Java 中的 HashMap 介紹
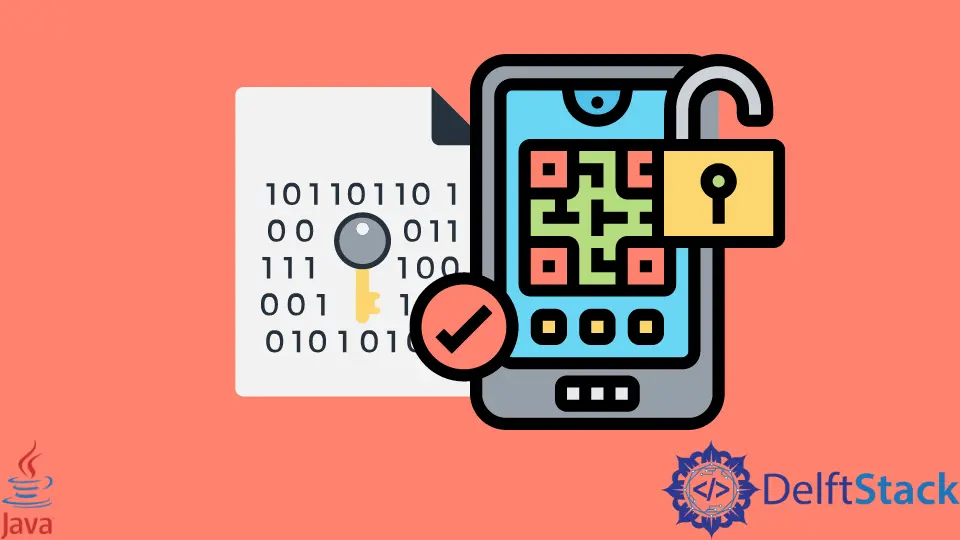
本教程是關於 Java 中 HashMap 的介紹。我們將詳細學習 HashMap 並瞭解如何宣告和操作插入的資料。
Java 中的 HashMap 介紹
如果你正在學習 HashMap,那麼你對 ArrayList
有一個基本的瞭解。在陣列列表中,我們使用索引(即 int 型別編號)儲存和訪問陣列的元素。
另一方面,HashMap 將元素儲存在鍵值對中,我們可以通過使用另一種資料型別的鍵訪問值。例如,鍵是整數資料型別,值是字串資料型別,或者兩者都可以是整數或字串數據型別。
我們可以將值儲存在陣列列表中,那麼為什麼要使用 HashMap 呢?僅僅是因為通過鍵訪問值嗎?
不,這是因為效能。
假設我們想在列表中找到一個特定的元素;這將花費 O(n)
時間。如果使用二進位制搜尋對列表進行排序,則時間複雜度將為 O(log n)
。
但是,如果我們使用 HashMap,時間複雜度將是 O(1)
(常數時間)來找到相同的值。
HashMap 位於 java.util 包中並實現 Map 介面。HashMap 類似於 HashTable 但不同步。
在 Java 中使用 HashMap 時要記住的要點
使用 HashMap 的人必須考慮以下幾點:
- 插入重複鍵替換對應鍵的值。
- 它具有基於該鍵的唯一鍵和值。請記住,這些值可能是多餘的。
- Java HashMap 不保留任何順序。
- HashMap 不同步。
- Java HashMap 可能包含多個空值和一個空鍵。
- HashMap 的初始預設容量為 16,負載因子為 0.75。
在 Java 中宣告並插入資料到 HashMap
在 Java 中宣告 HashMap 取決於我們想要什麼樣的安全型別。如果我們希望使用非泛型型別,我們可以如下宣告 HashMap。
示例程式碼:
import java.util.HashMap; // import the HashMap class
public class Main {
public static void main(String[] args) {
// create the hashmap object named students
HashMap students = new HashMap();
// add data in key-value form
students.put(1, "Mehvish");
students.put(2, "Thomas");
students.put(3, "Christoper");
students.put(4, "John");
students.put("Jimi", "John");
System.out.println(students);
}
}
輸出:
{1=Mehvish, 2=Thomas, 3=Christoper, 4=John, Jimi=John}
在這裡,我們插入了前四個元素,其中鍵是整數資料型別,但第五個元素的鍵是字串資料型別。這意味著我們在鍵的資料型別上沒有一致性。
它也可能發生在值上。由於這種情況併為避免這種情況,我們可以使用泛型型別並宣告 HashMap,如下所示。
示例程式碼:
import java.util.HashMap; // import the HashMap class
public class Main {
public static void main(String[] args) {
// create the hashmap object named students
HashMap<Integer, String> students = new HashMap<Integer, String>();
// add data in key-value form (roll number, name)
students.put(1, "Mehvish");
students.put(2, "Thomas");
students.put(3, "Christoper");
students.put(4, "John");
// print whole hashmap
System.out.println(students);
}
}
輸出:
{1=Mehvish, 2=Thomas, 3=Christoper, 4=John}
觀察 HashMap 宣告部分;它具有鍵和值的特定資料型別。我們不能插入與指定資料型別不同的元素(鍵值對)。
有時,我們將來需要將實現更改為 LinkedHashMap 或 TreeMap。在這種情況下,如下宣告 HashMap 會很有幫助。
請記住,students
是此處 Map 中的型別。我們必須將 Map
類匯入為 import java.util.Map;
通過以下方式宣告 HashMap。
Map<Integer, String> students = new HashMap<Integer, String>();
使用 get()
方法訪問 HashMap 的值
示例程式碼:
import java.util.HashMap; // import the HashMap class
public class Main {
public static void main(String[] args) {
// create the hashmap object named students
HashMap<Integer, String> students = new HashMap<Integer, String>();
// add data in key-value form (roll number, name)
students.put(1, "Mehvish");
students.put(2, "Thomas");
students.put(3, "Christoper");
students.put(4, "John");
// print whole hashmap
System.out.println(students);
System.out.println(students.get(2));
}
}
輸出:
{1=Mehvish, 2=Thomas, 3=Christoper, 4=John}
Thomas
在此示例中,put()
用於將特定元素(鍵值對)插入 HashMap。它有兩個引數,鍵和與該鍵對應的值。
get()
方法獲取鍵並從 HashMap 中檢索相應的值。
使用 remove()
方法從 HashMap 中刪除專案
示例程式碼:
import java.util.HashMap; // import the HashMap class
public class Main {
public static void main(String[] args) {
// create the hashmap object named students
HashMap<Integer, String> students = new HashMap<Integer, String>();
// add data in key-value form (roll number, name)
students.put(1, "Mehvish");
students.put(2, "Thomas");
students.put(3, "Christoper");
students.put(4, "John");
// print whole hashmap
System.out.println(students);
System.out.println(students.remove(2));
System.out.println(students);
}
}
輸出:
{1=Mehvish, 2=Thomas, 3=Christoper, 4=John}
Thomas
{1=Mehvish, 3=Christoper, 4=John}
remove()
函式接受鍵並從 HashMap 中刪除相應的項(鍵值對)。
在這裡,我們在刪除前後列印整個 HashMap。你可能會看到差異並進行觀察。
使用 replace()
方法更新 HashMap 中的值
示例程式碼:
import java.util.HashMap; // import the HashMap class
public class Main {
public static void main(String[] args) {
// create the hashmap object named students
HashMap<Integer, String> students = new HashMap<Integer, String>();
// add data in key-value form (roll number, name)
students.put(1, "Mehvish");
students.put(2, "Thomas");
students.put(3, "Christoper");
students.put(4, "John");
// print whole hashmap
System.out.println(students);
students.replace(1, "Sania") System.out.println(students);
}
}
輸出:
{1=Mehvish, 2=Thomas, 3=Christoper, 4=John}
{1=Sania, 2=Thomas, 3=Christoper, 4=John}
本節使用 replace()
方法,該方法獲取鍵和我們要在指定鍵處替換的值。在此示例中,我們在更新給定鍵的值之前和之後列印完整的 HashMap。
你可以在此處找到其他方法並使用它們來更新 HashMap 中的值。