How to Make Private Inner Class Member Public in Java
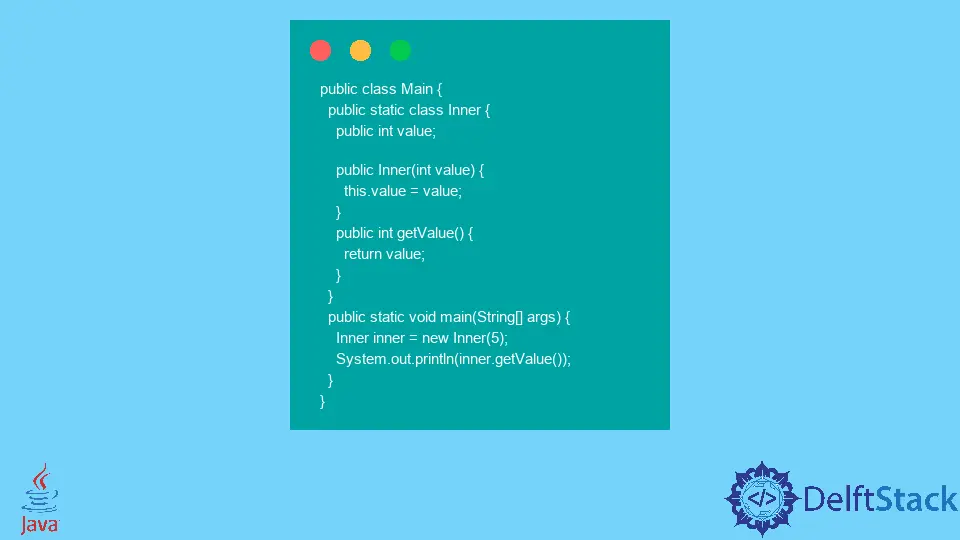
In Java, classes can have access levels such as private
, protected
, default
(package-private), and public
. When creating a class, the access level of its members (fields and methods) can be set using keywords like "private"
and "public"
.
By default, the access level of class members is default
, which means they are accessible only within the same package. However, sometimes it might be necessary to make a private
inner class member public
, and in this article, we will discuss why that could be the case.
Reasons to Make Private Inner Class Member Public in Java
1. Reusability
One of the main reasons to make a private
inner class public
is to increase its reusability. Making the inner class public
can be accessed and used by other classes, even those in different packages.
This can be useful when you want to create a common, reusable component that other parts of your application can use. For example, if you have an inner class representing a data structure, making it public
can allow other parts of your application to easily use it without having to recreate it.
2. Testing
Another reason to make a private
inner class public
is to facilitate testing. Making the inner class public
can be easily tested by external classes, including test classes.
This can be especially useful if the inner class contains complex logic that needs to be tested in isolation. For example, you might have a private
inner class that implements a sorting algorithm, and you want to write a test case to verify that the sorting logic works correctly.
By making the inner class public
, you can create a test case that invokes the sorting algorithm and verifies the results.
3. Flexibility
Making a private
inner class public
can also increase the flexibility of your code. For example, you might have a private
inner class representing a data structure.
You want to allow other parts of your application to extend this data structure to add new functionality. By making the inner class public
, you can allow other classes to inherit from it and extend its functionality, making your code more flexible and adaptable to future changes.
4. Debugging
Sometimes, making a private
inner class public
can make debugging easier. For example, you might have a private
inner class that implements a complex algorithm, and you want to step through the code in a debugger to understand how it works.
By making the inner class public
, you can directly access the inner class and its methods from a debugger, making it easier to debug and understand the implementation.
Example:
class Main {
private static class Inner {
private int value;
private Inner(int value) {
this.value = value;
}
private int getValue() {
return value;
}
}
public static void main(String[] args) {
Inner inner = new Inner(5);
System.out.println(inner.getValue());
}
}
In this example, the Inner
class is defined as a private
inner class within the Main
class. As a result, it is only accessible within the Main
class.
However, if we make the Inner
class public
and its members public, other classes can access and use this class, as shown below.
public class Main {
public static class Inner {
public int value;
public Inner(int value) {
this.value = value;
}
public int getValue() {
return value;
}
}
public static void main(String[] args) {
Inner inner = new Inner(5);
System.out.println(inner.getValue());
}
}
Now, other classes can access and use the Inner
class, making it more reusable, flexible, and easy to test and debug.
However, it is important to note that making a private
inner class public
should not be taken lightly and should only be done when there is a compelling reason to do so.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook