How to Convert Integer to String in Arduino
- Method 1: Using the String Class
-
Method 2: Using the
itoa()
Function - Method 3: Using String Concatenation
- Method 4: Converting String to Integer
- Conclusion
- FAQ
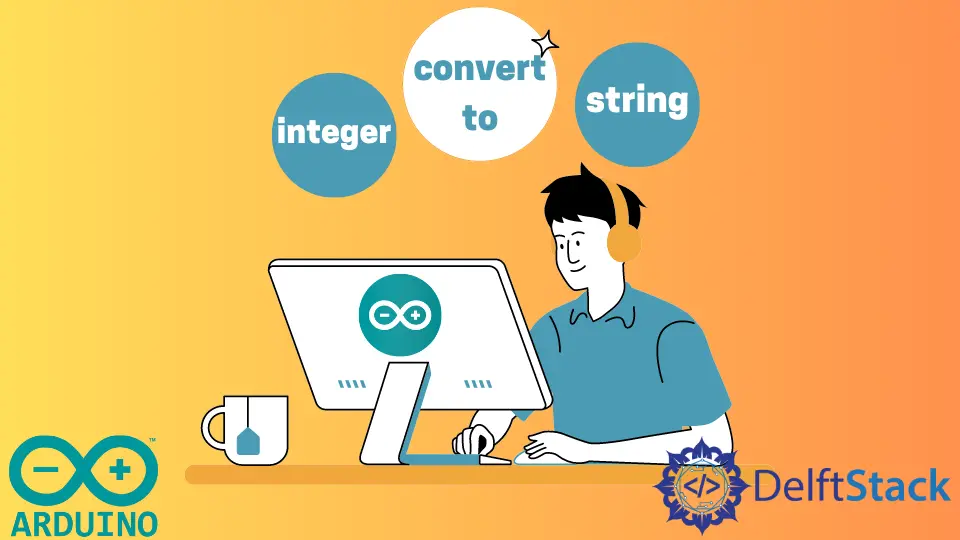
Converting integers to strings in Arduino is a common task that many developers encounter. Whether you’re working on a project that involves displaying values on an LCD or sending data over serial communication, knowing how to perform this conversion is crucial.
In this article, we’ll explore various methods to seamlessly convert integers to strings and vice versa in Arduino. With clear explanations and practical examples, you’ll have the tools you need to handle these conversions with confidence. Let’s dive into the world of Arduino programming and simplify the integer-to-string conversion process!
Method 1: Using the String Class
One of the most straightforward ways to convert an integer to a string in Arduino is by using the built-in String class. This method is particularly user-friendly and allows for easy manipulation of string data.
Here’s a simple example of how to perform this conversion:
void setup() {
Serial.begin(9600);
int number = 1234;
String strNumber = String(number);
Serial.println(strNumber);
}
void loop() {
}
Output:
1234
In this example, we start by initializing the serial communication at a baud rate of 9600. We then declare an integer variable named number
and assign it the value 1234
. The conversion to a string is done using String(number)
, which creates a new String object containing the integer’s value. Finally, we print the string representation of the integer to the Serial Monitor using Serial.println()
. This method is efficient and easy to read, making it a great choice for many applications.
Method 2: Using the itoa()
Function
Another effective way to convert integers to strings in Arduino is by using the itoa()
function. This function is part of the standard C library, and it allows for more control over the conversion process, especially when dealing with different number bases.
Here’s how you can use itoa()
to convert an integer to a string:
void setup() {
Serial.begin(9600);
int number = 5678;
char strNumber[10];
itoa(number, strNumber, 10);
Serial.println(strNumber);
}
void loop() {
}
Output:
5678
In this code snippet, we initialize the serial communication and declare an integer variable number
with the value 5678
. We then create a character array strNumber
to hold the converted string. The itoa()
function is called with three parameters: the integer to convert, the character array to store the result, and the base for conversion (in this case, base 10 for decimal). Finally, we print the converted string to the Serial Monitor. This method is particularly useful when you need to specify different bases, such as binary or hexadecimal.
Method 3: Using String Concatenation
Sometimes, you might want to convert an integer to a string by concatenating it with an empty string. This method is less common but can be useful in certain situations.
Here’s an example of how to do this:
void setup() {
Serial.begin(9600);
int number = 91011;
String strNumber = "" + String(number);
Serial.println(strNumber);
}
void loop() {
}
Output:
91011
In this example, we again initialize the serial communication and declare an integer variable number
with the value 91011
. The conversion is performed by concatenating an empty string with String(number)
. This technique effectively converts the integer to a string, which is then printed to the Serial Monitor. While this method is less efficient than the previous ones, it can be handy in scenarios where you are already working with strings.
Method 4: Converting String to Integer
Now that we’ve covered how to convert integers to strings, let’s briefly touch on how to convert strings back to integers. This is equally important, especially when you need to process user input or data received from sensors.
Here’s an example using the toInt()
method of the String class:
void setup() {
Serial.begin(9600);
String strNumber = "12345";
int number = strNumber.toInt();
Serial.println(number);
}
void loop() {
}
Output:
12345
In this example, we start with a string representation of a number, strNumber
, initialized to “12345”. The toInt()
method is called on this string, converting it back to an integer. The result is then printed to the Serial Monitor. This method is straightforward and efficient, making it an excellent choice for converting strings back to integers in Arduino projects.
Conclusion
In this article, we’ve explored several methods to convert integers to strings and vice versa in Arduino. From using the String class and the itoa()
function to string concatenation and the toInt()
method, you now have a range of techniques at your disposal. Each method has its advantages, so feel free to choose the one that best fits your project’s needs. With these skills, you can effectively manage data representation in your Arduino applications, making your coding experience smoother and more efficient.
FAQ
-
How do I convert a string to an integer in Arduino?
You can use thetoInt()
method of the String class to convert a string to an integer. -
Is it safe to use the String class in Arduino?
While the String class is convenient, it can lead to memory fragmentation in low-memory environments. For critical applications, consider using character arrays. -
Can I convert integers to strings in different bases?
Yes, you can use theitoa()
function and specify the base you want to use for conversion. -
What is the maximum size of a string in Arduino?
The maximum size of a string in Arduino depends on the available memory. However, it’s generally recommended to keep strings short to avoid memory issues. -
Can I use string concatenation for conversions in Arduino?
Yes, you can concatenate an empty string with a String object to convert an integer to a string, although it’s less efficient than other methods.
Related Article - Arduino String
- Arduino strcmp Function
- Arduino Strcpy Function
- How to Concatenate Strings in Arduino
- How to Parse a String in Arduino
- How to Split String in Arduino
- How to Compare Strings in Arduino