在 C# 中將字串轉換為十六進位制
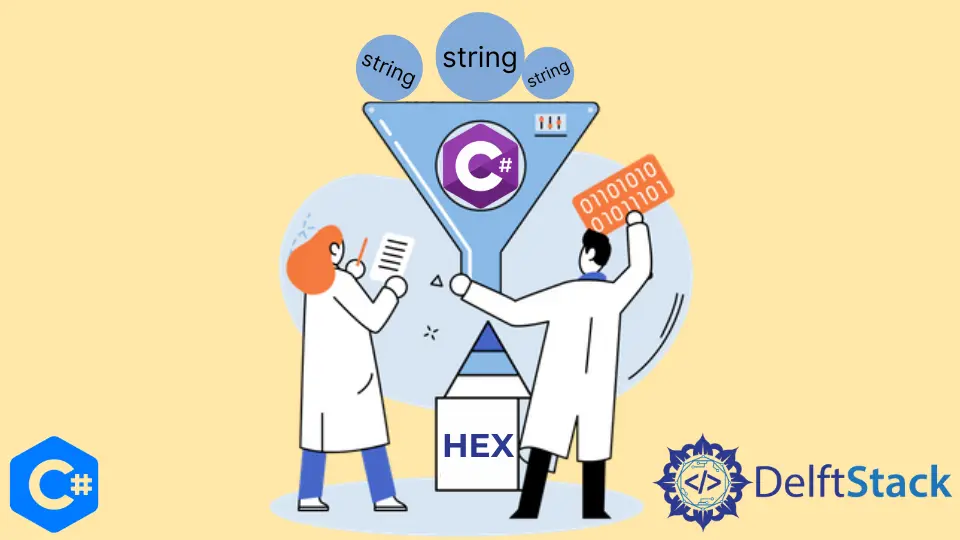
本教程將討論在 C# 中將字串轉換為十六進位制的方法。
在 C# 中使用 BitConverter.ToString()
方法將字串轉換為十六進位制
如果我們有一個包含以 10 為底的數值的字串,並且需要將其轉換為包含以 16 為底的數值的字串,我們可以使用 BitConverter.ToString()
方法。C# 中的 BitConverter.ToString(x)
方法將位元組陣列 x
中的每個元素轉換為十六進位制值。要使用 BitConverter.ToString()
方法,我們必須使用 Encoding.Default.GetBytes()
方法將字串變數轉換為位元組陣列。此方法將字串變數轉換為 C# 中的位元組陣列。BitConverter.ToString()
方法返回一個十六進位制字串,其中每個值都用 -
分隔。我們可以使用 String.Replace()
方法刪除 -
分隔符。
using System;
using System.Linq;
using System.Text;
namespace string_to_hex {
class Program {
static void Main(string[] args) {
string decString = "0123456789";
byte[] bytes = Encoding.Default.GetBytes(decString);
string hexString = BitConverter.ToString(bytes);
hexString = hexString.Replace("-", "");
Console.WriteLine(hexString);
}
}
}
輸出:
30313233343536373839
在上面的程式碼中,我們使用 C# 中的 BitConverter.ToString()
方法將具有十進位制值的字串 decString
轉換為具有十六進位制值的字串 hexString
。
在 C# 中使用 String.Format()
方法將字串轉換為十六進位制
String.Format()
方法根據 C# 中給定的格式說明符設定字串格式。{0:X2}
格式說明符指定十六進位制格式。我們可以在 String.Format()
方法內使用 {0:X2}
格式說明符,以將具有十進位制值的字串格式化為具有十六進位制值的字串。我們可以使用 LINQ 輕鬆地將十進位制字串的每個字元格式化為十六進位制格式。
using System;
using System.Linq;
using System.Text;
namespace string_to_hex {
class Program {
static void Main(string[] args) {
string decString = "0123456789";
var hexString =
string.Join("", decString.Select(c => String.Format("{0:X2}", Convert.ToInt32(c))));
Console.WriteLine(hexString);
}
}
}
輸出:
30313233343536373839
在上面的程式碼中,我們使用 String.Format()
方法和 C# 中的 LINQ 將具有十進位制值的字串變數 decString
轉換為具有十六進位制值的字串變數 hexString
。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn相關文章 - Csharp String
- C# 將字串轉換為列舉型別
- C# 中將整形 Int 轉換為字串 String
- 在 C# 中的 Switch 語句中使用字串
- 如何在 C# 中把一個字串轉換為布林值
- 如何在 C# 中把一個字串轉換為浮點數
- 如何在 C# 中將字串轉換為位元組陣列