C++ 中的函数重载
Jinku Hu
2023年10月12日
C++
C++ Function
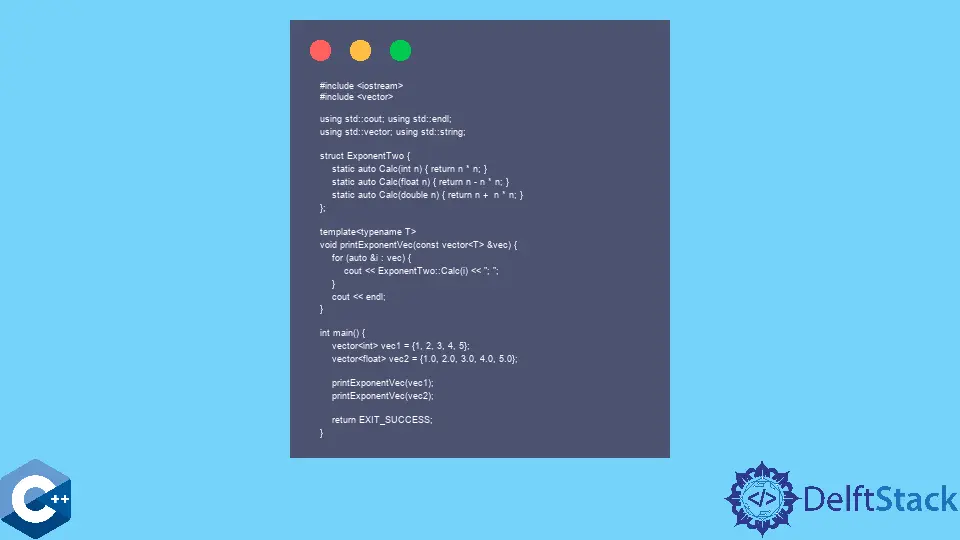
本文将演示在 C++ 中使用函数重载的多种方法。
在 C++ 中定义重载函数
函数重载被称为临时多态性的一部分,它提供了可应用于不同类型对象的函数。也就是说,这些函数在给定的范围内定义为相同的名称,并且它们的参数数量或类型应该不同。请注意,重载函数只是一组在编译时解析的不同函数。后一个特性将它们与用于虚函数的运行时多态区分开来。编译器观察参数的类型并选择要调用的函数。
重载函数的参数类型或参数数量必须不同。否则会出现编译错误。即使重载函数的返回类型不同或使用别名类型作为参数,编译器也会失败并出现错误。在下面的示例中,我们实现了一个 Math
结构,该结构具有三个用于数字求和的 static
函数。每个函数采用不同类型的参数对并返回相应的类型。因此,可以使用不同类型的向量元素调用 Math::sum
。请注意,struct
关键字在 C++ 中定义了一个类,其成员默认是公共的。
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::string;
using std::vector;
struct Math {
static auto sum(int x, int y) { return x + y; }
static auto sum(float x, float y) { return x + y; }
static auto sum(double x, double y) { return x + y; }
};
int main() {
vector<int> vec1 = {1, 2, 3, 4, 5};
vector<float> vec2 = {1.0, 2.0, 3.0, 4.0, 5.0};
for (const auto &item : vec1) {
cout << Math::sum(item, item * 2) << "; ";
}
cout << endl;
for (const auto &item : vec2) {
cout << Math::sum(item, item * 2) << "; ";
}
cout << endl;
return EXIT_SUCCESS;
}
输出:
3; 6; 9; 12; 15;
3; 6; 9; 12; 15;
在 C++ 中使用带有模板的重载函数
模板是一种用 C++ 语言实现的通用编程形式。它具有与函数重载相似的特性,因为两者都是在编译时推断出来的。请注意,以下代码片段具有输出通用向量元素的 printExponentVec
函数。因此它被用来对每个类型进行相同的操作,但是当我们要为不同的类型实现不同的函数体时,就应该使用函数重载。
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::string;
using std::vector;
struct ExponentTwo {
static auto Calc(int n) { return n * n; }
static auto Calc(float n) { return n - n * n; }
static auto Calc(double n) { return n + n * n; }
};
template <typename T>
void printExponentVec(const vector<T> &vec) {
for (auto &i : vec) {
cout << ExponentTwo::Calc(i) << "; ";
}
cout << endl;
}
int main() {
vector<int> vec1 = {1, 2, 3, 4, 5};
vector<float> vec2 = {1.0, 2.0, 3.0, 4.0, 5.0};
printExponentVec(vec1);
printExponentVec(vec2);
return EXIT_SUCCESS;
}
输出:
1; 4; 9; 16; 25;
0; -2; -6; -12; -20;
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu