在 C++ 中使用 getopt 处理参数
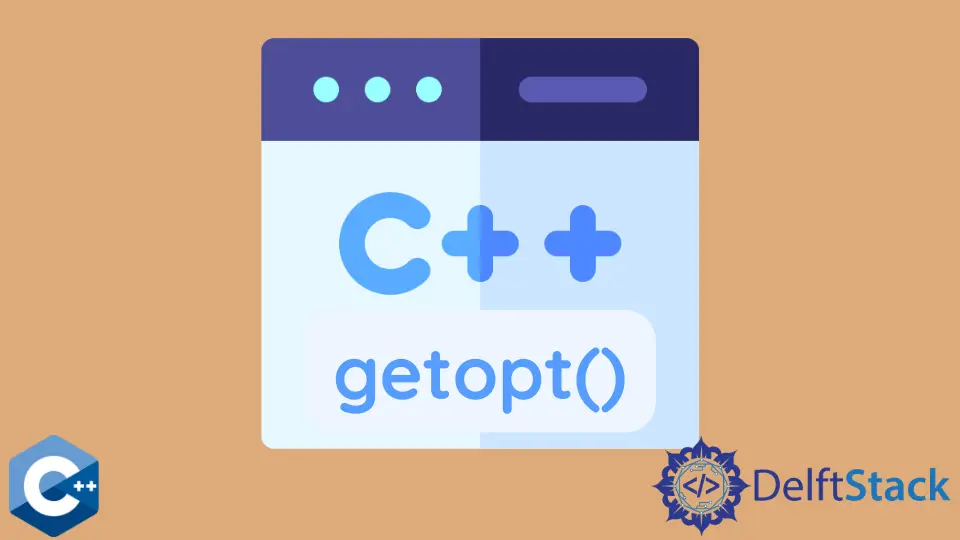
本文演示了如何使用 getopt()
函数来处理命令行中传递给代码的参数。本教程还解释了如何操作代码以执行特定输入的某些操作。
在 C++ 中使用 getopt()
函数处理参数
假设我们有如下所示的代码。
// File titled arg_test.cpp
#include <stdio.h>
#include <unistd.h>
int main(int argc, char *argv[]) {
int opt;
while ((opt = getopt(argc, argv, ":f:asd")) != -1) {
if (opt == 'a' || opt == 's' || opt == 'd') {
printf("Option Selected is: %c\n", opt);
} else if (opt == 'f') {
printf("Filename entered is: %s\n", optarg);
} else if (opt == ':') {
printf("Option requires a value \n");
} else if (opt == '?') {
printf("Unknown option: %c\n", optopt);
}
}
// optind is for the extra arguments that are not parsed by the program
for (; optind < argc; optind++) {
printf("Extra arguments, not parsed: %s\n", argv[optind]);
}
return 0;
}
getopt()
函数的语法如下:
getopt(int argc, char *const argv[], const char *optstring)
argc
是一个整数,argv
是一个字符数组。optstring
是一个字符列表,每个字符代表一个单个字符长的选项。
该函数在处理完输入中的所有内容后返回 -1
。
getopt()
函数在遇到它不认识的东西时返回 ?
。此外,这个无法识别的选项存储在外部变量 optopt
中。
默认情况下,如果选项需要一个值,例如,在我们的例子中,选项 f
需要一个输入值,所以 getopt()
将返回 ?
。但是,通过将冒号 (:
) 作为 optstring
的第一个字符,该函数返回:
而不是 ?
。
在我们的例子中,输入 a
、s
或 d
应该返回选项本身,使用 f
应该返回作为参数提供的文件名,其他所有内容都不应被视为有效选项。
要运行上述代码,你可以在终端中使用类似的命令:
gcc arg_test.cpp && ./a.out
当然,仅仅输入这个不会给你任何有用的输出。我们将输入一个示例用例和一个示例输出,并解释如何处理每个命令。
如果我们输入以下命令作为示例:
gcc arg_test.cpp && ./a.out -f filename.txt -i -y -a -d testingstring not_an_option
我们将得到以下输出:
Filename entered is: filename.txt
Unknown option: i
Unknown option: y
Option Selected is: a
Option Selected is: d
Extra arguments, not parsed: testingstring
Extra arguments, not parsed: not_an_option
选项 a
和 d
按预期返回。f
标志后给出的文件名也被报告,选项 i
和 y
进入 ?
类别并被识别为未知命令。
在这里,我们可以看到除了上述场景之外,我们在 getopt()
函数之后添加的最后一个循环处理了该函数未处理的剩余参数(testingstring
和 not_an_option
,在我们的例子中)。
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn