Cotangent Function in C++
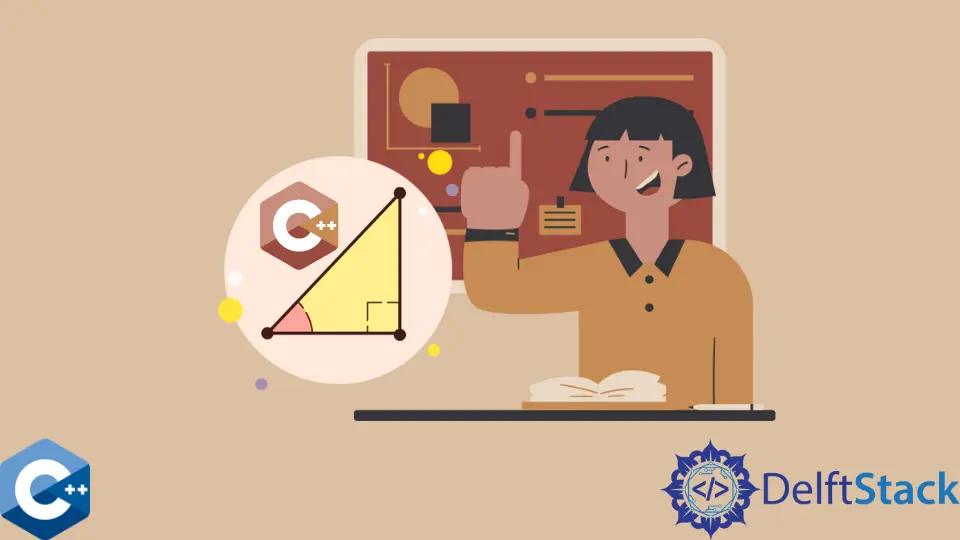
This brief guide is about the solution of trigonometric functions in C++. Many of the functions are readily available in the math
library, but some are unavailable, e.g., the cotangent
function.
For such functions, we will implement some efficient user-defined functions.
Trigonometric Functions in C++
Mathematical computations can be performed using the mathematical functions supplied in the math
or cmath
libraries in the C++ programming language.
These mathematical functions have been designed to perform difficult calculations. We will focus on trigonometric functions for this article, specifically sin
, cos
, tan
, and cot
.
Sine Function in C++
The sin
method is used to find the sine of an angle provided as a radian argument. This function takes one double integer as an input and returns the value of sin(x)
as a double integer.
double sin(double)
Before using these functions, remember to include the math.h
library file.
#include <math.h>
#include <iostream>
using namespace std;
int main() {
double a = 90;
cout << "sin ( " << a << " ) = " << sin(a) << endl;
}
Output:
Cosine Function in C++
To calculate the cos
of an angle provided as a radian argument, use the cosine
or cos
technique. This function takes one double integer as an input and returns the cos(x)
value as a double integer.
The syntax is as follows.
double cos(double)
The example to implement cos
function is:
#include <math.h>
#include <iostream>
using namespace std;
int main() {
double a = 90;
cout << "cos ( " << a << " ) = " << cos(a) << endl;
}
Output:
Tangent Function in C++
The tangent
or tan
technique is utilized to compute the tangent of an angle provided as a radian parameter. This function takes one double integer as an input and returns the value of tan(x) = sin(x)/cos(x)
as a double integer.
Syntax of this function is:
double tan(double)
Below is the code snippet implementing the tan
function.
#include <math.h>
#include <iostream>
using namespace std;
int main() {
double a = 90;
cout << "tan ( " << a << " ) = " << tan(a) << endl;
}
Output:
These are the basic trigonometric functions provided in the math
library. Apart from this, there are inverse functions of sin
, cos
and tan
also available, i.e., asin
, acos
, and atan
, which are the multiplicative inverse of sin
, cos
, and tan
, respectively.
But this math
library doesn’t provide the functionality for the functions like sec
, cosec
, and cot
of trigonometry, which are the reciprocal of sin
, cos
, and tan
, respectively.
Therefore, to implement these functions, we need to create user-defined functions. Our focus for this article is the cotangent
function, which is the reciprocal of the tan
function.
Cotangent Function in C++
There can be two methods to compute any radian value’s cotangent or cot
.
- take reciprocal of
tan
function - calculate
cos(x)/sin(x)
The following code snippet will calculate the cot
of the given radian value.
#include <math.h>
#include <iostream>
using namespace std;
double cot(double a) { return cos(a) / sin(a); }
int main() {
double a = 90;
cout << "cot ( " << a << " ) = " << cot(a) << endl;
}
Output:
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn