The ShellExecute() Function in C++
-
Understanding the
ShellExecute()
Function in C++ -
Use Cases of
ShellExecute()
Function in C++ - Best Practices and Considerations
-
Advantages of Using the
ShellExecute()
Function -
Disadvantages of Using the
ShellExecute()
Function - Conclusion
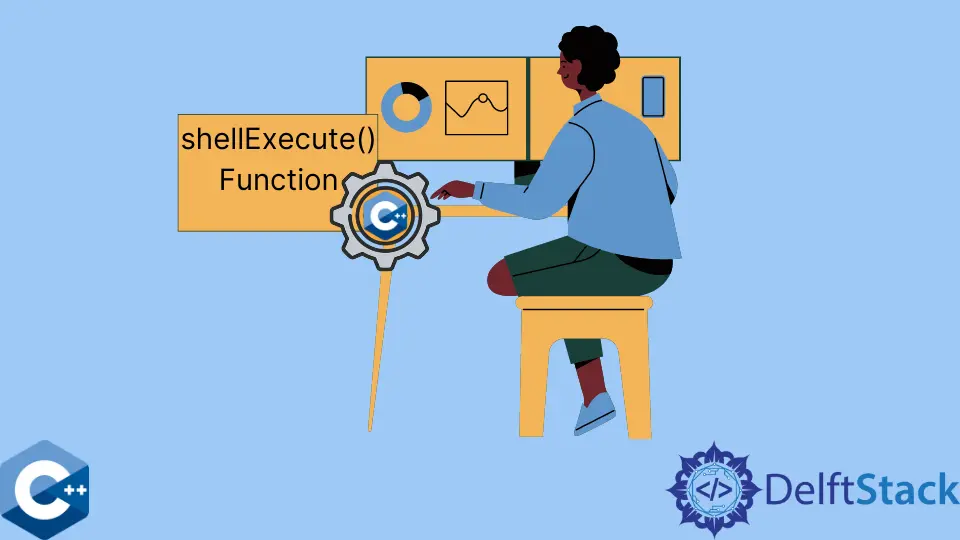
The ShellExecute()
function in C++ is a powerful tool that allows you to interact with the Windows Shell, enabling your program to perform various operations typically handled by the operating system itself. This function provides a seamless way to execute tasks such as opening files, URLs, launching applications, and more.
In this article, we’ll dive deep into the intricacies of the ShellExecute()
function, its parameters, use cases, and best practices.
Understanding the ShellExecute()
Function in C++
ShellExecute()
is a library function provided in the C++ standard library and is used for opening or running any executable file from the C++ program. You don’t need to take care of the program in which the required file will be opened; you only need to give the path or name of the file you need to open.
It is similar to Windows’ action of clicking an icon with the mouse, and it gets opened. This function is used to automate that action using C++ programming.
One of the main reasons ShellExecute()
is so well-liked is almost anything may be run using this API call. If the supplied file is not executable, the ShellExecute
API will search the registry for an appropriate program to launch the given file. For instance, if you provide a filename with the extension .txt
, it will likely launch Notepad with the file loaded.
The ShellExecute()
function is declared as follows:
HINSTANCE ShellExecute(HWND hwnd, LPCTSTR lpOperation, LPCTSTR lpFile,
LPCTSTR lpParameters, LPCTSTR lpDirectory, INT nShowCmd);
Let’s look at the details of the parameters:
hwnd(HWND)
: This parameter specifies the handle of the parent window or owner window for the new process. It helps to determine the parent-child relationship between windows.lpOperation (LPCTSTR)
: It is the name of the action that needs to be performed on the file. Below is the list of actions that can be used. These operations determine how the specified file or resource will be handled.open
orNULL
: ThelpFile
file is opened by the function. The file may be a document file or an executable file. We can also specify a folder name to open.print
: If the file specified bylpFile
is a document file, the function sends it to the printer for printing.explore
: If the file specified bylpFile
is the folder’s name, the function explores that folder.
lpFile (LPCTSTR)
: This parameter specifies the file or resource to be executed or opened. It can be a path to an executable, a document file, or a URL.lpParameters (LPCTSTR)
: If the operation requires additional parameters, they can be specified in this parameter. For example, if you’re opening a document, this could include specific instructions or options.lpDirectory (LPCTSTR)
: This is the working directory for the new process. If the operation requires a specific working directory, it can be set here.nShowCmd (INT)
: This parameter defines how the window should be displayed. Its value can be any of the following:SW_HIDE
: It shows the new application window after hiding the current one.W_MAXIMIZE
: It shows the specified window in maximized form.SW_MINIMIZE
: It shows the specified window in minimized form and displays the next-level window.SW_SHOW
: It activates the specified window in its default size and positionSW_SHOWMAXIMIZED
: It first activates the window and shows it in maximized form.SW_SHOWMINIMIZED
: It first activates the window and shows it in minimized form.SW_SHOWNORMAL
: enables and shows a window. Windows returns the window to its original size and location if it is minimized or maximized. When a window is first displayed, an application must provide this flag.- If
lpFile
is a document file specified, then thenShowCmd
value should be set to0
.
The function returns an instance handle (HINSTANCE
) that identifies the new process if successful. Otherwise, it returns an error code, typically SE_ERR_XXX
constants indicating the type of error.
On success, ShellExecute()
returns a value greater than 31
; otherwise, its return value can be:
0
: If the operating system does not provide memory or resources.ERROR_FILE_NOT_FOUND
: The file specified is not found at the location.ERROR_PATH_NOT_FOUND
: The path specified is wrong or is not found.ERROR_BAD_FORMAT
: There is an invalid.exe
file provided.
There can also be many other error codes, but we have discussed some common errors.
Use Cases of ShellExecute()
Function in C++
- Opening Files: One of the most common uses is to open files using the default associated application. For example, opening a PDF document with the default PDF reader.
- Launching URLs: You can use
ShellExecute()
to open web pages in the user’s default browser. - Executing Programs: It can be used to run executable files, which is especially useful for launching external tools or applications from your C++ program.
- Printing Documents: You can use
ShellExecute()
to print documents using the default printer. - Exploring Directories: The
"explore"
operation opens the specified directory in Windows Explorer.
Let us look at the coding example of the ShellExecute()
function:
#include <bits/stdc++.h>
#include <windows.h>
using namespace std;
int main() {
ShellExecute(NULL, "open", "MyTextFile.txt", NULL, NULL, SW_SHOWMAXIMIZED);
return 0;
}
This code uses ShellExecute()
to open the file "MyTextFile.txt"
with the default associated application. If successful, it returns 0
to the operating system.
After the code is run, MyTextFile
will be opened in the Notepad program. Similarly, we can provide a URL as well that will be opened in the browser like this:
#include <bits/stdc++.h>
#include <windows.h>
using namespace std;
int main() {
char webUrl[100] = "www.google.com";
ShellExecute(NULL, "open", webUrl, NULL, NULL, SW_SHOWNORMAL);
return 0;
}
Below is another example that utilizes the Windows API function ShellExecute()
for executing operations typically handled by the operating system.
#include <Windows.h>
int main() {
HINSTANCE result = ShellExecute(NULL, // hwnd
L"open", // operation
L"notepad.exe", // file
NULL, // parameters
NULL, // directory
SW_SHOWNORMAL // show command
);
if ((INT_PTR)result <= 32) {
// Handle error
switch ((INT_PTR)result) {
case SE_ERR_FNF:
// File not found
break;
// Add more cases as needed
default:
// Handle other errors
break;
}
}
return 0;
}
First, it includes the necessary Windows header file (Windows.h
). The main()
function is the program’s entry point.
Within main()
, ShellExecute()
is called. This function attempts to open "notepad.exe"
, the default text editor in Windows. Here’s the breakdown of the parameters:
NULL
: No specific parent window handle is provided.L"open"
: This specifies the operation to perform, which is to open the file or application.L"notepad.exe"
: This is the name of the file or application to be executed.NULL
: Since no additional parameters are needed, this is set toNULL
.NULL
: Similarly, no specific directory is specified.SW_SHOWNORMAL
: This parameter determines how the window will be displayed. In this case, it’s set toSW_SHOWNORMAL
, which means the window will be displayed in its default state.
The return value of ShellExecute()
is stored in result
, which is of type HINSTANCE
. If (INT_PTR)result
is less than or equal to 32
, it implies an error occurred.
In such cases, the program enters a switch statement to handle specific error cases. For instance, if SE_ERR_FNF
is returned, it means the file was not found. You can extend this switch statement to handle other error cases.
Finally, the program returns 0
, indicating successful execution.
Best Practices and Considerations
- Error Handling: Always check the return value of
ShellExecute()
to handle potential errors. If an error occurs, you can useGetLastError()
to retrieve the specific error code. - Security Considerations: Be cautious when using user-provided input directly in the
lpFile
orlpParameters
parameters to avoid potential security vulnerabilities. - Handle Return Value: Depending on the operation performed, you may want to take different actions. For example, if the operation is
"open"
, you might not need to wait for the process to complete. However, if it’s"print"
, you might want to ensure that the print job is completed. - Window Handle (
hwnd
): Providing the correct window handle can be crucial, especially if you want to maintain a specific UI behavior or parent-child relationship. - Cross-Platform Considerations: Keep in mind that
ShellExecute()
is a Windows-specific function. If you’re developing a cross-platform application, you may need to use platform-specific approaches for other operating systems.
Advantages of Using the ShellExecute()
Function
This API call can open, print, or browse files or folders using the standard Windows settings. You’ll be able to change those defaults as well.
Not all parameters must be filled in; several of them can be NULL (i.e., ease of use).
Disadvantages of Using the ShellExecute()
Function
16-bit software cannot be run via ShellExecute
. Comparable to WinExec
in complexity.
It is unable to indicate when the generated program has been completed.
Conclusion
The ShellExecute()
function in C++ is a versatile tool that empowers your program to interact with the Windows Shell seamlessly. Understanding its syntax, parameters, use cases, best practices, advantages, and disadvantages is essential for harnessing its full potential. By leveraging ShellExecute()
, you can provide a more dynamic and user-friendly experience in your Windows applications.