How to Convert String to Hex in C#
- Why Convert a String to Hexadecimal
- Convert String to Hex in C# Using a Custom Function
- Convert String to Hex in C# Using BitConverter
- Convert String to Hex in C# Using Hexadecimal Encoding Function (Newer .NET Versions)
- Convert String to Hex in C# Using LINQ
- Convert String to Hex in C# Using a Loop
- Conclusion
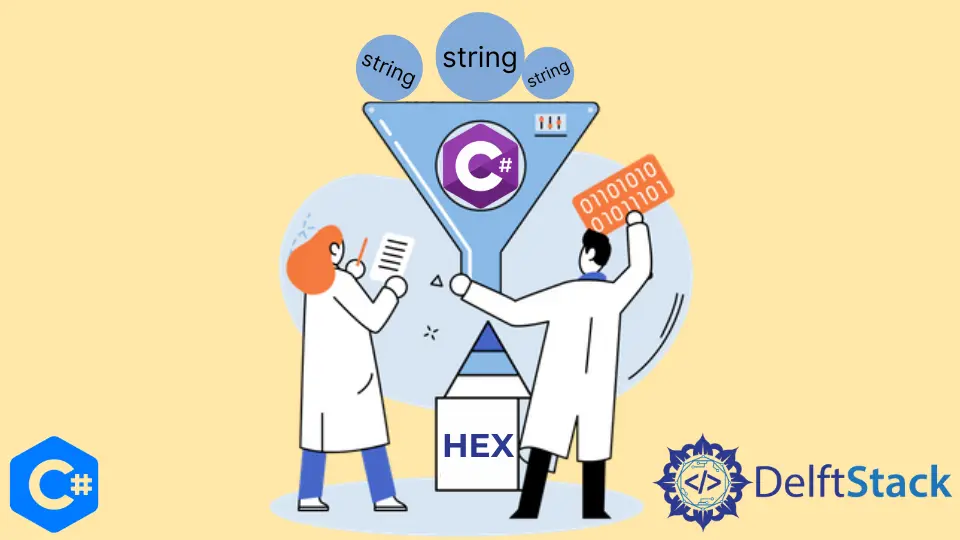
Converting a string to hexadecimal format is a common operation in programming, often required for various applications like encoding data, generating checksums, or working with binary data. In C#, this process can be achieved through multiple methods, each with its own advantages and use cases.
In this article, we will explore the various techniques to convert a string to hexadecimal format in C#.
Why Convert a String to Hexadecimal
Before we delve into the techniques, it’s important to understand why you might need to convert a string to a hexadecimal format. Hexadecimal, also known as hex, is a base-16 numeral system.
It is widely used in computing because it provides a more compact representation of binary data and is easier for humans to read and work with.
Here are some common use cases for converting strings to hexadecimal in C#:
-
Encoding Binary Data: Hexadecimal is often used to encode binary data, making it more human-readable. For example, representing a binary file as a hexadecimal string is useful when storing or transmitting data.
-
Checksums: Hexadecimal representations are commonly used in computing to calculate checksums, such as CRC (Cyclic Redundancy Check) or MD5. Hexadecimal checksums make it easy to verify data integrity.
-
Debugging and Logging: Hexadecimal representations are helpful when debugging and logging. They provide a concise way to display byte-level information.
-
Storing Non-Text Data: When dealing with non-text data like images or audio files, you might need to convert it to a hexadecimal string for storage or transmission.
Now that we understand the importance of converting strings to hexadecimal, let’s explore various methods to achieve this in C#.
Convert String to Hex in C# Using a Custom Function
One of the simplest ways to convert a string to hexadecimal in C# is to create a custom function. Here’s a sample implementation:
using System;
using System.Text;
class Program
{
public static string StringToHex(string input)
{
char[] chars = input.ToCharArray();
StringBuilder hex = new StringBuilder(chars.Length * 2);
foreach (char c in chars)
{
hex.AppendFormat("{0:X2}", (int)c);
}
return hex.ToString();
}
static void Main()
{
string originalString = "Hello, World!";
string hexString = StringToHex(originalString);
Console.WriteLine("Original String: " + originalString);
Console.WriteLine("Hexadecimal String: " + hexString);
}
}
Output:
Original String: Hello, World!
Hexadecimal String: 48656C6C6F2C20576F726C6421
In this example, we define a StringToHex
function that takes an input string and converts it into an array of characters. It then iterates through each character, converting it to its hexadecimal representation.
The "X2"
format specifier ensures that each hexadecimal digit is represented by two characters, providing a consistent and properly formatted hexadecimal string. Finally, the function returns the hexadecimal string.
This custom function is straightforward and works well for most scenarios. However, there are other methods to consider.
Convert String to Hex in C# Using BitConverter
The BitConverter
class is part of the System
namespace in C#, and it provides methods for converting data between various binary representations. It offers a straightforward approach to converting data types, including integers, floating-point numbers, and strings, to byte arrays and vice versa.
This class is widely employed in scenarios where you need to manipulate binary data efficiently.
Here’s an example:
using System;
using System.Text;
class Program
{
static void Main()
{
string originalString = "Hello, World!";
byte[] bytes = Encoding.UTF8.GetBytes(originalString);
string hexString = BitConverter.ToString(bytes).Replace("-", "");
Console.WriteLine("Original String: " + originalString);
Console.WriteLine("Hexadecimal String: " + hexString);
}
}
Output:
Original String: Hello, World!
Hexadecimal String: 48656C6C6F2C20576F726C6421
In this code snippet, we start by defining the original string, in this case, Hello, World!
.
Next, we use the Encoding.UTF8.GetBytes
method to convert the string into a byte array, encoding it in UTF-8 format. This step is essential, as it ensures that the string is correctly represented in a format that the BitConverter
class can process.
The heart of the conversion happens when we use the BitConverter.ToString
method, which transforms the byte array into a hexadecimal string. By default, the BitConverter.ToString
method includes hyphens between the hexadecimal pairs.
To obtain a clean, hyphen-free hexadecimal representation, we use the Replace("-", "")
method to remove the hyphens from the resulting string.
This approach is efficient and works well for most use cases, especially when you need to work with binary data.
Convert String to Hex in C# Using Hexadecimal Encoding Function (Newer .NET Versions)
Newer .NET versions provide an enhanced and more intuitive way to perform a string-to-hexadecimal conversion by offering a Hex
encoding function. This function is a part of the System.Text
namespace and simplifies the process of encoding a string as hexadecimal values.
While the BitConverter
class is a robust choice for this task, the Hex
encoding function streamlines the process even further, making it particularly appealing for developers working with the latest .NET framework versions.
Take a look at the example below:
using System;
using System.Text;
class Program
{
static void Main()
{
string originalString = "Hello, World!";
byte[] bytes = Encoding.Default.GetBytes(originalString);
string hexString = BitConverter.ToString(bytes).Replace("-", "");
Console.WriteLine("Original String: " + originalString);
Console.WriteLine("Hexadecimal String: " + hexString);
}
}
Output:
Original String: Hello, World!
Hexadecimal String: 48656C6C6F2C20576F726C6421
In this code example, we start by specifying the original string, which is Hello, World!
in this case.
We then utilize the Encoding.Default.GetBytes
method to convert the string into a byte array using the default character encoding. This step ensures that the string is appropriately represented in a format that the BitConverter
class can process.
Next, we employ the familiar BitConverter.ToString
method to transform the byte array into a hexadecimal string.
The resulting hexadecimal string will contain hyphens by default between each pair of hexadecimal characters. To obtain a clean, hyphen-free representation, we use the Replace("-", "")
method to eliminate the hyphens from the final string.
This approach is similar to Method 2 but takes advantage of newer features in the .NET framework.
Convert String to Hex in C# Using LINQ
For developers who prefer a more concise and functional approach, LINQ (Language-Integrated Query) offers an elegant solution to convert a string to its hexadecimal representation in C#. LINQ is a powerful feature of the language that enables developers to write expressive and readable code.
Below is an example:
using System;
using System.Linq;
class Program
{
static void Main()
{
string originalString = "Hello, World!";
string hexString = string.Concat(originalString.Select(c => ((int)c).ToString("X2")));
Console.WriteLine("Original String: " + originalString);
Console.println("Hexadecimal String: " + hexString);
}
}
Output:
Original String: Hello, World!
Hexadecimal String: 48656C6C6F2C20576F726C6421
In this code snippet, we begin by defining the original string, which is Hello, World!
in this case. Instead of converting the string to a byte array, we use LINQ to iterate through each character of the original string.
The heart of the LINQ-based conversion lies in the line:
string hexString = string.Concat(originalString.Select(c => ((int)c).ToString("X2")));
Here’s what this line does:
-
originalString.Select(c => ((int)c).ToString("X2"))
uses theSelect
method to transform each character in theoriginalString
into its hexadecimal representation. It does so by first casting the character to its corresponding Unicode code point integer representation using((int)c)
.The
ToString("X2")
method call then formats this integer as a hexadecimal string with two digits, ensuring each byte is represented as two hexadecimal characters. -
string.Concat
is used to concatenate the resulting hexadecimal representations into a single string. This results in the final hexadecimal string.
Using LINQ makes the code shorter and more readable, but it may not be the most efficient choice for very long strings or high-performance scenarios.
Convert String to Hex in C# Using a Loop
For those who prefer a more traditional, imperative approach, converting a string to its hexadecimal representation in C# can be achieved through a loop-based method. This technique can be especially helpful for educational purposes, as it provides a clear understanding of the conversion process.
To convert a string to a hexadecimal representation in C# using a loop, see the example below:
using System;
class Program
{
static void Main()
{
string originalString = "Hello, World!";
string hexString = "";
foreach (char c in originalString)
{
hexString += String.Format("{0:X2}", (int)c);
}
Console.WriteLine("Original String: " + originalString);
Console.WriteLine("Hexadecimal String: " + hexString);
}
}
Output:
Original String: Hello, World!
Hexadecimal String: 48656C6C6F2C20576F726C6421
Here, we start by defining the original string, which is Hello, World!
in this example. To hold the resulting hexadecimal representation, we initialize an empty string called hexString
.
The core of the loop-based conversion is the foreach
loop:
foreach (char c in originalString)
{
hexString += String.Format("{0:X2}", (int)c);
}
In this code block, foreach (char c in originalString)
iterates through each character (c
) in the originalString
.
Next, String.Format("{0:X2}", (int)c)
converts the character to its corresponding Unicode code point integer and then formats it as a hexadecimal string with two digits using the X2
format specifier. This formatted hexadecimal representation is then appended to the hexString
variable.
The final result is a hexadecimal string that contains the hexadecimal representation of each character in the original string.
This method is straightforward to understand, making it a good choice for educational purposes.
Conclusion
Converting a string to hexadecimal format in C# is a common task with various methods available to achieve it. The choice of method depends on your specific requirements, performance considerations, and coding style preferences.
In this guide, we explored five different methods:
-
Using a Custom Function: This method involves creating a custom function to convert a string to hexadecimal. It’s simple and suitable for most scenarios.
-
Using
BitConverter
: TheBitConverter
class provides a convenient way to convert strings to hexadecimal, especially when working with binary data. -
Using Hexadecimal Encoding Function (Newer .NET Versions): In newer versions of .NET, you can take advantage of a hexadecimal encoding function to achieve the conversion.
-
Using LINQ: This method is concise and functional, suitable for shorter code and situations where performance is not critical.
-
Using a Loop: A traditional loop-based approach that is easy to understand and suitable for educational purposes.
Choose the method that best fits your specific requirements and the version of .NET you are using. Regardless of your choice, you now have a solid understanding of how to convert strings to hexadecimal in C#.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Csharp String
- How to Convert String to Enum in C#
- How to Convert Int to String in C#
- How to Use Strings in Switch Statement in C#
- How to Convert a String to Boolean in C#
- How to Convert a String to Float in C#
- How to Convert a String to a Byte Array in C#