C++ Redefinition of Formal Parameter
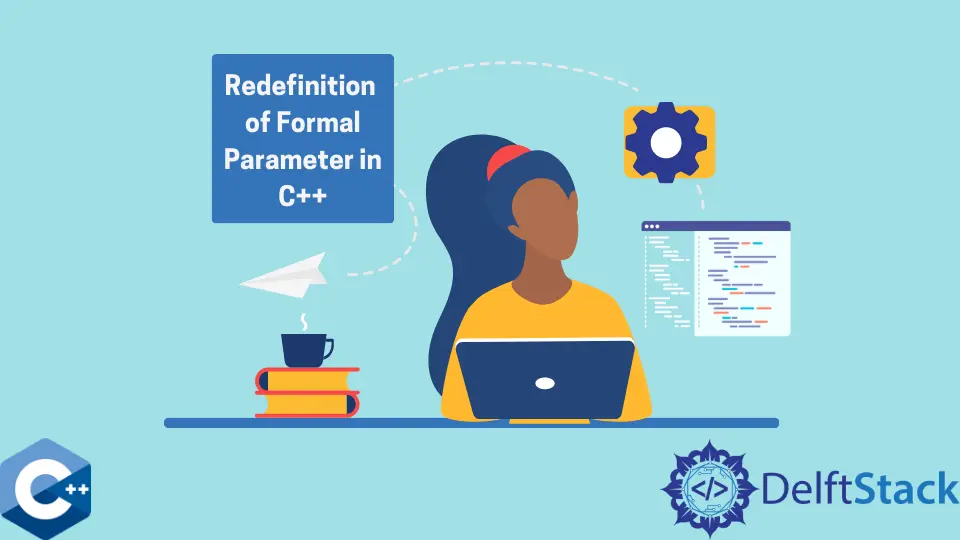
In this tutorial, we will discuss the issue of redefinition of formal parameters in C++.
First, we will discuss function definition and formal parameters. Next, we will cover the issue of redefinition of formal parameters.
Function Definition in C++
In programming, parameter and arguments are alternatively used. However, formally, there are few differences between them.
Parameters are written inside the parenthesis, which comes after the function name in the signature in the function header. Arguments are the values sent with a function call.
Note: Sometimes, arguments are called parameters, and parameters are called formal parameters.
It is essential to clear this terminology before further discussion. So, let’s look at a function header below:
void add (int a, int b)
See the signature of the add
function. Here, a
and b
are formal parameters.
See call to the add
function:
int result = add(2, 5);
Inside the function call, 2
and 5
are arguments sent to the function, whereas a
and b
formal parameters of the function add
will receive these values. It is important to note that besides formal parameters, a
and b
are also local variables of the function add
.
The difference between other local variables and formal parameters is that formal parameters receive values from outside the function whenever a function is called. However, the other local variables can’t receive values outside the function.
See another code:
void add(int a, int b) {
int result = a + b;
cout << "Result: " << result << '\n';
}
add(2, 5);
In this code example, there are two formal parameters (i.e., a
and b
) and three local variables (i.e., result
, a
, and b
). Inside the add
function, you can use all three variables similarly.
You may take input in these variables, assign some constant value or variable, and use them in expression.
After getting an idea about formal parameters, now let’s talk about the issue of redefinition of formal parameters.
Redefinition of Formal Parameter in C++
Being a programmer of C++, you must have an idea that there can’t be two variables with the same name in the same scope. For example, you can’t declare global variables of the same name (type doesn’t matter).
You can’t declare data members of the same name in the same class. Similarly, you can’t declare two local variables of the same name.
Let’s look at the following example to understand it better:
int a;
char a;
On compilation, this code gives the following error:
redefinion1.cpp:6:6: error: conflicting declaration ‘char a’
char a;
^
redefinion1.cpp:5:5: note: previous declaration as ‘int a’
int a;
Similar issue in the class definition:
class A {
int a;
int a;
};
On compilation, this code gives the following error:
redefinion1.cpp:9:6: error: redeclaration of ‘int A::a’
int a;
^
redefinion1.cpp:8:6: note: previous declaration ‘int A::a’
int a;
The message may be different, but the issue is the same, i.e., two same-name variables can’t exist in the same scope. However, having the same name variables in different scopes is possible.
For example, the below code will compile without an error:
#include <iostream>
using namespace std;
int a = 4;
void f() { cout << a << '\n'; }
int main() {
int a = 3;
cout << a << '\n';
f();
return 0;
}
The output of this code is:
3
4
In the main function, only local variable a
is visible/accessible (global variable is shadowed by local a
in this scope). Hence, the cout
statement prints the value of local variable a
, which is 3
.
In function f
, the global variable a
is visible/accessible, and the print
statement prints the value of the global variable, which is 4
.
Sometimes, by mistake, the programmers can use both formal parameters and local variables of the same name. In this case, both of them exist in the same scope.
Therefore, the compiler generates an error.
Let’s have a look at the code and the response of the compiler:
void f(int x) { int x = 4; }
Output:
redefinion1.cpp: In function ‘void f(int)’:
redefinion1.cpp:6:6: error: declaration of ‘int x’ shadows a parameter
int x = 4;
^
In the above code, function f
has a formal parameter x
and a local variable x
, both of which are local variables of function f
. Consequently, you can see the compiler error declaration of 'int x' shadows a parameter
, which means a local variable and a formal parameter have the same name.
In short, being a programmer of C++, you must be careful not to declare the local variables with similar names as the formal parameters. Otherwise, the compiler will not distinguish between them and generates the error.