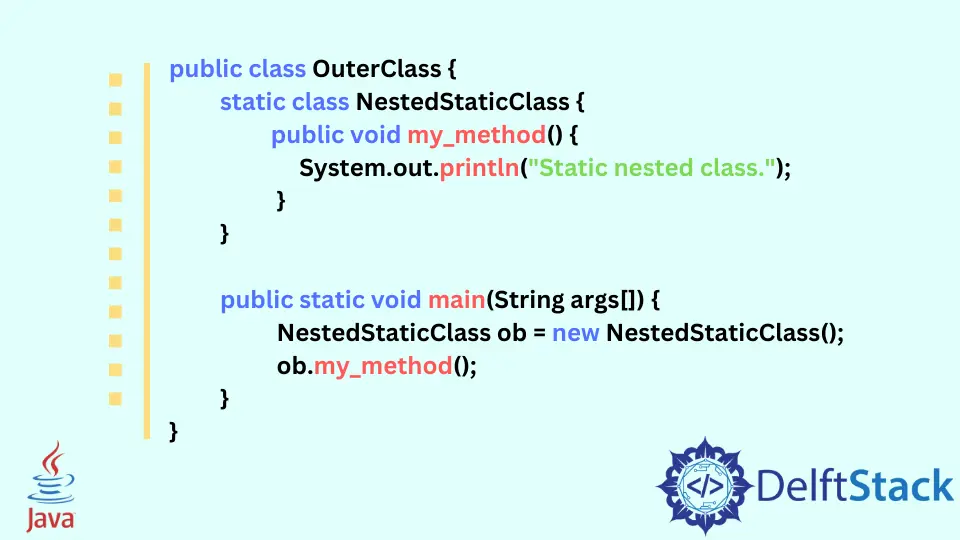
Java is a pure object-oriented programming language. You cannot create programs without a class.
Class in Java
A class is a blueprint or prototype representing a set of methods and properties that the class object can access. Let’s understand it through a real-life example.
Student
is a class which has some properties and methods like student name
, student roll no
, cal_gpa()
, cal_fee()
. These are some common properties for every student in a college or university.
It’s easy for us to create a class, define all the required properties, and access them with the object of the class for each student.
Code Example:
package articlecodesinjava;
class Student {
String name;
int rollNumber;
void cal_gpa() {
System.out.println("The method calculates CGPA.");
}
void cal_fee() {
System.out.println("The method calculates the Semester fee.");
}
}
public class ArticleCodesInJava {
public static void main(String[] args) {
// creating objects of Student class
Student Jinku = new Student();
Student Zeeshan = new Student();
System.out.println("This is Jinku's details.");
System.out.println(Jinku.name = "Jinku");
System.out.println(Jinku.rollNumber = 1);
Jinku.cal_fee();
Jinku.cal_gpa();
System.out.println("\n\nThis is Zeeshan's details.");
System.out.println(Zeeshan.name = "Zeeshan Afridi");
System.out.println(Zeeshan.rollNumber = 2);
Jinku.cal_fee();
Jinku.cal_gpa();
}
}
Output:
This is Jinku's details.
Jinku
1
The method calculates the Semester fee.
The method calculates CGPA.
This is Zeeshan's details.
Zeeshan Afridi
2
The method calculates the Semester fee.
The method calculates CGPA.
We have defined the blueprint of students as a class with basic information about them like name, roll number, CGPA, and fee. Now we can call these properties and methods for every student instead of defining all these for every student.
As you can see, we have created two objects, Jinku
and Zeeshan
, and called the properties and methods of the Student
class for these objects.
Structure of a Class in Java
Java is a strongly object-oriented programming language; even a single line of code requires a class and the main
method.
Syntax of a class:
AccessSpecifer classKeyword ClassName{
//Class body
}
Example:
Public class Student {
// Class body
}
AccessSpecifer
can bepublic
,private
, orprotected
based on the accessibility of the code.- The
class
keyword is mandatory to define a class; otherwise, you cannot create a class. ClassName
is also mandatory to create a class. Best practices and guides say that the names should be meaningful, making the code easier to understand and more readable.Class body
is the boundaries or limits of the class. You can define variables and methods inside the class body.
The class body starts with {
and ends with }
.
Types of Nested Classes in Java
A nested class refers to a class within another class. The phenomenon is similar to nested conditions and loops.
Java allows you to create nested classes. It enables you to group classes that are used in one place logically, and also it increases the use of encapsulation.
Syntax of a nested class:
class OuterClass { // OuterClass
Class InnerClass { // InnerClass }
}
In Java, there are two types of nested classes. These are the following:
- Static nested classes
- Inner classes
Static Nested Classes in Java
A static nested class is defined as a static member of its outer class with the keyword static
. A static nested class can be accessed without instantiating the outer class using other static members.
Similar to other static members, a static class doesn’t have access to its outer class’s methods and instance variables.
Code Example:
public class OuterClass {
static class NestedStaticClass { // Outer class
public void my_method() { // Inner class
System.out.println("This is a static nested class.");
}
}
public static void main(String args[]) { // Main function
// object of nested class
NestedStaticClass ob = new NestedStaticClass();
ob.my_method();
}
}
Output:
This is a static nested class.
Inner Classes in Java
Security is always the top priority of almost every software or organization. Java is well known for its security, and inner class is one of the components that help us achieve those security standards.
To access the inner class, first, you need to create an object of the outer class, and then using that object, you can make the object of the inner class.
Unlike a regular or simple class, an inner class has the authority to access the protected
and private
specified properties and methods. Also, you can declare the inner class as private
if you don’t want external objects to access the inner class.
Code Example:
public class OuterClass {
class NestedStaticClass { // Outer class
public void my_method() { // Inner class
System.out.println("This is a nested inner class.");
}
}
public static void main(String args[]) { // Main function
// object of nested class
OuterClass OuterObj = new OuterClass();
OuterClass.NestedStaticClass obj = OuterObj.new NestedStaticClass();
obj.my_method();
}
}
Output:
This is a nested inner class.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn