How to Sort HashMap in Java
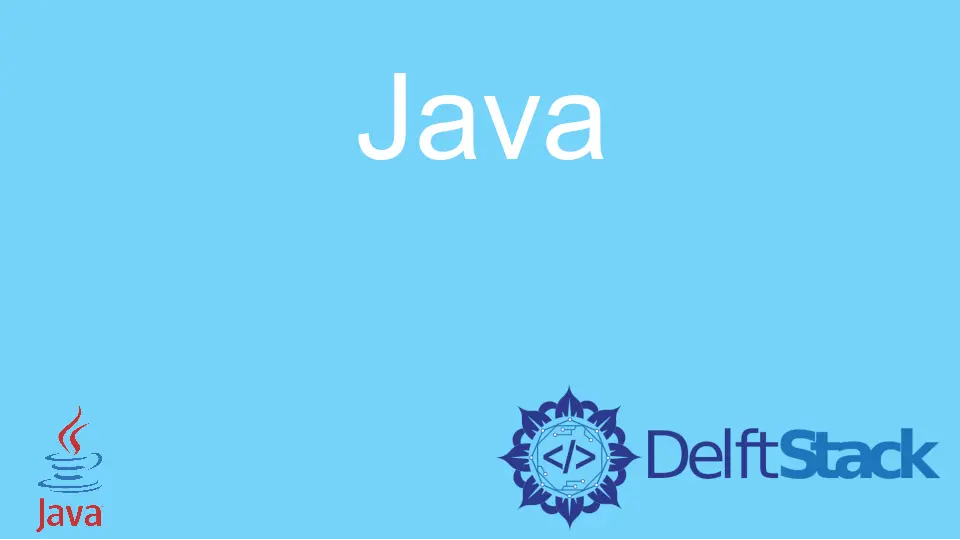
Hashmaps aren’t made for sorting. They are made for quick retrieval. So, a far easy method would be to take each element from the Hashmap and place them in a data structure that’s better for sorting, like a heap or a set, and then sort them there.
But if your idea is to sort using a Hashmap, we will discuss a few methods in this tutorial.
If we need to sort HashMap, we do it explicitly according to the required criteria. We can sort HashMaps by keys or by value in Java.
Sort a HashMap by Keys in Java
Using the keys, we can sort a HashMap in two ways: a LinkedHashMap
or a TreeMap
.
While using the LinkedHashMap
approach, it is vital to obtain a key set. Upon receiving such a key set, we translate these into a list. This list is then sorted accordingly and added to the LinkedHashMap
in the same order. There is no duplicity of keys in sorting HashMap by keys.
Code:
import static java.util.stream.Collectors.*;
import java.lang.*;
import java.util.*;
import java.util.stream.*;
import java.util.stream.Collectors;
public class Main {
// unsorted
static Map<String, Integer> lhmap = new HashMap<>();
public static void sort() {
HashMap<String, Integer> x = lhmap.entrySet()
.stream()
.sorted((i1, i2) -> i1.getKey().compareTo(i2.getKey()))
.collect(Collectors.toMap(Map.Entry::getKey,
Map.Entry::getValue, (z1, z2) -> z1, LinkedHashMap::new));
// Show Sorted HashMap
for (Map.Entry<String, Integer> start : x.entrySet()) {
System.out.println("Your Key " + start.getKey() + ", Your Value = " + start.getValue());
}
}
public static void main(String args[]) {
// insert value
lhmap.put("a", 1);
lhmap.put("aa", 3);
lhmap.put("ab", 5);
lhmap.put("ba", 7);
lhmap.put("bb", 8);
lhmap.put("aac", 23);
lhmap.put("bac", 8);
sort();
}
}
Output:
Your Key a, Your Value = 1
Your Key aa, Your Value = 3
Your Key aac, Your Value = 23
Your Key ab, Your Value = 5
Your Key ba, Your Value = 7
Your Key bac, Your Value = 8
Your Key bb, Your Value = 8
We can also use a TreeMap
. One of the implementations of SortedMap
is a TreeMap
which helps keep keys in natural order or custom order specified by the comparator provided while creating TreeMap
. This implies that the user can process entries of the HashMap in sorted order. However, the user cannot pass a HashMap having mappings in a specific order because HashMap doesn’t guarantee an order.
Code:
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.HashMap;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.TreeMap;
public class SortHashmap {
public static void main(String args[]) {
// Create a HashMap object ob
HashMap<Integer, String> ob = new HashMap<Integer, String>();
// addding keys and values
ob.put(23, "Vedant");
ob.put(7, "Aryan");
ob.put(17, "Tarun");
ob.put(9, "Farhan");
Iterator<Integer> it = ob.keySet().iterator();
System.out.println("Before Sorting");
while (it.hasNext()) {
int key = (int) it.next();
System.out.println("Roll number: " + key + " name: " + ob.get(key));
}
System.out.println("\n");
Map<Integer, String> map = new HashMap<Integer, String>();
System.out.println("After Sorting");
// using the TreeMap constructor in order to sort the HashMap
TreeMap<Integer, String> tm = new TreeMap<Integer, String>(ob);
Iterator itr = tm.keySet().iterator();
while (itr.hasNext()) {
int key = (int) itr.next();
System.out.println("Roll no: " + key + " name: " + ob.get(key));
}
}
}
Sort a HashMap by Values in Python
This method aims to keep the entry items in a list, and then we sort this list of items depending on their value. These values and keys are then retrieved from the items’ list and then placed accordingly in a new HashMap.
Due to this new HashMap is sorted entirely based on the values.
To compare items based on their values, we must create a comparator
. While using this approach, we have to keep in mind that we can store duplicate values.
See the code below.
import java.util.Collections;
import java.util.Comparator;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
public class SortingByValue // implementing the HashMap
{
Map<String, Integer> map = new HashMap<String, Integer>();
public static void main(String[] args) {
SortingByValue ob = new SortingByValue();
ob.TheMap();
System.out.println("SORTING IN ASCENDING ORDER:");
ob.SortingByValue(true);
System.out.println("SORTING IN DESCENDING ORDER");
ob.SortingByValue(false);
}
void TheMap() // Creating a method to add elements into the HashMap
{
map.put("SUZUKI", 65090);
map.put("HERO", 24020);
map.put("DUCATI", 90000);
map.put("YAMAHA", 71478);
map.put("HARLEY", 86946);
map.put("KWASAKI", 99990);
System.out.println("VALUE BEFORE SORTING:: ");
printMap(map);
}
// sorting the elements ACCORDING to values
void SortingByValue(boolean order) {
// converting HashMap into a List
List<Entry<String, Integer>> list = new LinkedList<Entry<String, Integer>>(map.entrySet());
// sorting the list comprising items
Collections.sort(list, new Comparator<Entry<String, Integer>>() {
public int compare(Entry<String, Integer> obj1, Entry<String, Integer> obj2) {
if (order) {
// comparing the two object and returning an integer
return obj1.getValue().compareTo(obj2.getValue());
} else {
return obj2.getValue().compareTo(obj1.getValue());
}
}
});
// Displaying the sorted HashMap
Map<String, Integer> sortedhashMap = new LinkedHashMap<String, Integer>();
for (Entry<String, Integer> entry : list) {
sortedhashMap.put(entry.getKey(), entry.getValue());
}
printMap(sortedhashMap);
}
// Function for Displaying the elements
public void printMap(Map<String, Integer> map) {
System.out.println("BIKE\t\t PRICE ");
for (Entry<String, Integer> entry : map.entrySet()) {
System.out.println(entry.getKey() + "\t \t" + entry.getValue());
}
System.out.println("\n");
}
}