How to Handle Arguments Using getopt in C++
- Understanding getopt in C++
- Parsing Command-Line Arguments with getopt
- Advanced Usage of getopt
- Conclusion
- FAQ
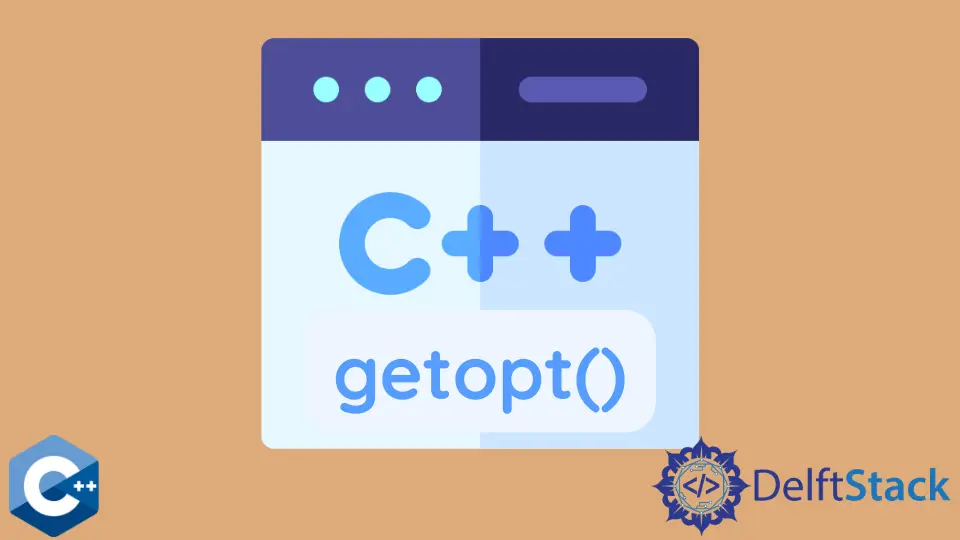
When developing applications in C++, handling command-line arguments can be a crucial aspect of your program’s functionality. One of the most effective ways to manage these arguments is by using the getopt
function. This powerful tool allows you to parse command-line options easily, enabling you to create user-friendly interfaces for your applications.
In this article, we will explore how to use getopt
in C++ to handle various arguments. We will break down the process step by step, providing clear code examples and explanations. By the end of this article, you’ll be equipped with the knowledge to enhance your C++ applications with robust command-line argument handling.
Understanding getopt in C++
The getopt
function is a standard library function in C and C++ that provides a way to parse command-line options. It allows you to specify short options (like -a
, -b
) and long options (like --all
, --help
). The basic syntax of getopt
is straightforward, making it an excellent choice for handling command-line arguments in your C++ programs.
Here’s a simple illustration of how to use getopt
:
#include <iostream>
#include <unistd.h>
int main(int argc, char *argv[]) {
int opt;
while ((opt = getopt(argc, argv, "ab:c")) != -1) {
switch (opt) {
case 'a':
std::cout << "Option a selected\n";
break;
case 'b':
std::cout << "Option b selected with value: " << optarg << "\n";
break;
case 'c':
std::cout << "Option c selected\n";
break;
default:
std::cerr << "Unknown option\n";
return 1;
}
}
return 0;
}
Output:
Option a selected
Option b selected with value: value
Option c selected
In this example, we include the necessary headers and define the main
function. We use a while
loop to iterate through the command-line arguments. The getopt
function takes three parameters: argc
, argv
, and a string of valid options. Each valid option can be followed by a colon if it requires an argument. In the switch statement, we handle each option accordingly, outputting relevant messages.
Parsing Command-Line Arguments with getopt
Now that we have a basic understanding of getopt
, let’s delve deeper into how to effectively parse command-line arguments. One of the key advantages of using getopt
is its ability to handle both short and long options seamlessly. This flexibility allows developers to create more intuitive command-line interfaces.
Here’s an enhanced example that incorporates both short and long options:
#include <iostream>
#include <unistd.h>
#include <cstring>
int main(int argc, char *argv[]) {
int opt;
while ((opt = getopt(argc, argv, "a:b:c")) != -1) {
switch (opt) {
case 'a':
std::cout << "Option a selected with value: " << optarg << "\n";
break;
case 'b':
std::cout << "Option b selected with value: " << optarg << "\n";
break;
case 'c':
std::cout << "Option c selected\n";
break;
case '?':
if (optopt == 'a' || optopt == 'b') {
std::cerr << "Option -" << static_cast<char>(optopt) << " requires an argument\n";
} else {
std::cerr << "Unknown option: " << static_cast<char>(optopt) << "\n";
}
return 1;
default:
return 1;
}
}
return 0;
}
Output:
Option a selected with value: valueA
Option b selected with value: valueB
Option c selected
In this code, we handle cases where options require arguments. If a required argument is missing, an error message is displayed. The getopt
function also sets the global variable optopt
to indicate which option caused the error, allowing for more informative error messages.
Advanced Usage of getopt
As your application grows, you may need to handle more complex scenarios, such as parsing long options or combining multiple options. The getopt_long
function, available in the <getopt.h>
header, allows you to do just that. This extended version of getopt
can parse both short and long options, making it more versatile.
Here’s an example illustrating how to use getopt_long
:
#include <iostream>
#include <unistd.h>
#include <getopt.h>
int main(int argc, char *argv[]) {
int opt;
struct option long_options[] = {
{"help", no_argument, nullptr, 'h'},
{"version", no_argument, nullptr, 'v'},
{"output", required_argument, nullptr, 'o'},
{nullptr, 0, nullptr, 0}
};
while (true) {
int option_index = 0;
opt = getopt_long(argc, argv, "hvo:", long_options, &option_index);
if (opt == -1) break;
switch (opt) {
case 'h':
std::cout << "Help message\n";
break;
case 'v':
std::cout << "Version 1.0\n";
break;
case 'o':
std::cout << "Output file: " << optarg << "\n";
break;
default:
return 1;
}
}
return 0;
}
Output:
Help message
Version 1.0
Output file: output.txt
In this example, we define a structure array long_options
to specify long options. Each entry in the array corresponds to a long option, indicating whether it requires an argument. The getopt_long
function works similarly to getopt
, but it also recognizes long options, providing a more user-friendly experience.
Conclusion
Handling command-line arguments in C++ using getopt
can significantly enhance your application’s usability. Whether you are dealing with simple options or more complex scenarios involving long options, getopt
provides a robust solution. By following the examples and explanations provided in this article, you should now feel confident in implementing command-line argument parsing in your C++ applications. Remember, a well-designed command-line interface can greatly improve user experience and make your applications more versatile.
FAQ
-
What is getopt in C++?
getopt is a function used in C and C++ to parse command-line options and arguments easily. -
How do I handle options that require arguments using getopt?
You can specify a colon after the option character in the option string to indicate that it requires an argument. -
Can I use long options with getopt?
Yes, you can use the getopt_long function to handle both short and long options in your C++ applications. -
What should I do if a required argument is missing?
You can check for the optopt variable to determine which option is missing an argument and provide an appropriate error message. -
Is getopt available in all C++ compilers?
Yes, getopt is part of the standard library and is available in most C++ compilers.
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn