Introduction to Java NIO Package
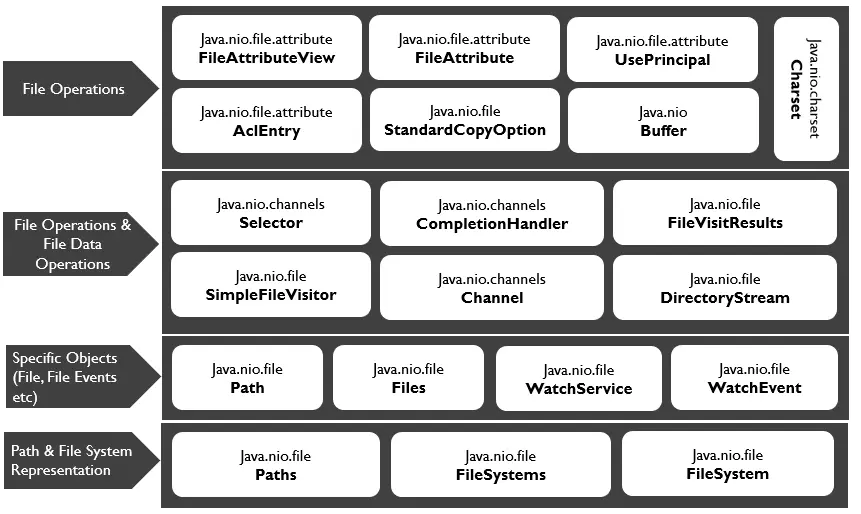
This tutorial introduces the Java NIO
package. We already have a java.io
package for performing read/write operations, but java.nio
allows us to work differently with additional features and functionalities.
How? Let’s dive into its details.
Introduction to Java NIO
Package
Java New Input/Output (NIO
) is a high-performance file handling structure and networking API. The java.nio
package has all the NIO
classes; it does not replace stream-based input/output (IO
) classes in the java.io
package.
The Java NIO
was introduced from the JDK 4
version works as an alternative to java.io
with some extra features and functionalities to work with Input/Output (IO
) differently compared to the standard Input/Output (IO
).
We already know that the java.io
package has all the necessary classes that we can use for Java I/O operations, while the java.nio
introduces buffer
classes that can be used throughout the Java NIO
APIs.
You may have a question: when we already have a package java.io
, what is the reason for introducing a new one named java.nio
?
Primary Reasons to Use Java NIO
Package
We prefer using the java.nio
package for two reasons.
-
The
java.nio
package uses a buffer-oriented approach that lets us move back and forth in the buffer. It uses a block of memory (also called a buffer) to read and cache data, which is accessed quickly from the buffer whenever needed; we can also write data into the buffer. -
The
java.nio
package can perform non-blocking Input/Output (IO
) operations. Here, non-blocking means it can read the data/information whichever it finds ready.For example, we have a thread that asks the channel to read the data/information from the buffer; the thread goes for other operations in that time frame and continues again where it left the work. Meanwhile, the reading process is completed and boosts the overall performance.
The primary difference between NIO
and IO
is that NIO
is non-blocking while IO
is blocking.
We can prioritize the use of Java NIO
over the IO
package whenever we are looking for buffer oriented approach and high-speed Input/Output operations.
NIO
package, you must understand the Java IO
package.Main Components of Java NIO
Package
It has the following main components to work properly.
-
Buffer
- As thejava.nio
package is buffer-oriented, it has buffers for primitive data types. The buffers are used as containers where we can read data from/to the buffer for further processing by using a channel. -
Channel
- The channel class ofjava.nio
is similar to streams (IO
streams) used for two-way communication. By using channels, we can do non-blocking input/output operations; we can also read/write data from/to buffer from the channels.The channels are used as a gateway where the connection to various entities is described by channels that can perform non-blocking
IO
operations. -
Selector
- We can use selectors for accomplishing non-blockingIO
operations. A selector (an object) monitors more than one channel for the events.We can say that selectors select the channels ready for performing Input/Output operations.
Use NIO
Package to Read and Write to a File in Java
Following is the graphical representation of various classes that can be used to perform different operations.
You can read about java.nio
in detail here, but we are focused on reading from file and writing to file-related operations in this tutorial; see the following examples.
Example Code (for reading from a file):
import java.io.IOException;
import java.nio.charset.Charset;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.List;
public class readFromFile {
public static void main(String[] args) {
Path filePath = Paths.get("file.txt");
Charset charSet = Charset.forName("ISO-8859-1");
try {
List<String> lines = Files.readAllLines(filePath, charSet);
for (String line : lines) {
System.out.println(line);
}
} catch (IOException e) {
System.out.println(e);
}
}
}
Output (read from a file and write to a file):
We read data from the specified file and print it on the command line in the first code snippet. In the second code example, we are writing the data (a small string) to the given file and printing it on the screen to tell what is being written in the file.
In the first example code, we call the Paths.get()
method the factory method for the Path
instance. In simple words, we can say that it creates a Path
instance whenever we call the static method named get()
of java.nio.file.Paths
class.
This method takes either a sequence of strings (these strings will be joined to make a path) or a string as parameters and converts it to the Path
instance. It throws InvalidPathException
if any illegal characters are in the string we pass.
Like File
, the java.nio.file.Path
may refer to either relative or an absolute path within the file system.
Charset
is used for character encoding. We are using ISO-8859-1
character encoding, but you can specify UTF-8
or any other that you want to use.
The readAllLines()
method reads a text file line by line. We save the read data into a List
of String
(see the code).
Further, we use a for
loop to display all the read lines on the screen.
In the second code example, all the logic is the same except this line of code.
Files.write(filePath, message.getBytes());
The File.write()
is a static method of the Java NIO
package used to write in the specified file.