Introduction to HashMap in Java
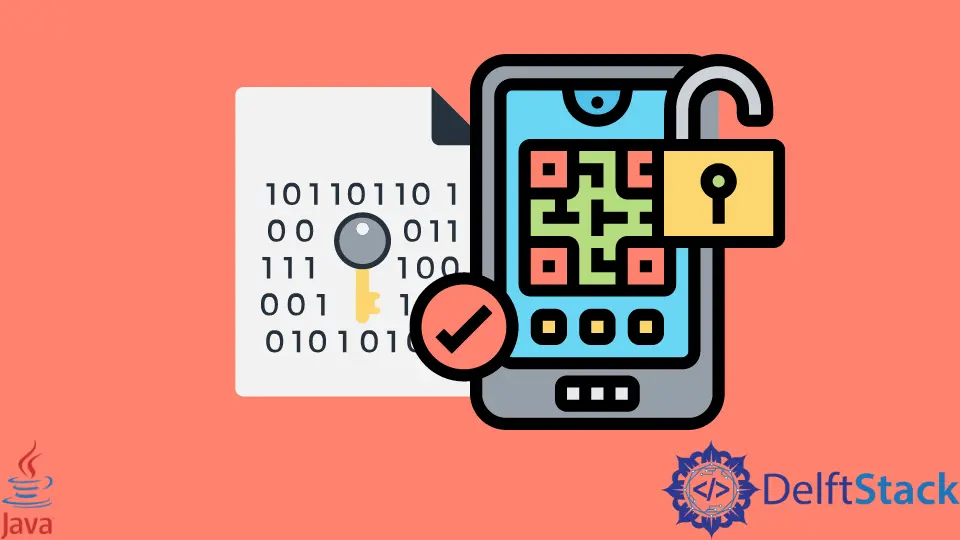
This tutorial is about the introduction to HashMap in Java. We will learn the HashMap in detail and understand how we can declare and manipulate the inserted data.
Introduction to HashMap in Java
If you are studying HashMap, you have a basic understanding of ArrayList
. In the array list, we store and access the elements of the array using the index (which is the int type number).
On the other hand, the HashMap stores elements in the key-value pair from where we can access the values by using keys of another data type. For instance, the keys are integer data type, and values are string data type, or both can be either an integer or string data type.
We can store values in an array list, so why use a HashMap? Is it just because to access value via keys?
No, it is because of performance.
Suppose we want to find a particular element in the list; it would take O(n)
time. The time complexity would be O(log n)
if the list is sorted using the binary search.
However, if we use the HashMap, the time complexity would be O(1)
(the constant time) to find the same value.
HashMap resides in java.util package and implement the Map interface. HashMap is similar to HashTable but unsynchronized.
Important Points to Remember While Using HashMap in Java
Someone who is using HashMap must consider the following points:
- Inserting the duplicate key replaces the value of the corresponding key.
- It has unique keys and values based on that key. Remember, the values can be redundant.
- Java HashMap preserves no order.
- HashMap is unsynchronized.
- Java HashMap may contain more than one null value and one null key.
- HashMap’s initial default capacity is 16 with a 0.75 load factor.
Declare and Insert Data Into the HashMap in Java
Declaring HashMap in Java depends on what kind of type of safety we want. If we wish to use a non-generic type, we can declare HashMap as follows.
Example code:
import java.util.HashMap; // import the HashMap class
public class Main {
public static void main(String[] args) {
// create the hashmap object named students
HashMap students = new HashMap();
// add data in key-value form
students.put(1, "Mehvish");
students.put(2, "Thomas");
students.put(3, "Christoper");
students.put(4, "John");
students.put("Jimi", "John");
System.out.println(students);
}
}
Output:
{1=Mehvish, 2=Thomas, 3=Christoper, 4=John, Jimi=John}
Here, we inserted the first four elements where the key is of integer data type, but the fifth element has a key of string data type. It means we don’t have consistency in the key’s data type.
It may occur for values as well. Due to this kind of situation and to avoid it, we can use a generic type and declare the HashMap as given below.
Example code:
import java.util.HashMap; // import the HashMap class
public class Main {
public static void main(String[] args) {
// create the hashmap object named students
HashMap<Integer, String> students = new HashMap<Integer, String>();
// add data in key-value form (roll number, name)
students.put(1, "Mehvish");
students.put(2, "Thomas");
students.put(3, "Christoper");
students.put(4, "John");
// print whole hashmap
System.out.println(students);
}
}
Output:
{1=Mehvish, 2=Thomas, 3=Christoper, 4=John}
Observe the HashMap declaration part; it has specific data types for the keys and values. We can’t insert the elements (key-value pair) that differ from the specified data types.
Sometimes, we need to change the implementations to LinkedHashMap or TreeMap in the future. In that case, declaring the HashMap as follows would be helpful.
Remember, the students
are types in the Map here. We must import the Map
class as import java.util.Map;
to declare the HashMap in the following way.
Map<Integer, String> students = new HashMap<Integer, String>();
Use the get()
Method to Access Values of the HashMap
Example code:
import java.util.HashMap; // import the HashMap class
public class Main {
public static void main(String[] args) {
// create the hashmap object named students
HashMap<Integer, String> students = new HashMap<Integer, String>();
// add data in key-value form (roll number, name)
students.put(1, "Mehvish");
students.put(2, "Thomas");
students.put(3, "Christoper");
students.put(4, "John");
// print whole hashmap
System.out.println(students);
System.out.println(students.get(2));
}
}
Output:
{1=Mehvish, 2=Thomas, 3=Christoper, 4=John}
Thomas
In this example, put()
is used to insert a particular element (the key-value pair) to the HashMap. It takes two parameters, the key and the value corresponding to that key.
The get()
method takes the key and retrieves the corresponding value from the HashMap.
Use the remove()
Method to Delete an Item From the HashMap
Example code:
import java.util.HashMap; // import the HashMap class
public class Main {
public static void main(String[] args) {
// create the hashmap object named students
HashMap<Integer, String> students = new HashMap<Integer, String>();
// add data in key-value form (roll number, name)
students.put(1, "Mehvish");
students.put(2, "Thomas");
students.put(3, "Christoper");
students.put(4, "John");
// print whole hashmap
System.out.println(students);
System.out.println(students.remove(2));
System.out.println(students);
}
}
Output:
{1=Mehvish, 2=Thomas, 3=Christoper, 4=John}
Thomas
{1=Mehvish, 3=Christoper, 4=John}
The remove()
function accepts the key and removes the corresponding item (the key-value pair) from the HashMap.
Here, we are printing the whole HashMap before and after deletion. You may see the difference and observe.
Use the replace()
Method to Update the Value in the HashMap
Example code:
import java.util.HashMap; // import the HashMap class
public class Main {
public static void main(String[] args) {
// create the hashmap object named students
HashMap<Integer, String> students = new HashMap<Integer, String>();
// add data in key-value form (roll number, name)
students.put(1, "Mehvish");
students.put(2, "Thomas");
students.put(3, "Christoper");
students.put(4, "John");
// print whole hashmap
System.out.println(students);
students.replace(1, "Sania") System.out.println(students);
}
}
Output:
{1=Mehvish, 2=Thomas, 3=Christoper, 4=John}
{1=Sania, 2=Thomas, 3=Christoper, 4=John}
This section uses the replace()
method that takes the key and the value we want to replace at the specified key. In this example, we print the complete HashMap before and after updating the value at the given key.
You can find other methods here and use them to update the value in the HashMap.