Java 中的 HashMap 排序
Aryan Tyagi
2023年10月12日
Java
Java HashMap
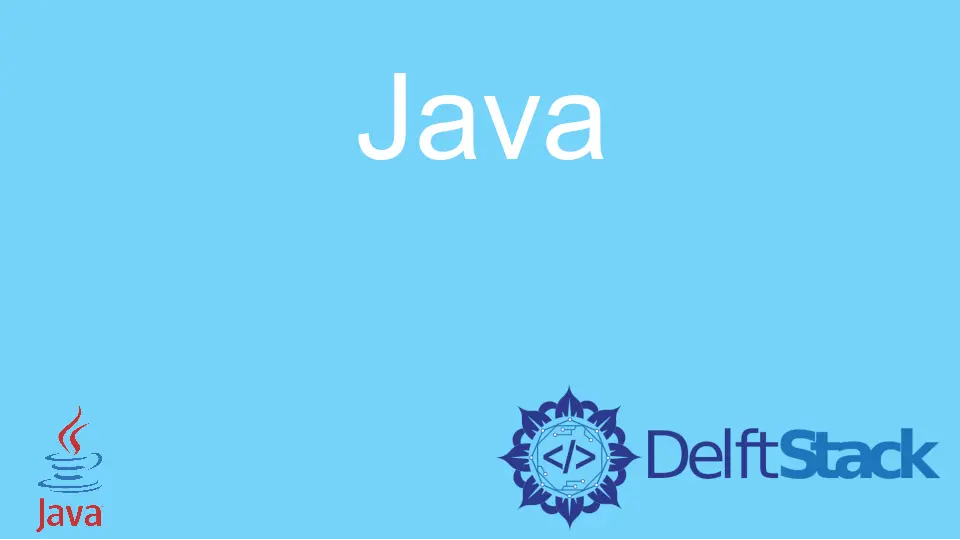
哈希图不是用于排序的。它们是为快速检索而设计的。因此,一个非常简单的方法是从 Hashmap 中取出每个元素并将它们放在一个更适合排序的数据结构中,如堆或集合,然后在那里对它们进行排序。
但是,如果你的想法是使用 Hashmap 进行排序,我们将在本教程中讨论一些方法。
如果我们需要对 HashMap 进行排序,我们会根据所需的标准明确地进行排序。我们可以在 Java 中按键或值对 HashMap 进行排序。
在 Java 中按键对 HashMap 进行排序
使用键,我们可以通过两种方式对 HashMap 进行排序:LinkedHashMap
或 TreeMap
。
在使用 LinkedHashMap
方法时,获取密钥集至关重要。收到这样的密钥集后,我们将它们翻译成一个列表。这个列表然后相应地排序并以相同的顺序添加到 LinkedHashMap
。按键对 HashMap 进行排序时没有键的重复性。
代码:
import static java.util.stream.Collectors.*;
import java.lang.*;
import java.util.*;
import java.util.stream.*;
import java.util.stream.Collectors;
public class Main {
// unsorted
static Map<String, Integer> lhmap = new HashMap<>();
public static void sort() {
HashMap<String, Integer> x = lhmap.entrySet()
.stream()
.sorted((i1, i2) -> i1.getKey().compareTo(i2.getKey()))
.collect(Collectors.toMap(Map.Entry::getKey,
Map.Entry::getValue, (z1, z2) -> z1, LinkedHashMap::new));
// Show Sorted HashMap
for (Map.Entry<String, Integer> start : x.entrySet()) {
System.out.println("Your Key " + start.getKey() + ", Your Value = " + start.getValue());
}
}
public static void main(String args[]) {
// insert value
lhmap.put("a", 1);
lhmap.put("aa", 3);
lhmap.put("ab", 5);
lhmap.put("ba", 7);
lhmap.put("bb", 8);
lhmap.put("aac", 23);
lhmap.put("bac", 8);
sort();
}
}
输出:
Your Key a, Your Value = 1
Your Key aa, Your Value = 3
Your Key aac, Your Value = 23
Your Key ab, Your Value = 5
Your Key ba, Your Value = 7
Your Key bac, Your Value = 8
Your Key bb, Your Value = 8
我们也可以使用 TreeMap
。SortedMap
的实现之一是 TreeMap
,它有助于将键保持在自然顺序或由创建 TreeMap
时提供的比较器指定的自定义顺序。这意味着用户可以按排序顺序处理 HashMap 的条目。但是,用户不能传递具有特定顺序映射的 HashMap,因为 HashMap 不保证顺序。
代码:
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.HashMap;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.TreeMap;
public class SortHashmap {
public static void main(String args[]) {
// Create a HashMap object ob
HashMap<Integer, String> ob = new HashMap<Integer, String>();
// addding keys and values
ob.put(23, "Vedant");
ob.put(7, "Aryan");
ob.put(17, "Tarun");
ob.put(9, "Farhan");
Iterator<Integer> it = ob.keySet().iterator();
System.out.println("Before Sorting");
while (it.hasNext()) {
int key = (int) it.next();
System.out.println("Roll number: " + key + " name: " + ob.get(key));
}
System.out.println("\n");
Map<Integer, String> map = new HashMap<Integer, String>();
System.out.println("After Sorting");
// using the TreeMap constructor in order to sort the HashMap
TreeMap<Integer, String> tm = new TreeMap<Integer, String>(ob);
Iterator itr = tm.keySet().iterator();
while (itr.hasNext()) {
int key = (int) itr.next();
System.out.println("Roll no: " + key + " name: " + ob.get(key));
}
}
}
在 Python 中按值对 HashMap 进行排序
此方法旨在将条目项保留在列表中,然后我们根据它们的值对这个项目列表进行排序。然后从项目的列表中检索这些值和键,然后相应地放置在新的 HashMap 中。
由于这个新的 HashMap 完全基于值排序。
为了根据它们的值比较项目,我们必须创建一个 comparator
。在使用这种方法时,我们必须记住我们可以存储重复的值。
请参考下面的代码。
import java.util.Collections;
import java.util.Comparator;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
public class SortingByValue // implementing the HashMap
{
Map<String, Integer> map = new HashMap<String, Integer>();
public static void main(String[] args) {
SortingByValue ob = new SortingByValue();
ob.TheMap();
System.out.println("SORTING IN ASCENDING ORDER:");
ob.SortingByValue(true);
System.out.println("SORTING IN DESCENDING ORDER");
ob.SortingByValue(false);
}
void TheMap() // Creating a method to add elements into the HashMap
{
map.put("SUZUKI", 65090);
map.put("HERO", 24020);
map.put("DUCATI", 90000);
map.put("YAMAHA", 71478);
map.put("HARLEY", 86946);
map.put("KWASAKI", 99990);
System.out.println("VALUE BEFORE SORTING:: ");
printMap(map);
}
// sorting the elements ACCORDING to values
void SortingByValue(boolean order) {
// converting HashMap into a List
List<Entry<String, Integer>> list = new LinkedList<Entry<String, Integer>>(map.entrySet());
// sorting the list comprising items
Collections.sort(list, new Comparator<Entry<String, Integer>>() {
public int compare(Entry<String, Integer> obj1, Entry<String, Integer> obj2) {
if (order) {
// comparing the two object and returning an integer
return obj1.getValue().compareTo(obj2.getValue());
} else {
return obj2.getValue().compareTo(obj1.getValue());
}
}
});
// Displaying the sorted HashMap
Map<String, Integer> sortedhashMap = new LinkedHashMap<String, Integer>();
for (Entry<String, Integer> entry : list) {
sortedhashMap.put(entry.getKey(), entry.getValue());
}
printMap(sortedhashMap);
}
// Function for Displaying the elements
public void printMap(Map<String, Integer> map) {
System.out.println("BIKE\t\t PRICE ");
for (Entry<String, Integer> entry : map.entrySet()) {
System.out.println(entry.getKey() + "\t \t" + entry.getValue());
}
System.out.println("\n");
}
}
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe