Difference Between Hashtable and Hashmap in Java
-
Hashtable
vsHashMap
-
Create
Hashtable
in Java -
Create
HashMap
in Java -
Store
null
inHashMap
in Java -
Store
null
inHashtable
in Java
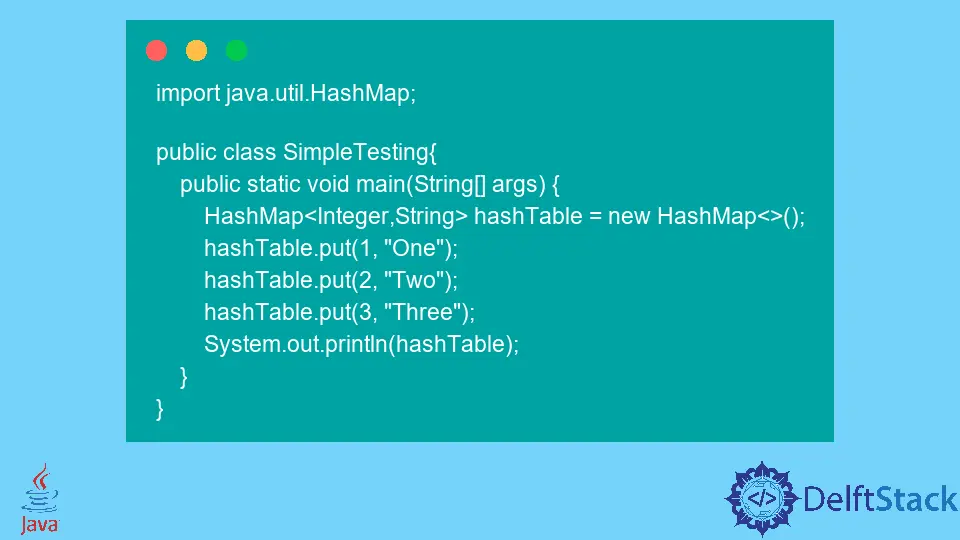
This tutorial introduces the differences between Hashtable
and HashMap
in Java also lists some example codes to understand the topic.
Hashtable is a class in Java collections framework that is used to store data in key-value pairs. It is a legacy class in Java and works on the hash concept to store elements. In the latest Java versions, Hashtable is not recommended. We should rather use HashMap that is more advanced than Hashtable. See the below table that summarizes key differences between Hashtable and HashMap in Java.
Hashtable
vs HashMap
Hashtable |
HashMap |
---|---|
Synchronized | Non-synchronized |
Null not allowed | Allow null |
Legacy class | Not legacy |
It is slow | It is fast |
In the above comparison table, we can clearly see the major differences between Hashtable and HashMap. Now, Let’s see some examples.
As Hashtable
is internally synchronized, this makes Hashtable
slightly slower than the HashMap
.
Create Hashtable
in Java
In this example, we are creating Hashtable. It is the simplest way to create Hashtable, and we just need to import Hashtable in our code. This class is located in the java.util
package. See the example below.
import java.util.Hashtable;
public class SimpleTesting {
public static void main(String[] args) {
Hashtable<Integer, String> hashTable = new Hashtable<>();
hashTable.put(1, "One");
hashTable.put(2, "Two");
hashTable.put(3, "Three");
System.out.println(hashTable);
}
}
Output:
{3=Three, 2=Two, 1=One}
Create HashMap
in Java
In this example, we are creating HashMap
in Java. It is the simplest way to create HashMap, and we just need to import HashMap in our code. This class is located in the java.util
package. See the example below.
import java.util.HashMap;
public class SimpleTesting {
public static void main(String[] args) {
HashMap<Integer, String> hashTable = new HashMap<>();
hashTable.put(1, "One");
hashTable.put(2, "Two");
hashTable.put(3, "Three");
System.out.println(hashTable);
}
}
Output:
{3=Three, 2=Two, 1=One}
Store null
in HashMap
in Java
The HashMap
allows null to be stored, which means we can store a null key and multiple null values in the HashMap
. This class is useful when you have null in your collections. See the example below.
import java.util.HashMap;
public class SimpleTesting {
public static void main(String[] args) {
HashMap<Integer, String> hashTable = new HashMap<>();
hashTable.put(1, "One");
hashTable.put(2, "Two");
hashTable.put(3, "Three");
hashTable.put(null, "Four");
hashTable.put(null, null);
System.out.println(hashTable);
}
}
Output:
{null=null, 1=One, 2=Two, 3=Three}
Store null
in Hashtable
in Java
The Hashtable
does not allow null to be stored, which means we cannot store a null in the Hashtable
. This class is not useful when you have null in your collections. See the example below.
It throws a NullPointerException
exception if a null value is stored.
import java.util.Hashtable;
public class SimpleTesting {
public static void main(String[] args) {
Hashtable<Integer, String> hashTable = new Hashtable<>();
hashTable.put(1, "One");
hashTable.put(2, "Two");
hashTable.put(3, "Three");
hashTable.put(null, "Four");
hashTable.put(null, null);
System.out.println(hashTable);
}
}
Output:
Exception in thread "main" java.lang.NullPointerException