How to Initialize HashMap in Java
- Initialize a HashMap Using the Traditional Way in Java
- Initialize a HashMap by Creating Anonymous Subclass in Java
-
Initialize a HashMap With Single Key-Value Pair Using
Collections.singletonMap()
in Java -
Initialize a HashMap Using
Collectors.toMap()
in Java 8 -
Initialize a HashMap Using
Map.of()
in Java 9
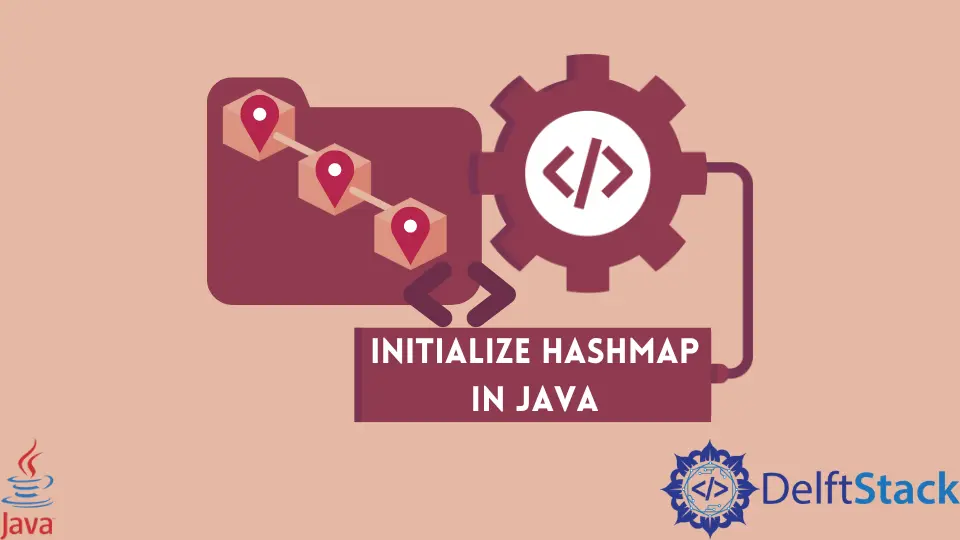
HashMap is a very convenient way to store data in a key-value pair. There are several ways to initialize a HashMap, and with every update, Java made it easier to achieve it.
Initialize a HashMap Using the Traditional Way in Java
The most common and standard way to initialize a HashMap is given in the program below.
We declare a Map
and put the key-value data types as String
in the example. We initialize the HashMap
using new HashMap()
.
This type of initialization generates a mutable type of HasMap
, and we insert some data in it using the put()
method where the first argument is the key
. The second argument is the value
.
In the last statement, we print the whole HashMap
.
import java.util.HashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) {
Map<String, String> hashMap = new HashMap<>();
hashMap.put("key1", "String1");
hashMap.put("key2", "String2");
hashMap.put("key3", "String3");
System.out.println(hashMap);
}
}
Output:
{key1=String1, key2=String2, key3=String3}
Initialize a HashMap by Creating Anonymous Subclass in Java
Another way to initialize a HashMap is to use double-braces and the put()
method to insert the data.
We first declare and then initialize a HashMap
with the data in the following code. The mapExample
is mutable, and we can put another data after the initialization.
This way of initializing the map is not recommended because it creates a new subclass with an anonymous class and an extra class is not a very efficient way to do it.
import java.util.HashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) {
Map<String, String> mapExample = new HashMap<>() {
{
put("Key01", "String01");
put("Key02", "String02");
put("Key03", "String03");
}
};
mapExample.put("Key04", "String04");
System.out.println(mapExample);
}
}
Output:
{Key01=String01, Key02=String02, Key03=String03, Key04=String04}
Initialize a HashMap With Single Key-Value Pair Using Collections.singletonMap()
in Java
This is a way to initialize a HashMap by only inserting a single key-value pair data in it. We use the static method singletonMap()
of the Collections
class, which returns an immutable map with only a single entry.
In the below example, we call the Collections.singletonMap()
function and pass the key-value data. The Map
is immutable, so we cannot modify or insert more data after initialization.
import java.util.Collections;
import java.util.Map;
public class Main {
public static void main(String[] args) {
Map<String, String> mapExample = Collections.singletonMap("key1", "value1");
System.out.println(mapExample);
}
}
Output:
{key1=value1}
Initialize a HashMap Using Collectors.toMap()
in Java 8
In the Java 8 update, the Stream API was introduced, a sequence of elements containing methods to perform operations on various types in Java, like Collections and Arrays.
The below program shows the use of Stream
. We use the Stream API to call the of()
method that sequentially returns a single element in a Stream
.
In the of()
method, we pass the object type of the element we want to return. We specify new String[][]
, which is an array of String
type, and then in the array, we insert the key-value pairs.
To convert the sequential stream of String
array elements to a Map
, we call the collect()
method, and in this function, we call Collectors.toMap()
that maps the passed data into a Map
.
In the Collectors.toMap()
method, we take the first element of the array and put it as a key and the second as the value. After all this, the stream
returns a single Map
that we store in mapExample
.
import java.util.Map;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class Main {
public static void main(String[] args) {
Map<String, String> mapExample =
Stream.of(new String[][] {{"key1", "21"}, {"key2", "22"}, {"key3", "33"}})
.collect(Collectors.toMap(
collectorData -> collectorData[0], collectorData -> collectorData[1]));
System.out.println(mapExample);
}
}
Output:
{key1=21, key2=22, key3=33}
Initialize a HashMap Using Map.of()
in Java 9
Another way to initialize a HashMap is to use the Map.of()
function of the Java 9 version. Map.of()
maps the keys and values passed as pairs, and then it returns an immutable HashMap
.
Note that Map.of()
can take a maximum of up to ten entries only.
import java.util.Map;
public class Main {
public static void main(String[] args) {
Map<String, String> mapExample = Map.of("key1", "Value1", "key2", "Value2", "key", "Value3");
System.out.println(mapExample);
}
}
Output:
{key=Value3, key1=Value1, key2=Value2}
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn