Differences between HashMap, HashSet and Hashtable in Java
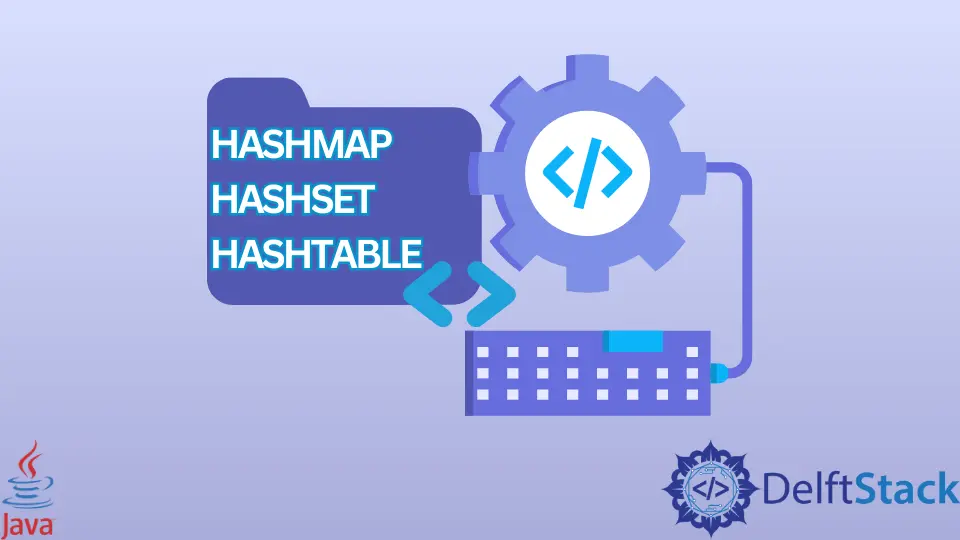
Java’s Collection
interface provides us with various interfaces and classes to implement a variety of data structures and algorithms.
This tutorial will discuss HashMap
, HashSet
, and Hashtable
in Java.
First, let us understand what hash tables are in general.
We can use hash tables to store elements in a key-value pair pattern, which means that every key has a value associated with it. Key is a unique value that is used to index the values. Value is the data related to the corresponding key.
The hash table data structure follows the concept of hashing, where a new index is processed using the keys. The element which corresponds to that key is then stored in the index. This is the concept of Hashing.
Let h(x)
be the hash function, and k
is a key, then h(k)
will give a new index to store the elements linked with k
.
Java HashMap
The HashMap
is a class of Java’s collections framework that provides us with the hash table data structure. It stores the elements as a key-value pair, where keys are the unique identifiers paired to a specific value on a map. The HashMap
class implements the Map interface, which further extends the Collections interface.
The HashMap
is unsynchronized, which means it is not thread-safe. We can access it using multiple threads and modify it at the same time. It can be made thread-safe externally.
Another characteristic of HashMap
is that it can hold a null key or value pairs.
For Example,
import java.util.HashMap;
public class Main {
public static void main(String[] args) {
HashMap<String, Integer> h = new HashMap<>();
h.put("One", 1);
h.put("Two", 2);
h.put("Three", 3);
System.out.println(h);
}
}
Output:
HashMap: {One=1, Two=2, Three=3}
Java Hashtable
The Hashtable
class of Java implements the hash table data structure. Similar to HashMap
, it also stores the elements as a key-value pair. But it differs from a HashMap
as it is synchronized. It stores the key-value pair in the hash table. It implements the Map interface.
First, in the Hashtable
, we specify the object as the key, with its value as a pair. The key is then hashed, and then we use the resulting hash code is used as an index for the value stored within the table.
Owing to this, the problem associated with Hashtable
is that synchronizing each method call is relatively not insignificant. It is not required every time. Hence to overcome this issue, authors of the collections framework came up with a new class called the HashMap
(which also clarifies that it maps the elements) which is unsynchronized.
If one does not want to use method-level synchronization, one could skip the Hashtable
and use Collections.synchronizedMap()
that turns a map into a synchronized map. Alternatively, we might use ConcurrentHashMap
, which according to its documentation, offers the same functionality as Hashtable
but has better performance and some additional functionalities.
For example,
import java.io.*;
import java.util.*;
public class Main {
public static void main(String args[]) {
Hashtable<Integer, String> h = new Hashtable<>();
h.put(1, "one");
h.put(2, "two");
h.put(3, "three");
System.out.println(h);
}
}
Output:
{3=three, 2=two, 1=one}
Java HashSet
The HashSet
is a class of Java’s collections framework that provides us with the implementation of hash table data structure (just like HashMap
). Still, it implements the Set
interface (Unlike HashMap
), which further extends the Collections interface. This is generally used when we do not need to map keys to value pairs.
The HashSet
differs from the Hashtable
in a way that HashSet
cannot hold duplicate values. The key-value pairs are unique. In terms of functionality, HashSet
has nothing in common with the HashMap
whatsoever, but it happens to use a HashMap
internally to implement the Set interface.
For example,
import java.util.HashSet;
public class Main {
public static void main(String[] args) {
HashSet<Integer> h = new HashSet<>();
h.add(5);
h.add(10);
h.add(15);
System.out.println(h);
}
}
Output:
[5, 10, 15]
Related Article - Java HashMap
- Introduction to HashMap in Java
- How to Initialize HashMap in Java
- Collision in Hashmap in Java
- How to Sort a HashMap by Key in Java
- How to Sort HashMap in Java