How to Convert Char to Int in Arduino
-
Use the
atoi()
Function to Convertchar
toint
in Arduino -
Use ASCII Values to Convert
char
toint
in Arduino -
Use Type Casting to Convert
char
toint
in Arduino -
Use the
toInt()
Function to Convertchar
toint
in Arduino -
Use the
sscanf()
Function to Convertchar
toint
in Arduino -
Use the
Serial.parseInt()
Function to Convertchar
toint
in Arduino - Conclusion
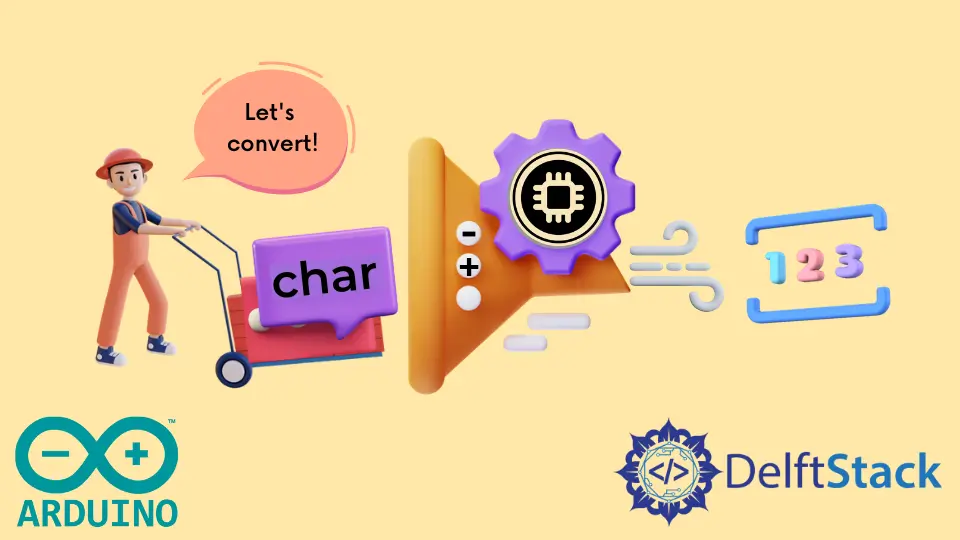
Converting a char
to an int
is common in Arduino programming. It allows you to manipulate and perform mathematical operations on character data.
This tutorial will discuss six methods to convert a char
into an int
in Arduino.
Use the atoi()
Function to Convert char
to int
in Arduino
The atoi()
function is a standard C library function that converts a string (character array) containing numerical representation into its integer equivalent.
void setup() {
Serial.begin(9600);
char charValue[] = "1234";
int intValue = atoi(charValue);
Serial.println(intValue);
}
void loop() {
// Your code here
}
void setup() { ... }
: This is thesetup
function, where you initialize your code. We start the serial communication at a baud rate of9600
.char charValue[] = "1234";
: This line initializes a character arraycharValue
with the value"1234"
.int intValue = atoi(charValue);
: Here, we use theatoi()
function to convert the character arraycharValue
to an integerintValue
.Serial.println(intValue);
: This line prints the converted integer value to the serial monitor.
Output:
1234
Use ASCII Values to Convert char
to int
in Arduino
This method can only convert a single char
into an int
. You must subtract a zero of type char
from the char
to convert it into an int
.
This method exploits the ASCII values to convert characters to integers.
void setup() {
Serial.begin(9600);
char charValue = '5';
int intValue = charValue - '0';
Serial.println(intValue);
}
void loop() {
// Your code here
}
void setup() { ... }
: Similar to the previous method, this is thesetup
function where we initialize serial communication.char charValue = '5';
: This line initializes a character variablecharValue
with the value'5'
.int intValue = charValue - '0';
: Here, we subtract the ASCII value of'0'
from the ASCII value ofcharValue
. This converts the character'5'
to its integer value5
.Serial.println(intValue);
: This line prints the converted integer value to the serial monitor.
Output:
5
Use Type Casting to Convert char
to int
in Arduino
Type casting involves explicitly changing the data type of a variable. In this case, we cast a character to an integer.
void setup() {
Serial.begin(9600);
char charValue = '7';
int intValue = int(charValue - '0');
Serial.println(intValue);
}
void loop() {
// Your code here
}
void setup() { ... }
: As before, this is thesetup
function where we initialize serial communication.char charValue = '7';
: This line initializes a character variablecharValue
with the value'7'
.int intValue = int(charValue - '0');
: Here, we use type casting to convert the result ofcharValue - '0'
to an integer.Serial.println(intValue);
: This line prints the converted integer value to the serial monitor.
Output:
7
Use the toInt()
Function to Convert char
to int
in Arduino
To use the toInt()
function to convert a char
to an int
in Arduino, you’ll first need to convert the char
to a String
and then use the toInt()
function.
void setup() {
Serial.begin(9600);
char charValue = '7';
String stringValue(charValue);
int intValue = stringValue.toInt();
Serial.println(intValue);
}
void loop() {
// Your code here
}
void setup() { ... }
: This is thesetup
function where we initialize serial communication.char charValue = '7';
: This line initializes a character variablecharValue
with the value'7'
.String stringValue(charValue);
: Here, we create aString
object namedstringValue
by passing thecharValue
to the constructor. This converts thechar
to aString
.int intValue = stringValue.toInt();
: We then use thetoInt()
function to convert theString
object to an integer. The resulting integer value is stored inintValue
.Serial.println(intValue);
: This line prints the converted integer value to the serial monitor.
Output:
7
Use the sscanf()
Function to Convert char
to int
in Arduino
The sscanf()
function reads the buffered data based on the specified format.
Syntax:
sscanf(char *data, char *format, [&var1, &var2, ... ]);
Where:
*data
is the pointer to the array.*format
is the sequence of characters specifying how thedata
shall be interpreted.&var1,...
is the additional argument specifying the location to save the extracted data.
We can use the below code to read the char
to int
.
sscanf(someChar, "%d", &result);
Here, %d
specifies that the char
shall be read as a signed integer decimal.
The complete Arduino code to convert char
to int
is below.
void setup() {
Serial.begin(9600); /*Serial Communication begins*/
char *someChar = "50";
int result = 0;
sscanf(someChar, "%d", &result);
Serial.println(result);
}
void loop() {}
Output:
50
Use the Serial.parseInt()
Function to Convert char
to int
in Arduino
You can use this method if you are reading input from a serial port of an Arduino and want to convert the received input into an Int
.
void loop() {
if (Serial.available() > 0) {
int valA = Serial.parseInt();
}
}
Parsing will stop if no value has been read or a non-digit is read. If no valid input is read until timeout, then 0
will be returned.
See Serial.setTimeout()
to set the timeout of the serial. Check this link for more information about the Serial.parseInt()
function.
Conclusion
In this tutorial, we’ve explored six methods to convert a char
to an int
in Arduino. Each method has its advantages and use cases, so choose the one that best suits your application.
Understanding these methods will give you greater flexibility in handling character data in your Arduino projects.