How to Return an Array From a Function in C++
- Understanding Array Return Types in C++
- Method 1: Returning a Pointer to a Dynamically Allocated Array
- Method 2: Using std::array
- Method 3: Using std::vector
- Conclusion
- FAQ
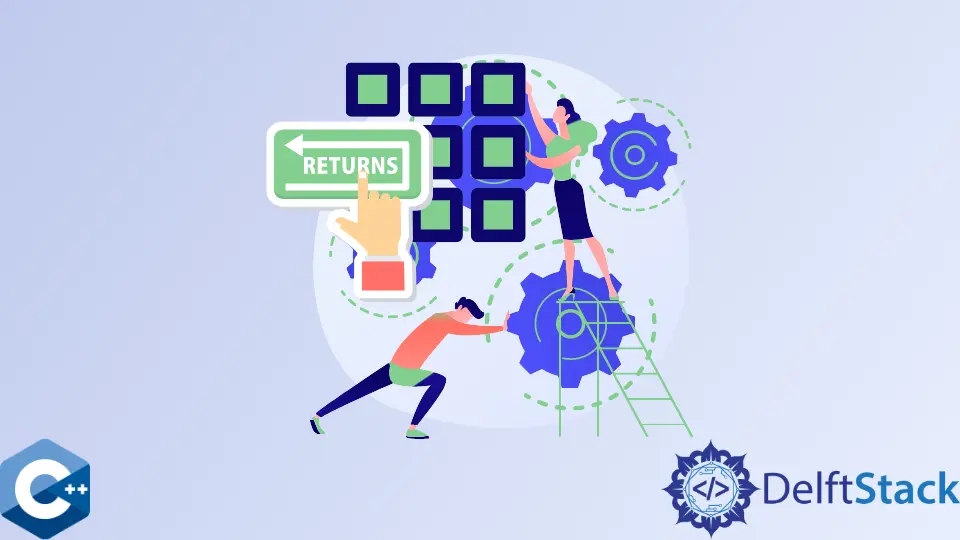
Returning an array from a function in C++ can be a bit tricky, especially for those new to the language. Unlike some other programming languages, C++ does not allow you to return arrays directly. However, there are several ways to effectively return an array from a function and access its elements.
This article will explore these methods, providing clear examples and explanations to help you understand the process. Whether you’re a beginner or looking to brush up on your C++ skills, this guide will equip you with the knowledge you need to handle arrays in functions confidently.
Understanding Array Return Types in C++
In C++, when you want to return an array from a function, you need to consider the limitations of the language. Directly returning an array is not possible because arrays decay into pointers when passed to functions. This means that the function cannot return the entire array as it would require returning a pointer instead. To work around this, you can use several methods, including returning a pointer to a dynamically allocated array, using std::array
or std::vector
, or passing an array by reference.
Method 1: Returning a Pointer to a Dynamically Allocated Array
One common method to return an array from a function is to dynamically allocate the array using new
and return a pointer to it. This way, the caller is responsible for deallocating the memory afterward to avoid memory leaks.
Here’s how you can do it:
#include <iostream>
int* createArray(int size) {
int* arr = new int[size];
for (int i = 0; i < size; i++) {
arr[i] = i * 10;
}
return arr;
}
int main() {
int size = 5;
int* myArray = createArray(size);
for (int i = 0; i < size; i++) {
std::cout << myArray[i] << " ";
}
delete[] myArray;
return 0;
}
Output:
0 10 20 30 40
In this example, the createArray
function dynamically allocates an array of integers. It fills the array with values that are multiples of 10. The function returns a pointer to the first element of the array. In the main
function, we call createArray
, store the returned pointer in myArray
, and then print the elements. Finally, we use delete[]
to free the allocated memory. This method is effective but requires careful memory management to prevent leaks.
Method 2: Using std::array
Another modern approach in C++ is to use std::array
, which is a part of the C++ Standard Library. This method allows you to return an array without worrying about memory management, as std::array
handles it for you.
Here’s a simple example:
#include <iostream>
#include <array>
std::array<int, 5> createArray() {
std::array<int, 5> arr;
for (int i = 0; i < arr.size(); i++) {
arr[i] = i * 10;
}
return arr;
}
int main() {
std::array<int, 5> myArray = createArray();
for (const auto& value : myArray) {
std::cout << value << " ";
}
return 0;
}
Output:
0 10 20 30 40
In this example, the createArray
function returns a std::array
of integers. The size of the array is fixed at compile time, which enhances safety and performance. In the main
function, we directly capture the returned array and print its contents. This method is highly recommended for its simplicity and safety, as std::array
automatically manages the memory.
Method 3: Using std::vector
If you need a more flexible array that can change size dynamically, std::vector
is the way to go. Vectors are part of the C++ Standard Library and provide dynamic resizing capabilities, making them ideal for a wide range of applications.
Here’s how you can return a vector from a function:
#include <iostream>
#include <vector>
std::vector<int> createVector(int size) {
std::vector<int> vec(size);
for (int i = 0; i < size; i++) {
vec[i] = i * 10;
}
return vec;
}
int main() {
int size = 5;
std::vector<int> myVector = createVector(size);
for (const auto& value : myVector) {
std::cout << value << " ";
}
return 0;
}
Output:
0 10 20 30 40
In this example, the createVector
function creates a std::vector
of integers. It initializes the vector with the specified size and fills it with multiples of 10. The vector is returned to the caller, where it can be used just like an array. This method is highly efficient and safe, as std::vector
manages memory automatically and can grow or shrink as needed.
Conclusion
Returning an array from a function in C++ may seem daunting at first, but with the right techniques, it becomes manageable. Whether you choose to return a pointer to a dynamically allocated array, use std::array
, or opt for std::vector
, each method has its advantages. Understanding these methods will not only enhance your C++ programming skills but also improve your ability to handle data structures effectively. With practice, you’ll find that working with arrays in functions becomes second nature.
FAQ
-
Can I return a regular array from a function in C++?
No, you cannot return a regular array directly. You can return a pointer to a dynamically allocated array, usestd::array
, orstd::vector
. -
What is the difference between std::array and std::vector?
std::array
has a fixed size known at compile time, whilestd::vector
can dynamically resize as elements are added or removed. -
How do I avoid memory leaks when using dynamic arrays?
Always remember to usedelete[]
to free the memory allocated withnew
once you are done using the array. -
Is using std::vector better than using raw pointers?
Yes,std::vector
provides automatic memory management, which reduces the risk of memory leaks and makes your code safer and easier to maintain. -
Can I return an array of different types from a function?
In C++, you cannot return an array of different types directly. You can usestd::variant
or a struct/class to encapsulate different types if needed.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ Function
- C++ Redefinition of Formal Parameter
- The ShellExecute() Function in C++
- The const Keyword in Function Declaration of Classes in C++
- How to Handle Arguments Using getopt in C++
- Time(NULL) Function in C++
- Cotangent Function in C++