Ordina HashMap in Java
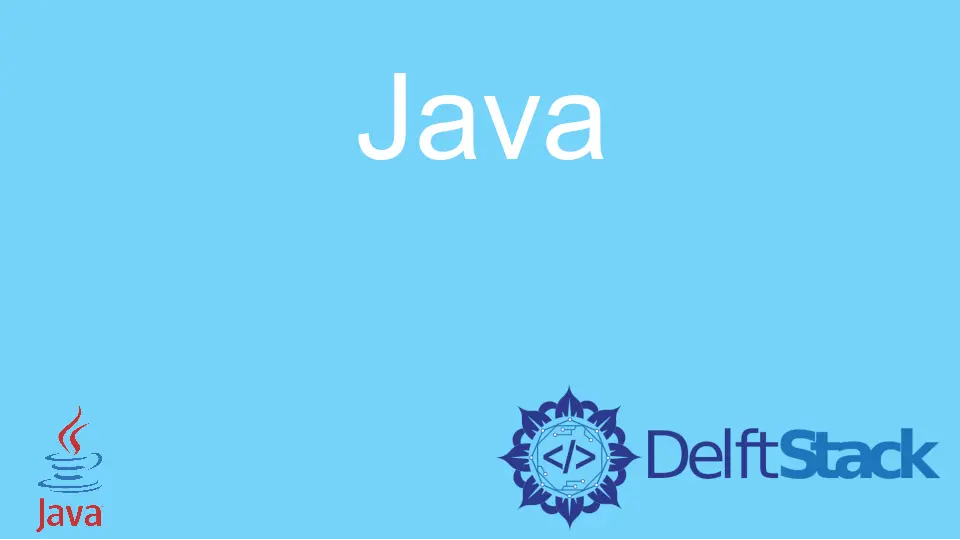
Le hashmap non sono fatte per l’ordinamento. Sono fatti per un rapido recupero. Quindi, un metodo molto semplice sarebbe prendere ogni elemento dalla Hashmap e inserirli in una struttura di dati che è migliore per l’ordinamento, come un mucchio o un set, e poi ordinarli lì.
Ma se la tua idea è quella di ordinare usando una Hashmap, discuteremo alcuni metodi in questo tutorial.
Se abbiamo bisogno di ordinare HashMap, lo facciamo esplicitamente secondo i criteri richiesti. Possiamo ordinare HashMaps per chiavi o per valore in Java.
Ordina una HashMap per chiavi in Java
Usando i tasti, possiamo ordinare una HashMap in due modi: una LinkedHashMap
o una TreeMap
.
Durante l’utilizzo dell’approccio LinkedHashMap
, è fondamentale ottenere un set di chiavi. Dopo aver ricevuto un tale set di chiavi, li traduciamo in un elenco. Questo elenco viene quindi ordinato di conseguenza e aggiunto a LinkedHashMap
nello stesso ordine. Non c’è duplicità delle chiavi nell’ordinamento di HashMap per chiavi.
Codice:
import static java.util.stream.Collectors.*;
import java.lang.*;
import java.util.*;
import java.util.stream.*;
import java.util.stream.Collectors;
public class Main {
// unsorted
static Map<String, Integer> lhmap = new HashMap<>();
public static void sort() {
HashMap<String, Integer> x = lhmap.entrySet()
.stream()
.sorted((i1, i2) -> i1.getKey().compareTo(i2.getKey()))
.collect(Collectors.toMap(Map.Entry::getKey,
Map.Entry::getValue, (z1, z2) -> z1, LinkedHashMap::new));
// Show Sorted HashMap
for (Map.Entry<String, Integer> start : x.entrySet()) {
System.out.println("Your Key " + start.getKey() + ", Your Value = " + start.getValue());
}
}
public static void main(String args[]) {
// insert value
lhmap.put("a", 1);
lhmap.put("aa", 3);
lhmap.put("ab", 5);
lhmap.put("ba", 7);
lhmap.put("bb", 8);
lhmap.put("aac", 23);
lhmap.put("bac", 8);
sort();
}
}
Produzione:
Your Key a, Your Value = 1
Your Key aa, Your Value = 3
Your Key aac, Your Value = 23
Your Key ab, Your Value = 5
Your Key ba, Your Value = 7
Your Key bac, Your Value = 8
Your Key bb, Your Value = 8
Possiamo anche usare una TreeMap
. Una delle implementazioni di SortedMap
è una TreeMap
che aiuta a mantenere le chiavi nell’ordine naturale o nell’ordine personalizzato specificato dal comparatore fornito durante la creazione di TreeMap
. Ciò implica che l’utente può elaborare le voci di HashMap in ordine ordinato. Tuttavia, l’utente non può passare una HashMap con mappature in un ordine specifico perché HashMap non garantisce un ordine.
Codice:
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.HashMap;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.TreeMap;
public class SortHashmap {
public static void main(String args[]) {
// Create a HashMap object ob
HashMap<Integer, String> ob = new HashMap<Integer, String>();
// addding keys and values
ob.put(23, "Vedant");
ob.put(7, "Aryan");
ob.put(17, "Tarun");
ob.put(9, "Farhan");
Iterator<Integer> it = ob.keySet().iterator();
System.out.println("Before Sorting");
while (it.hasNext()) {
int key = (int) it.next();
System.out.println("Roll number: " + key + " name: " + ob.get(key));
}
System.out.println("\n");
Map<Integer, String> map = new HashMap<Integer, String>();
System.out.println("After Sorting");
// using the TreeMap constructor in order to sort the HashMap
TreeMap<Integer, String> tm = new TreeMap<Integer, String>(ob);
Iterator itr = tm.keySet().iterator();
while (itr.hasNext()) {
int key = (int) itr.next();
System.out.println("Roll no: " + key + " name: " + ob.get(key));
}
}
}
Ordina una HashMap per valori in Python
Questo metodo mira a mantenere gli elementi della voce in un elenco, quindi ordiniamo questo elenco di elementi in base al loro valore. Questi valori e chiavi vengono quindi recuperati dall’elenco degli elementi e quindi inseriti di conseguenza in una nuova HashMap.
A causa di questo nuovo HashMap è ordinato interamente in base ai valori.
Per confrontare gli articoli in base ai loro valori, dobbiamo creare un comparator
. Durante l’utilizzo di questo approccio, dobbiamo tenere presente che possiamo memorizzare valori duplicati.
Vedi il codice qui sotto.
import java.util.Collections;
import java.util.Comparator;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
public class SortingByValue // implementing the HashMap
{
Map<String, Integer> map = new HashMap<String, Integer>();
public static void main(String[] args) {
SortingByValue ob = new SortingByValue();
ob.TheMap();
System.out.println("SORTING IN ASCENDING ORDER:");
ob.SortingByValue(true);
System.out.println("SORTING IN DESCENDING ORDER");
ob.SortingByValue(false);
}
void TheMap() // Creating a method to add elements into the HashMap
{
map.put("SUZUKI", 65090);
map.put("HERO", 24020);
map.put("DUCATI", 90000);
map.put("YAMAHA", 71478);
map.put("HARLEY", 86946);
map.put("KWASAKI", 99990);
System.out.println("VALUE BEFORE SORTING:: ");
printMap(map);
}
// sorting the elements ACCORDING to values
void SortingByValue(boolean order) {
// converting HashMap into a List
List<Entry<String, Integer>> list = new LinkedList<Entry<String, Integer>>(map.entrySet());
// sorting the list comprising items
Collections.sort(list, new Comparator<Entry<String, Integer>>() {
public int compare(Entry<String, Integer> obj1, Entry<String, Integer> obj2) {
if (order) {
// comparing the two object and returning an integer
return obj1.getValue().compareTo(obj2.getValue());
} else {
return obj2.getValue().compareTo(obj1.getValue());
}
}
});
// Displaying the sorted HashMap
Map<String, Integer> sortedhashMap = new LinkedHashMap<String, Integer>();
for (Entry<String, Integer> entry : list) {
sortedhashMap.put(entry.getKey(), entry.getValue());
}
printMap(sortedhashMap);
}
// Function for Displaying the elements
public void printMap(Map<String, Integer> map) {
System.out.println("BIKE\t\t PRICE ");
for (Entry<String, Integer> entry : map.entrySet()) {
System.out.println(entry.getKey() + "\t \t" + entry.getValue());
}
System.out.println("\n");
}
}