How to Create Counters in Java
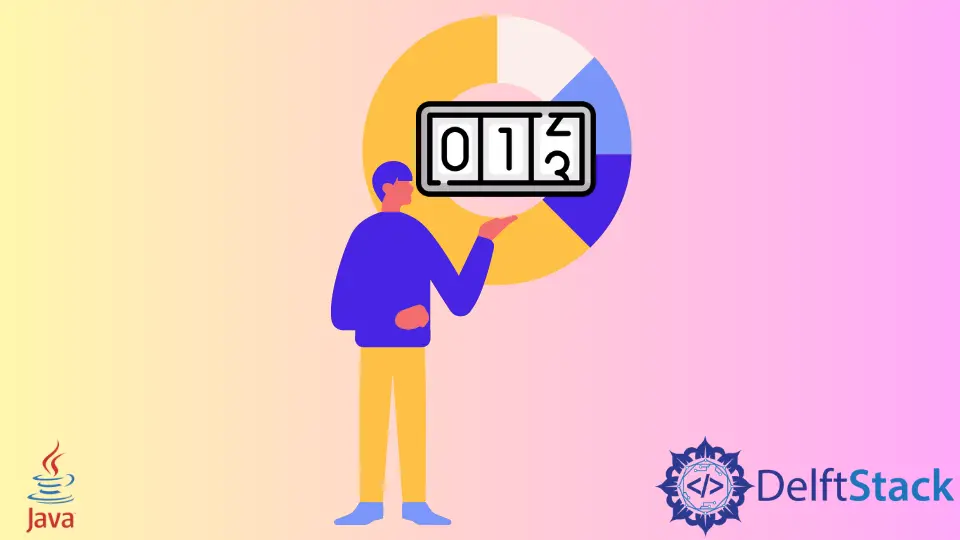
Today we will discuss the counter
variable in Java. In the following sections, we will see how we can use the counter
variable.
Counter in Loops
A counter is nothing but a variable name that specifies when we want a value to increment or decrement in a loop.
Below is an example that uses the counter
variable. The main()
method contains a counter
variable of type int
and is initialized with 0
.
We use the for
loop that runs ten times and in which with every iteration we increment the value counter
using counter++
, which is a shorthand format of counter = counter + 1
. To print the value of counter
we create a function printMsg()
that prints the counter
.
public class JavaExample {
public static void main(String[] args) {
int counter = 0;
for (int i = 0; i < 10; i++) {
counter++;
printMsg(counter);
}
}
static void printMsg(int counterValue) {
System.out.println("Your counter value is: " + counterValue);
}
}
Output:
Your counter value is: 1
Your counter value is: 2
Your counter value is: 3
Your counter value is: 4
Your counter value is: 5
Your counter value is: 6
Your counter value is: 7
Your counter value is: 8
Your counter value is: 9
Your counter value is: 10
Click Counter Using Java GUI Library
The counter
variable can also be useful when creating a click counter that counts the number of clicks on a button. For example, we need a window and a button to use the Java AWT library, a GUI library with several components like buttons, labels, etc.
We create a Frame
and set its size in the code. Then we create a Button
and a Label
. We use the label to print the number of clicks on the button.
We need an ActionListener
to listen to the button’s click action and for that we use the addActionListener()
to create the anonymous class ActionListener
and its actionPerformed()
function.
The actionPerformed()
is called when the button is clicked, and in this function, we increase the value of counter
by 1
. We create the counter
variable outside the main()
method and use the static
keyword because we cannot modify the variable if it is declared in the main()
method and used in the ActionListener
class.
After we increment the value of counter
in the actionPerformed()
method we convert the value to a String
using String.valueOf()
and then set it to the Label
component using label.setText()
.
At last, we add the components to the AWT frame
and set its visibility to true
. In the output, we can see a button, and when it is clicked, the click counter’s value increases by 1
.
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class JavaExample {
static int counter = 0;
public static void main(String[] args) {
Frame frame = new Frame("Counter Example");
frame.setSize(400, 300);
Button button = new Button("Click");
button.setBounds(100, 50, 100, 40);
Label label = new Label();
label.setBounds(100, 100, 200, 100);
button.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
counter++;
String counterAsString = String.valueOf(counter);
label.setText("Click Counter: " + counterAsString);
}
});
frame.add(button);
frame.add(label);
frame.setLayout(null);
frame.setVisible(true);
}
}
Output:
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn