How to Convert ArrayList to Int Array in Java
-
Importance of Converting
ArrayList
to Int Array in Java -
Methods on How to Convert
ArrayList
to Int Array -
Best Practices in Converting
ArrayList
to Int Array in Java - Conclusion
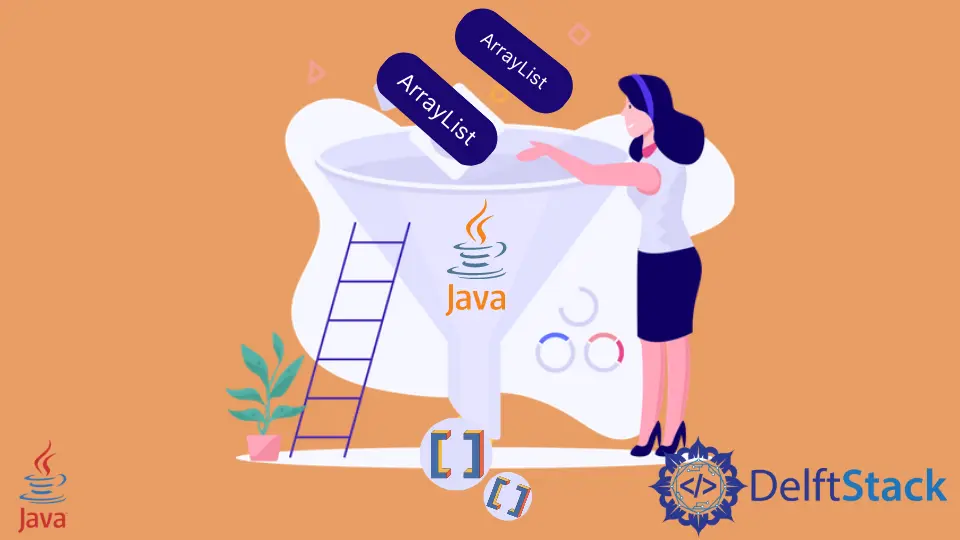
Explore the significance, methodologies, and best practices of converting ArrayList
to int array in Java for streamlined data handling.
Importance of Converting ArrayList
to Int Array in Java
Converting an ArrayList
to an int array in Java is crucial for seamless integration with methods or systems that require primitive integer arrays. It ensures compatibility between different parts of a program, facilitating efficient data processing and enhancing the overall versatility of the code.
This conversion is particularly important when dealing with legacy code, external libraries, or APIs that expect or operate with primitive int arrays rather than ArrayLists
. It simplifies data exchange and enables smooth collaboration between diverse components within a Java application.
Methods on How to Convert ArrayList
to Int Array
Using the toArray()
Method
The toArray()
method in Java simplifies converting ArrayList
to an int array, offering a concise and efficient solution. It eliminates complexity found in other methods, providing a direct and readable approach.
This method ensures a seamless transition between ArrayList
and array types, contributing to code clarity and ease of implementation.
Let’s delve into a comprehensive example where we convert an ArrayList
of integers into a primitive int array using the toArray()
method:
import java.util.ArrayList;
public class ArrayListToIntArrayWithToArrayMethod {
public static void main(String[] args) {
// Create an ArrayList of integers
ArrayList<Integer> arrayList = new ArrayList<>();
arrayList.add(1);
arrayList.add(2);
arrayList.add(3);
arrayList.add(4);
Integer[] integerArray = new Integer[arrayList.size()];
Integer[] intArray = arrayList.toArray(integerArray);
// Display the original ArrayList
System.out.println("Original ArrayList: " + arrayList);
// Display the resulting int array
System.out.print("Converted int Array: [");
for (int i = 0; i < intArray.length; i++) {
System.out.print(intArray[i]);
if (i < intArray.length - 1) {
System.out.print(", ");
}
}
System.out.println("]");
}
}
We start by importing the ArrayList
class for dynamic array functionality. Creating an ArrayList
named arrayList
, we add four integers to the list.
Next, we create an array of Integer
objects, integerArray
, with the same size as the ArrayList
. Using the toArray
method, we convert the ArrayList
to an array, utilizing integerArray
as an argument.
The original contents of the ArrayList
are displayed. Finally, we showcase the resulting intArray
using a for
loop for clarity, ensuring proper formatting with commas separating array elements.
Upon executing the code, the output will be:
The code effectively demonstrates the conversion of an ArrayList
of integers to an array of Integer
objects using the toArray
method. The resulting array (intArray
) maintains the order of elements from the original ArrayList
.
This approach is convenient and allows for precise control over the type of the resulting array. The output showcases the successful transformation, highlighting the clarity and efficiency of this method for such conversions in Java programming.
Using the Java 8 Stream API Method
Java 8 Stream API simplifies converting ArrayList
to an int array by providing a concise and expressive approach. Compared to traditional methods or loops, Stream API enhances readability and reduces boilerplate code.
It promotes a functional programming style, enabling developers to achieve the conversion with fewer lines of code, making the process more elegant and efficient.
Let’s consider a practical example where we have an ArrayList
of integers and desire a seamless transformation into a primitive int array using the Stream API:
import java.util.ArrayList;
import java.util.List;
public class ArrayListToIntArrayWithStream {
public static void main(String[] args) {
// Create an ArrayList of integers
List<Integer> arrayList = new ArrayList<>();
arrayList.add(1);
arrayList.add(2);
arrayList.add(3);
arrayList.add(4);
// Convert ArrayList to int array using Java 8 Stream API
int[] intArray = arrayList.stream().mapToInt(Integer::intValue).toArray();
// Display the original ArrayList
System.out.println("Original ArrayList: " + arrayList);
// Display the resulting int array
System.out.print("Converted int Array: [");
for (int i = 0; i < intArray.length; i++) {
System.out.print(intArray[i]);
if (i < intArray.length - 1) {
System.out.print(", ");
}
}
System.out.println("]");
}
}
Now, let’s explore the code, unraveling the process of converting an ArrayList
to an int array using the Java 8 Stream API. To begin, we create an ArrayList
containing integers—a common starting point for data manipulation.
Leveraging the Stream API’s stream()
method, we transform the ArrayList
into a stream of elements. The mapToInt(Integer::intValue)
function facilitates the mapping of each element to its primitive int
value.
Ultimately, the toArray()
method concludes the transformation, producing an array of primitive ints. To provide context, the original ArrayList
is displayed.
Finally, the resulting int array is presented, employing a conventional loop for clear and formatted output.
Upon executing the code, the output will be:
The Java 8 Stream API brings an expressive and efficient approach to transforming collections. In this context, converting an ArrayList
of integers to a primitive int array becomes a simple task.
Leveraging the power of Stream API not only simplifies the code but also embraces the functional programming paradigm, making it a valuable addition to the Java developer’s toolkit. As we witness the resulting array seamlessly derived from the ArrayList
, it exemplifies the elegance and effectiveness of the Java 8 Stream API in modern Java programming.
Using the for
Loop Method
Using a for
loop for converting ArrayList
to an int array in Java offers simplicity and direct control, making the code easy to understand. It provides a straightforward approach without the additional complexity introduced by other methods, ensuring readability and efficiency in scenarios where a concise and traditional solution is preferred.
Let’s dive into a practical example where we convert an ArrayList
of integers into a primitive int array using a traditional for
loop:
import java.util.ArrayList;
public class ArrayListToIntArrayWithForLoop {
public static void main(String[] args) {
// Create an ArrayList of integers
ArrayList<Integer> arrayList = new ArrayList<>();
arrayList.add(1);
arrayList.add(2);
arrayList.add(3);
arrayList.add(4);
// Convert ArrayList to int array using a for loop
int[] intArray = new int[arrayList.size()];
for (int i = 0; i < arrayList.size(); i++) {
intArray[i] = arrayList.get(i);
}
// Display the original ArrayList
System.out.println("Original ArrayList: " + arrayList);
// Display the resulting int array
System.out.print("Converted int Array: [");
for (int i = 0; i < intArray.length; i++) {
System.out.print(intArray[i]);
if (i < intArray.length - 1) {
System.out.print(", ");
}
}
System.out.println("]");
}
}
Now, let’s explore the simplicity and effectiveness of converting an ArrayList
to a primitive int array using a for
loop. Begin by creating an ArrayList
of integers, a commonly used container in Java.
For the conversion, initialize an empty int array with the same size as the ArrayList
. Utilize a for
loop to iterate through each element, fetching and assigning values to the corresponding index in the int array.
This straightforward approach ensures clear and readable code. Display the original ArrayList
to provide a reference, and showcase the resulting int array with a for
loop for proper formatting, ensuring clarity in array representation.
Upon executing the code, the output will be:
The for
loop, a fundamental construct in Java, provides a clear and readable solution. As we witness the resulting array derived from the ArrayList
, it exemplifies the elegance and power inherent in the straightforward for
loop method, demonstrating that sometimes simplicity is the key to an elegant solution.
Best Practices in Converting ArrayList
to Int Array in Java
Use of the toArray
Method
Leverage the toArray()
method provided by Java for a straightforward conversion from ArrayList
to an int array. This built-in method simplifies the process and ensures code clarity.
Specify Array Type
When using toArray()
, explicitly specify the array type, ensuring the resulting array matches the desired primitive type (e.g., Integer[]
for ArrayList<Integer>
).
Looping for Customization
If customization is required, opt for a traditional for
loop. This approach provides more control, enabling specific handling or modifications during the conversion process.
Consider Stream API for Conciseness
In scenarios where brevity is crucial, explore the Java 8 Stream API. It offers a concise and expressive way to convert ArrayList
to an int array, enhancing code readability.
Conclusion
Efficiently converting ArrayList
to an int array in Java is crucial for seamless data integration. The toArray()
method simplifies the process, offering a built-in solution.
Explicitly specifying array types ensures compatibility. Utilizing traditional for
loops provides customization options.
For concise code, the Java 8 Stream API offers brevity. Adopting these methods adheres to best practices, promoting code clarity and versatility in handling diverse data structures.
Related Article - Java Array
- How to Concatenate Two Arrays in Java
- How to Convert Byte Array in Hex String in Java
- How to Remove Duplicates From Array in Java
- How to Count Repeated Elements in an Array in Java
- Natural Ordering in Java
- How to Slice an Array in Java