Difference Between Hashmap and Map in Java
-
the
Map
Interface in Java -
the
HashMap
Class in Java -
Use a
Map
Reference to Hold Objects in Java -
Use a
Map
Reference to Hold Objects in Java
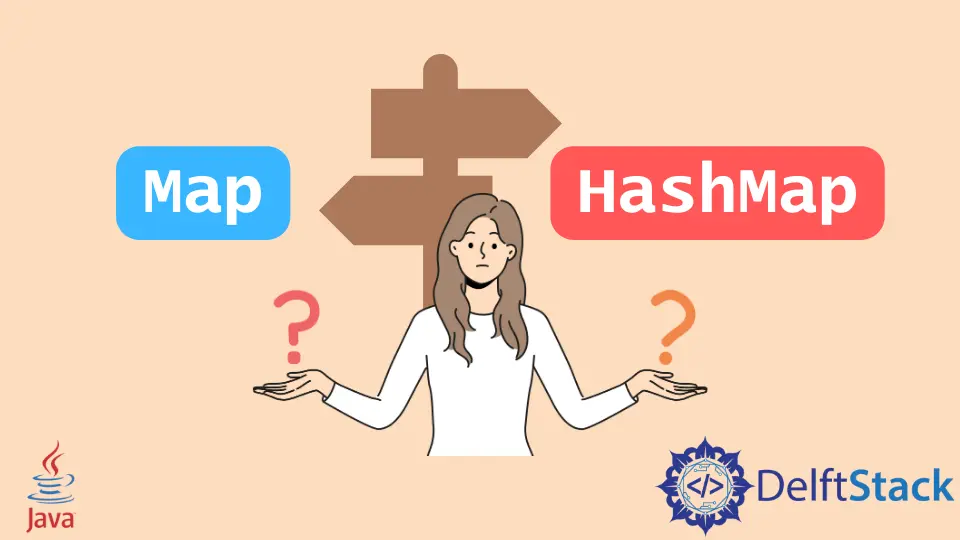
This tutorial introduces the main differences between Map
and HashMap
in Java.
In Java, Map
is an interface used to store data in key-value pair, whereas HashMap
is the implementation class of the Map
interface. Java has several classes (TreeHashMap
, LinkedHashMap
) that implement the Map
interface to store data into key-value pair. Let’s see some examples.
the Map
Interface in Java
The Map
interface alone can not be used to hold data, but we can create an object of its implementation classes and then use the Map
reference to hold the object. Here, we use the HashMap
class to store data and the Map
interface to hold the reference of this class. See the example below.
import java.util.HashMap;
import java.util.Map;
public class SimpleTesting {
public static void main(String[] args) {
Map<String, Integer> map = new HashMap<>();
map.put("One", 1);
map.put("Two", 2);
map.put("Three", 3);
System.out.println(map);
}
}
Output:
{One=1, Two=2, Three=3}
the HashMap
Class in Java
HashMap
is an implementation class of the Map
interface. So, we can use it to create a collection of key-value pairs. See the example below.
import java.util.HashMap;
public class SimpleTesting {
public static void main(String[] args) {
HashMap<String, Integer> map = new HashMap<>();
map.put("One", 1);
map.put("Two", 2);
map.put("Three", 3);
System.out.println(map);
}
}
Output:
{One=1, Two=2, Three=3}
Use a Map
Reference to Hold Objects in Java
Since Map
is an interface, we can use it to hold the reference of its implementation classes such as HashMap
, TreeMap
, etc. We can hold a TreeMap
or HashMap
object into the Map
interface. See the example below.
import java.util.HashMap;
import java.util.Map;
import java.util.TreeMap;
public class SimpleTesting {
public static void main(String[] args) {
Map<String, Integer> map = new HashMap<>();
map.put("One", 1);
map.put("Two", 2);
map.put("Three", 3);
System.out.println(map);
Map<String, Integer> tmap = new TreeMap<>(map);
System.out.println(tmap);
}
}
Output:
{One=1, Two=2, Three=3}
{One=1, Three=3, Two=2}
Use a Map
Reference to Hold Objects in Java
It is an important example of using the Map
reference while working with its implementation classes. See, we have a method that takes a Map
object as an argument. So, at the time of call, we can pass the object of any classes such as HashMap
or HashTable
. See the example below.
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.TreeMap;
public class SimpleTesting {
static void printMap(Map<String, Integer> map) {
for (String key : map.keySet()) {
System.out.println(key + ":" + map.get(key));
}
}
public static void main(String[] args) {
HashMap<String, Integer> hashmap = new HashMap<>();
hashmap.put("One", 1);
hashmap.put("Two", 2);
hashmap.put("Three", 3);
printMap(hashmap);
TreeMap<String, Integer> tmap = new TreeMap<>(hashmap);
printMap(tmap);
LinkedHashMap<String, Integer> lmap = new LinkedHashMap<>(hashmap);
printMap(lmap);
}
}