How to Convert Int to Hex in C#
-
Convert Int to Hex Using the
ToString()
Method in C# -
Convert Int to Hex Using the
String.Format
Method in C# - Convert Int to Hex Using String Interpolation in C#
-
Convert Hex to Int With the
Convert.ToInt32()
Function in C# - Conclusion
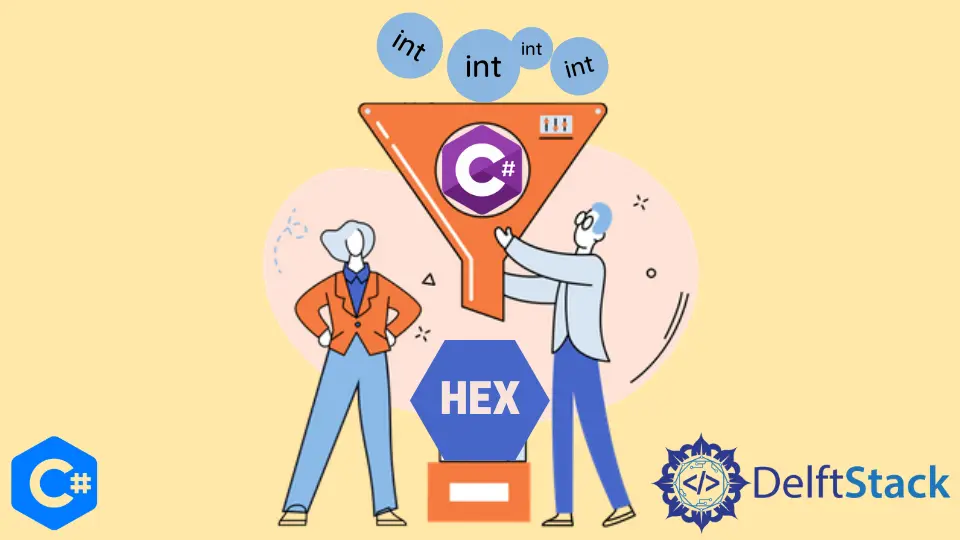
In C# programming, converting integers to their hexadecimal representation is a task that frequently arises, particularly when dealing with low-level programming, memory addresses, or binary data. While there are multiple methods to accomplish this conversion, understanding and utilizing a variety of techniques can be a valuable asset for any C# developer.
In this article, we have explored how to convert integers to their hexadecimal equivalent using different methods. We’ve covered four primary methods to achieve this: ToString()
, String.Format
, string interpolation, and the Convert.ToInt32()
function.
By understanding and implementing these methods, you can choose the one that best fits your specific needs and coding style. Let’s delve into each method and explore how it works.
Convert Int to Hex Using the ToString()
Method in C#
The ToString()
method in C# is a method available on most types in the .NET
framework, and it allows you to convert an instance of a class or a value of a basic data type to its string representation. Its basic syntax is as follows:
public string ToString();
However, the power of ToString()
lies in its overloads that allow you to specify various formatting options for different data types. For converting an integer to a hexadecimal representation, you can use the following syntax:
public string ToString(string format);
Where:
format
is a string that specifies the format to use when converting the value to a string.
For converting integers to hexadecimal, you can use a format specifier like X
or X2
. The X
format specifier is used for standard hexadecimal representation, and X2
specifies a two-character wide hexadecimal representation with leading zeros.
You can customize the X
format specifier with different widths as needed.
Here’s a basic example:
using System;
class Program {
static void Main() {
int number = 255;
string hex = number.ToString("X");
Console.WriteLine("Decimal: " + number);
Console.WriteLine("Hexadecimal: " + hex);
}
}
When you run this program, it will output:
Decimal: 255
Hexadecimal: FF
In the above code, we initialize an integer number
with the value 255
. We then call ToString("X")
on the integer, which instructs it to format as a hexadecimal string.
You can customize the hexadecimal formatting using the format string passed to ToString()
. For example, you can control the width and add leading zeros to the hexadecimal representation.
Here’s an example:
using System;
class Program {
static void Main() {
int number = 15;
string hex = number.ToString("X2");
Console.WriteLine("Decimal: " + number);
Console.WriteLine("Hexadecimal: " + hex);
}
}
When you run this program, it will output:
Decimal: 15
Hexadecimal: 0F
In this case, the X2
format specifier tells C# to format the integer as a hexadecimal string with a width of 2
characters. Since 15
is smaller than 0x10
(16
in decimal), it adds a leading zero to make the result 0F
.
Handling Negative Integers
When converting negative integers to hexadecimal, you might encounter some surprises.
By default, C# represents negative numbers in two’s complement form. To convert a negative integer to its hexadecimal representation, you’ll need to convert it to its unsigned representation first.
Here’s an example:
using System;
class Program {
static void Main() {
int negativeNumber = -42;
uint unsignedNumber = (uint)negativeNumber;
string hex = unsignedNumber.ToString("X");
Console.WriteLine("Original Negative Number: " + negativeNumber);
Console.WriteLine("Unsigned Number: " + unsignedNumber);
Console.WriteLine("Hexadecimal: " + hex);
}
}
When you run this program, it will output:
Original Negative Number: -42
Unsigned Number: 4294967254
Hexadecimal: FFFFFFD6
This code snippet converts the original negative integer negativeNumber
(-42
) to an unsigned integer (unsignedNumber
) by casting it to uint
.
It then converts the unsigned integer to its hexadecimal representation, which is FFFFFFD6
, and displays the original negative number, the unsigned number, and the hexadecimal representation on the console.
Handling Large Integers
For large integers, it’s essential to ensure that the hexadecimal representation includes all necessary digits. You can use the format specifier X
with a width greater than the actual number of hexadecimal digits to achieve this.
Here’s an example:
using System;
class Program {
static void Main() {
int largeNumber = 50000;
string hex = largeNumber.ToString("X8");
Console.WriteLine("Decimal: " + largeNumber);
Console.WriteLine("Hexadecimal: " + hex);
}
}
When you run this program, it will output:
Decimal: 50000
Hexadecimal: 0000C350
In this case, the code snippet converts the integer largeNumber
with the value 50000
to its hexadecimal representation with a width of 8
characters, which includes leading zeros. The hexadecimal representation is 0000C350
, and both the decimal and hexadecimal representations are displayed on the console.
In this case, we set the format specifier to X8
, which tells C# to format the integer as a hexadecimal string with a width of 8
characters. It ensures that the resulting string includes all eight hexadecimal digits, even if some of them are zeros.
Convert Int to Hex Using the String.Format
Method in C#
While you can use the ToString()
method, as discussed in a previous section, you can also achieve the same result using the String.Format
method, which provides more formatting options.
The String.Format
method in C# is a powerful tool for creating formatted strings. It allows you to combine text with placeholders, where each placeholder is defined using curly braces {}
.
Inside the curly braces, you specify the data and its formatting. To convert an integer to its hexadecimal representation, you can use the X
format specifier within the String.Format
method.
The basic syntax of the String.Format
method is as follows:
string formattedString = string.Format(format, arg0, arg1, ...);
Where:
format
is a composite format string that specifies the format of the resulting string. It contains placeholders enclosed in curly braces{}
.arg0, arg1, ...
are the arguments to be formatted and inserted into the placeholders specified in theformat
string.
The placeholders in the format
string are represented using curly braces {}
with an optional index that indicates which argument should be inserted into that placeholder. For example, {0}
represents the first argument, {1}
represents the second argument, and so on.
You can also include format specifiers within the placeholders to control how the arguments are formatted. For converting integers to hexadecimal, you would use the X
format specifier, like {0:X}
, to format the first argument as a hexadecimal string.
Here’s a simple example:
using System;
class Program {
static void Main() {
int number = 255;
string hex = string.Format("{0:X}", number);
Console.WriteLine("Decimal: " + number);
Console.WriteLine("Hexadecimal: " + hex);
}
}
When you run this program, it will output:
Decimal: 255
Hexadecimal: FF
Here, {0:X}
within the format string specifies that the first argument (number
) should be formatted as a hexadecimal string. The resulting hex
variable will contain the value FF
in this case.
You can also customize the formatting further by specifying additional format options within the curly braces. For example, you can control the width of the hexadecimal representation and add leading zeros:
using System;
class Program {
static void Main() {
int number = 15;
string hex = string.Format("{0:X2}", number);
Console.WriteLine("Decimal: " + number);
Console.WriteLine("Hexadecimal: " + hex);
}
}
When you run this program, it will output:
Decimal: 15
Hexadecimal: 0F
In this code, {0:X2}
tells String.Format
to format the number as a hexadecimal string with a width of 2
characters. It ensures that the resulting string includes a leading zero, resulting in 0F
.
Handling Negative Integers
When converting negative integers to hexadecimal using String.Format
, you might encounter the same two’s complement form as with ToString()
. To handle negative integers correctly, you should convert them to their unsigned representation first:
using System;
class Program {
static void Main() {
int negativeNumber = -42;
uint unsignedNumber = (uint)negativeNumber;
string hex = string.Format("{0:X}", unsignedNumber);
Console.WriteLine("Original Negative Number: " + negativeNumber);
Console.WriteLine("Unsigned Number: " + unsignedNumber);
Console.WriteLine("Hexadecimal: " + hex);
}
}
When you run this program, it will output:
Original Negative Number: -42
Unsigned Number: 4294967254
Hexadecimal: FFFFFFD6
In this code, we cast the negative integer to an unsigned integer using (uint)
and then use String.Format
to create the hexadecimal representation. The resulting hex
variable contains FFFFFFD6
for the value -42
.
Handling Large Integers
For large integers, you can use the String.Format
method to ensure that the hexadecimal representation includes all necessary digits. By specifying the width, you can create a consistent output:
using System;
class Program {
static void Main() {
int largeNumber = 50000;
string hex = string.Format("{0:X8}", largeNumber);
Console.WriteLine("Decimal: " + largeNumber);
Console.WriteLine("Hexadecimal: " + hex);
}
}
When you run this program, it will output:
Decimal: 50000
Hexadecimal: 0000C350
Here, the code snippet converts the integer largeNumber
with the value 50000
to its hexadecimal representation with a width of 8
characters, ensuring that leading zeros are included. The decimal and hexadecimal representations are displayed on the console.
Convert Int to Hex Using String Interpolation in C#
String interpolation is a convenient and modern feature in C# that allows you to embed expressions directly within string literals. This feature provides a more natural and readable way to format strings.
String interpolation in C# is accomplished by prefixing a string literal with the $
symbol. Inside the string, you can embed expressions enclosed within curly braces {}
.
The expressions are evaluated at runtime, and their results are included in the final string. This makes it a powerful tool for formatting strings with dynamic content.
To convert an integer to hexadecimal using string interpolation, you can use the format specifier X
within the curly braces.
Here’s a basic example:
using System;
class Program {
static void Main() {
int number = 255;
string hex = $"{number:X}";
Console.WriteLine("Decimal: " + number);
Console.WriteLine("Hexadecimal: " + hex);
}
}
When you run this program, it will output:
Decimal: 255
Hexadecimal: FF
In this code, the value of number
is directly embedded into the string using string interpolation.
The format specifier :X
within the curly braces specifies that the value should be formatted as a hexadecimal string. The result is stored in the hex
variable, which will contain FF
in this example.
String interpolation allows you to easily customize the formatting of the hexadecimal representation.
For example, you can control the width and add leading zeros:
using System;
class Program {
static void Main() {
int number = 15;
string hex = $"{number:X2}";
Console.WriteLine("Decimal: " + number);
Console.WriteLine("Hexadecimal: " + hex);
}
}
When you run this program, it will output:
Decimal: 15
Hexadecimal: 0F
Here, we use string interpolation to directly embed the value of number
within the string, formatted as a two-character wide hexadecimal string with a leading zero using :X2
. We then display both the decimal and hexadecimal representations on the console.
Handling Negative Integers
When converting negative integers to hexadecimal using string interpolation, you should handle them similarly to other methods.
You can convert the negative integer to its unsigned representation before formatting it:
using System;
class Program {
static void Main() {
int negativeNumber = -42;
uint unsignedNumber = (uint)negativeNumber;
string hex = $"{unsignedNumber:X}";
Console.WriteLine("Original Negative Number: " + negativeNumber);
Console.WriteLine("Unsigned Number: " + unsignedNumber);
Console.WriteLine("Hexadecimal: " + hex);
}
}
When you run this program, it will output:
Original Negative Number: -42
Unsigned Number: 4294967254
Hexadecimal: FFFFFFD6
In this code, we first cast the negative integer negativeNumber
to an unsigned integer (unsignedNumber
) using (uint)
, and then we use string interpolation to create the hexadecimal representation.
The output includes the original negative number, the unsigned number, and the hexadecimal representation.
Handling Large Integers
For large integers, you can ensure that the hexadecimal representation includes all necessary digits by specifying the width using string interpolation:
using System;
class Program {
static void Main() {
int largeNumber = 50000;
string hex = $"{largeNumber:X8}";
Console.WriteLine("Decimal: " + largeNumber);
Console.WriteLine("Hexadecimal: " + hex);
}
}
When you run this program, it will output:
Decimal: 50000
Hexadecimal: 0000C350
Convert Hex to Int With the Convert.ToInt32()
Function in C#
In the previous sections, we discussed the methods to convert an integer value to a hexadecimal value.
Now, we will convert the same hexadecimal value from the previous examples back to an integer value in C#. The Convert
class provides the functionality of conversion between various base data types in C#.
The Convert.ToInt32()
function is a method that converts a specified value to a 32-bit signed integer. While its primary use is for converting various data types to integers, it can also be employed to convert hexadecimal strings into their equivalent integer values.
The Convert.ToInt32()
method in C# is used to convert a specified value to a 32-bit signed integer. The basic syntax of the Convert.ToInt32()
method is as follows:
int result = Convert.ToInt32(value, base);
Where:
value
is the value you want to convert to an integer. It can be of various data types, including string, double, decimal, etc.base
specifies the base (radix) of the value. This argument is optional. If omitted, the default base is10
. If you want to convert a hexadecimal string to an integer, you should specify the base as16
.
Here’s a simple example:
using System;
class Program {
static void Main() {
string hexString = "1A"; // Hexadecimal value
int intValue = Convert.ToInt32(hexString, 16);
Console.WriteLine("Hexadecimal String: " + hexString);
Console.WriteLine("Integer Value: " + intValue);
}
}
When you run this program, it will output:
Hexadecimal String: 1A
Integer Value: 26
In this code, we define a hexadecimal string hexString
with the value 1A
. We then use the Convert.ToInt32()
method with a base of 16
to convert the hexadecimal string to an integer.
The program displays both the original hexadecimal string and the resulting integer value on the console.
Handling Negative Hexadecimal Values
To handle negative hexadecimal values, you can use the Convert.ToInt32()
function in the same way. However, ensure that the input string includes the negative sign (-
) to indicate a negative value.
For example:
using System;
class Program {
static void Main() {
string hexString = "-1E"; // Negative hexadecimal value
int intValue = ConvertNegativeHexToInt(hexString);
Console.WriteLine("Hexadecimal String: " + hexString);
Console.WriteLine("Integer Value: " + intValue);
}
static int ConvertNegativeHexToInt(string hexString) {
if (hexString.StartsWith("-")) {
string positiveHex = hexString.Substring(1); // Remove the '-' sign
int intValue = Convert.ToInt32(positiveHex, 16);
return -intValue; // Make it negative
} else {
return Convert.ToInt32(hexString, 16);
}
}
}
When you run this program, it will output:
Hexadecimal String: -1E
Integer Value: -30
In this code, the hexString
represents the negative hexadecimal value -1E
, and the result is the integer value of -30
.
Custom Hexadecimal Values
The Convert.ToInt32()
function is flexible and can handle various hexadecimal formats. For example, you can use the 0x
prefix, which is common in programming, and still convert it to an integer:
using System;
class Program {
static void Main() {
string hexString = "0x10"; // Hexadecimal value with "0x" prefix
int intValue = Convert.ToInt32(hexString, 16);
Console.WriteLine("Hexadecimal String: " + hexString);
Console.WriteLine("Integer Value: " + intValue);
}
}
When you run this program, it will output:
Hexadecimal String: 0x10
Integer Value: 16
In this code, we define a hexadecimal string hexString
with the value 0x10
, which includes the 0x
prefix. We then use the Convert.ToInt32()
method with a base of 16
to convert the hexadecimal string to an integer.
The program displays both the original hexadecimal string and the resulting integer value on the console.
Exception Handling
When working with the Convert.ToInt32()
function for hexadecimal conversion, it’s essential to consider potential exceptions. For example, if you pass an invalid hexadecimal string, the function will throw a FormatException
or an OverflowException
if the value is out of the valid integer range.
Here’s a complete working C# code snippet that demonstrates how to handle exceptions when converting an invalid hexadecimal string using the Convert.ToInt32()
method:
using System;
class Program {
static void Main() {
string invalidHexString = "G123"; // Invalid hexadecimal value
int intValue = 0;
try {
intValue = Convert.ToInt32(invalidHexString, 16);
Console.WriteLine("Integer Value: " + intValue);
} catch (FormatException e) {
Console.WriteLine("Invalid Hexadecimal Format: " + e.Message);
} catch (OverflowException e) {
Console.WriteLine("Hexadecimal Value is out of Range: " + e.Message);
}
}
}
When you run this program with the invalid hexadecimal string G123
, it will output:
Invalid Hexadecimal Format: Could not find any recognizable digits.
In this code, we attempt to convert the invalid hexadecimal string G123
using the Convert.ToInt32()
method. Since the string is not in a valid hexadecimal format, it will throw a FormatException
exception, which is caught and handled appropriately.
The program provides an error message indicating the invalid hexadecimal format.
Conclusion
This article has provided a comprehensive overview of the different methods to convert an integer into a hexadecimal: ToString()
, String.Format
, and string interpolation. Each method has its unique advantages and use cases, and having a solid understanding of all of them equips you with the flexibility to handle a wide range of programming scenarios.
The ToString()
method offers simplicity and ease of use, with various format options to customize the output. String.Format
provides control over formatting and allows for precise width and alignment.
String interpolation offers a modern and concise way to embed expressions directly within string literals, simplifying the code. Finally, the Convert.ToInt32()
function is used for both converting integers to hexadecimal and converting hexadecimal back to integers since it handles various formats, including negative and custom hexadecimal strings.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Csharp Integer
- How to Convert Int to String in C#
- How to Convert Int to Enum in C#
- Random Int in C#
- Random Number in a Range in C#
- How to Convert String to Int in C#
- Integer Division in C#