How to Move File From Current Directory to a New Directory in Java
-
Use the
Files.move()
Method of Java NIO to Move File From the Current Directory to a New Directory -
Use the
renameTo()
Method to Move a File From the Current Directory to a New Directory
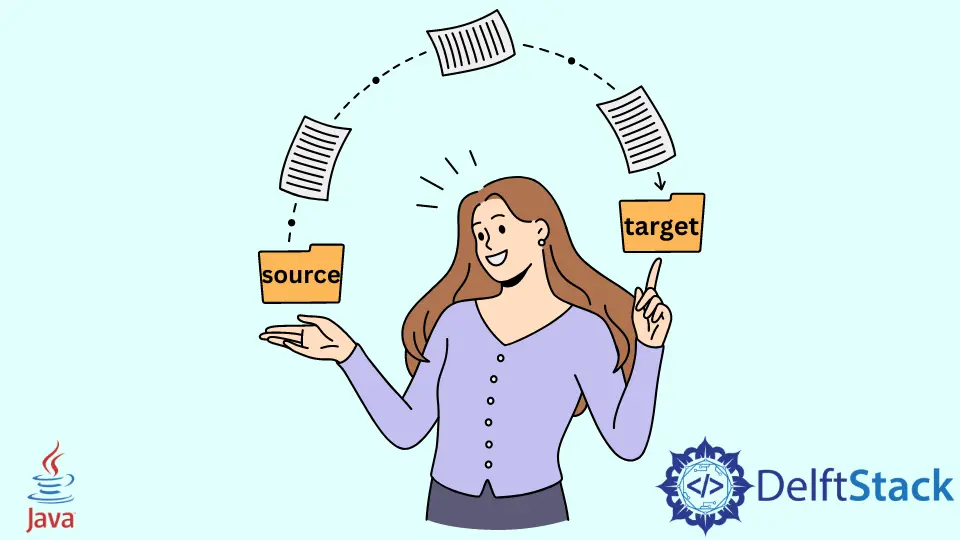
This tutorial presents different ways to move a file in Java. We will learn about the two methods to move a file from one directory to another directory locally (on the same machine).
These methods include the Files.move()
method of Java NIO (New Input Output) package and the renameTo()
method that is contained by Java.io.File package.
Use the Files.move()
Method of Java NIO to Move File From the Current Directory to a New Directory
We can use the following solution if we have Java 7 or above.
Example code:
import java.io.IOException;
import java.nio.file.*;
public class MoveFile {
public static void main(String[] args) {
Path sourcePath = Paths.get("./moveFile.txt");
Path targetPath = Paths.get(System.getProperty("user.home") + "/Desktop/Files/moveFile.txt");
try {
Files.move(sourcePath, targetPath);
} catch (FileAlreadyExistsException ex) {
System.out.println("The target path already has a target file");
} catch (IOException exception) {
System.out.format("I/O error: %s%n", exception);
}
}
}
This code snippet also moves the specified file from one location to another using the renameTo()
method.
The renameTo()
method takes a new abstract destination path, renames the file (if we specify a new name but do not rename it for this solution), and moves the file to the target location.
It returns true if the file is moved successfully, and we are printing a message to show that the file is moved and display a warning if the given file is not moved. You may see the code given above.