How to Convert JSON to Map in Java
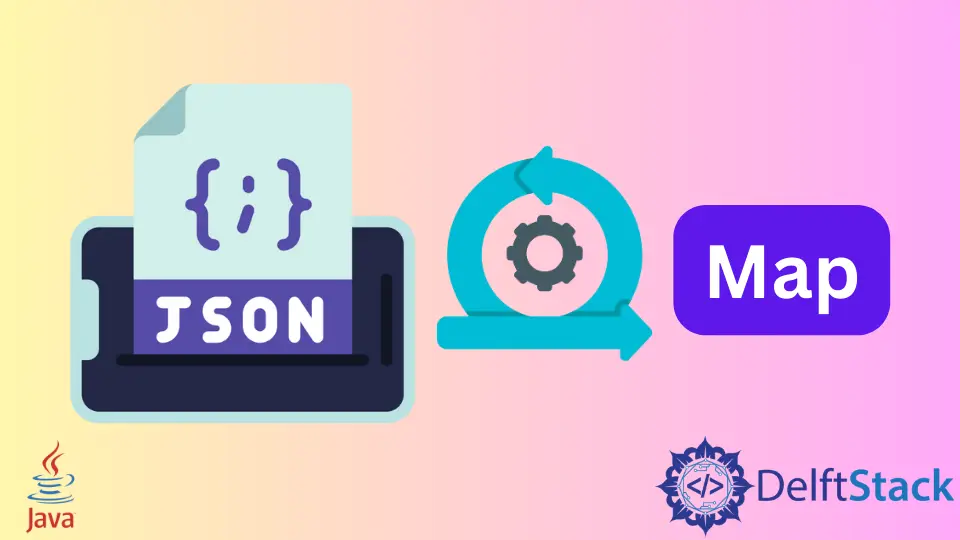
We will introduce how to convert an existing JSON to a Map in Java. JSON is often used to structure the data, but sometimes we have to get the JSON values into some other data type and then work on it.
Several libraries are created to work with JSON in Java. We will see the two most used JSON parsing libraries that allow us to do many JSON operations.
Below is the JSON that we are going to work on in this tutorial for a better understanding.
{
"login" :
{
"userName" : "John Doe",
"email" : "johndoe@john.com"
}
}
Jackson
Library to Convert JSON
Into Map
in Java
We have to include the below dependency in our project so that the Jackson
library’s methods and classes can be inherited.
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.11.2</version>
<scope>compile</scope>
</dependency>
After adding the dependency, we will first get the local JSON file using the FileInputStream()
method,
We need to call ObjectMapper().readValue()
from the Jackson
library to convert our JSON
to Map
. The readValue(JSON, ClassType)
function takes two arguments, the JSON
and the ClassType
that we want the JSON to be formatted. As we want to convert JSON
to Map
format, we will use Hashmap.class
.
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.HashMap;
public class Main {
public static void main(String[] args) throws IOException {
InputStream getLocalJsonFile = new FileInputStream("/sample.json");
HashMap<String, Object> jsonMap = new ObjectMapper().readValue(getLocalJsonFile, HashMap.class);
System.out.println(jsonMap);
}
}
Output:
{login={userName=John Doe, email=johndoe@john.com}}
GSON
Library to Convert JSON
to Map
in Java
First, we must include our project’s dependency to include all the GSON methods and classes in our application.
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.8.6</version>
</dependency>
GSON
is a widely used JSON library that we will parse and convert into Java Map.
Gson().fromJson(json, type)
expects two arguments, one of which is the JSON file which we can get by using new JsonReader(new FileReader(jsonFilePath))
and the second argument is the type which we want the JSON to be converted to.
Check out the below example.
import com.google.common.reflect.TypeToken;
import com.google.gson.Gson;
import com.google.gson.stream.JsonReader;
import java.io.FileReader;
import java.io.IOException;
import java.lang.reflect.Type;
import java.util.Map;
public class Main {
public static void main(String[] args) throws IOException {
JsonReader getLocalJsonFile = new JsonReader(new FileReader("/sample.json"));
Type mapTokenType = new TypeToken<Map<String, Map>>() {}.getType();
Map<String, String[]> jsonMap = new Gson().fromJson(getLocalJsonFile, mapTokenType);
System.out.println(jsonMap);
}
}
Output:
{login={userName=John Doe, email=johndoe@john.com}}
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java JSON
- How to Pretty-Print JSON Data in Java
- How to Serialize Object to JSON in Java
- How to Convert JSON Data to String in Java
- How to Deserialize JSON in Java
- How to Handle JSON Arrays in Java
- How to Convert JSON to Java object