C++ 中的函式過載
Jinku Hu
2023年10月12日
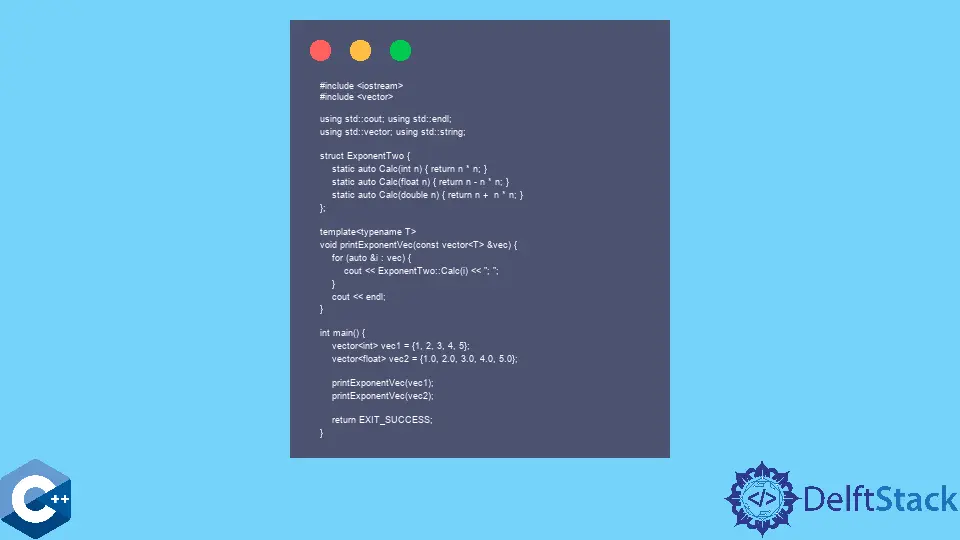
本文將演示在 C++ 中使用函式過載的多種方法。
在 C++ 中定義過載函式
函式過載被稱為臨時多型性的一部分,它提供了可應用於不同型別物件的函式。也就是說,這些函式在給定的範圍內定義為相同的名稱,並且它們的引數數量或型別應該不同。請注意,過載函式只是一組在編譯時解析的不同函式。後一個特性將它們與用於虛擬函式的執行時多型區分開來。編譯器觀察引數的型別並選擇要呼叫的函式。
過載函式的引數型別或引數數量必須不同。否則會出現編譯錯誤。即使過載函式的返回型別不同或使用別名型別作為引數,編譯器也會失敗並出現錯誤。在下面的示例中,我們實現了一個 Math
結構,該結構具有三個用於數字求和的 static
函式。每個函式採用不同型別的引數對並返回相應的型別。因此,可以使用不同型別的向量元素呼叫 Math::sum
。請注意,struct
關鍵字在 C++ 中定義了一個類,其成員預設是公共的。
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::string;
using std::vector;
struct Math {
static auto sum(int x, int y) { return x + y; }
static auto sum(float x, float y) { return x + y; }
static auto sum(double x, double y) { return x + y; }
};
int main() {
vector<int> vec1 = {1, 2, 3, 4, 5};
vector<float> vec2 = {1.0, 2.0, 3.0, 4.0, 5.0};
for (const auto &item : vec1) {
cout << Math::sum(item, item * 2) << "; ";
}
cout << endl;
for (const auto &item : vec2) {
cout << Math::sum(item, item * 2) << "; ";
}
cout << endl;
return EXIT_SUCCESS;
}
輸出:
3; 6; 9; 12; 15;
3; 6; 9; 12; 15;
在 C++ 中使用帶有模板的過載函式
模板是一種用 C++ 語言實現的通用程式設計形式。它具有與函式過載相似的特性,因為兩者都是在編譯時推斷出來的。請注意,以下程式碼片段具有輸出通用向量元素的 printExponentVec
函式。因此它被用來對每個型別進行相同的操作,但是當我們要為不同的型別實現不同的函式體時,就應該使用函式過載。
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::string;
using std::vector;
struct ExponentTwo {
static auto Calc(int n) { return n * n; }
static auto Calc(float n) { return n - n * n; }
static auto Calc(double n) { return n + n * n; }
};
template <typename T>
void printExponentVec(const vector<T> &vec) {
for (auto &i : vec) {
cout << ExponentTwo::Calc(i) << "; ";
}
cout << endl;
}
int main() {
vector<int> vec1 = {1, 2, 3, 4, 5};
vector<float> vec2 = {1.0, 2.0, 3.0, 4.0, 5.0};
printExponentVec(vec1);
printExponentVec(vec2);
return EXIT_SUCCESS;
}
輸出:
1; 4; 9; 16; 25;
0; -2; -6; -12; -20;
作者: Jinku Hu