Arduino sscanf() Function
-
Understanding the Arduino
sscanf()
Function - Parameters and Format Specifiers
- Example Usage
- Error Handling
- Benefits and Considerations
- Conclusion
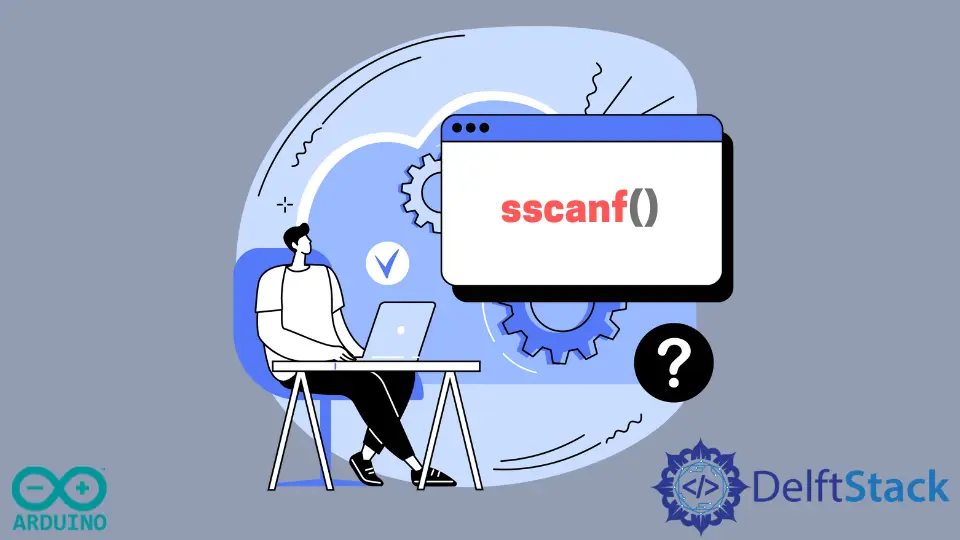
The sscanf()
function within Arduino is a powerful tool for parsing and extracting data from strings. Understanding its functionality can significantly enhance your ability to handle incoming data in a structured manner.
Here’s an in-depth exploration of the sscanf()
function and its application in Arduino programming. This tutorial will discuss reading formatted data from a character array or string using the sscanf()
function in Arduino.
Understanding the Arduino sscanf()
Function
The sscanf()
function in Arduino is derived from the standard C library function scanf()
. Its primary purpose is to parse formatted strings, similar to scanf()
, but instead of reading from the input stream (like keyboard input), sscanf()
extracts data from a specified string.
We can save numbers, symbols, characters, and other data inside a text or string file. We can use the sscanf()
function to read the data in a specific format from a text, string, or character array.
The basic syntax of the sscanf()
function is below
sscanf(inputString, format, arg1, arg2, ...);
inputString
: The string from which data needs to be extracted.format
: A format string that determines how the data should be parsed.arg1, arg2, ...
: Variables where the extracted data will be stored.
In the above syntax, inputString
contains the given string or character array we want to read using the format
defined in the second argument. After defining the format
, we can list the number of variables we want to store the formatted data.
Parameters and Format Specifiers
The format
parameter in sscanf()
consists of format specifiers that define the expected data types and structure of the input string. Here are some commonly used format specifiers:
%d
: Represents an integer.%f
: Denotes a floating-point number.%c
: Indicates a single character.%s
: Represents a string of characters.%x
: Denotes a hexadecimal number.%[^delimiter]
: Reads a string until it encounters the specified delimiter.
Example Usage
For example, consider we have a string that contains the date of an event, and we want to separate the date, month, and year and store them in separate variables. See the code below:
char *data = "28 April 2022";
int date = 0;
int year = 0;
char month[10];
void setup() {
Serial.begin(9600);
Serial.println(data);
sscanf(data, "%2d %s %4d", &date, month, &year);
Serial.println(date);
Serial.println(month);
Serial.println(year);
}
void loop() {}
Output:
28 April 2022
28
April
2022
We created the data
variable to store the given string in the above code. The date
, year
, and month
variables store day, year, and month values separately.
We initialized the month
variable with a size of 10, but we can change its size according to the given string that we want to save in the variable. We used the Serial.begin()
function to initialize the serial monitor of Arduino, which we will use to display the result.
To get an integer value, we use the %d
symbol, and the number 2
with it defines how many numbers we want to read. We use the %s
symbol to get a string, which will return the characters until white space.
If there is no space in the given string, we can use the %c
and a number to specify how many characters we want to read. We cannot use %s
because there is no white space, which will not return the expected result.
After the format, to save the result in an integer, we have to use the &
symbol, and for a string or character array, we only have to use the variable name. In the above example, we used the white space as the delimiter to format the data, but we can also use any character, symbol, or number.
Comma as Delimiter to Format the Data
In this example, consider if we have a comma in place of the white space inside the date string.
To extract the required result, we have to use the comma inside the sscanf()
function in place of the white space. We also have to use the %c
symbol instead of the %s
symbol because there is no white space present in the string.
For example, let’s repeat the above example with a different delimiter. See the code below:
char *data = "28,April,2022";
int date = 0;
int year = 0;
char month[10];
void setup() {
Serial.begin(9600);
Serial.println(data);
sscanf(data, "%2d,%5c,%4d", &date, month, &year);
Serial.println(date);
Serial.println(month);
Serial.println(year);
}
void loop() {}
Output:
28,April,2022
28
April
2022
We use the %5c
symbol inside the sscanf()
function in the above code because we only want to read five characters. If we read more than five characters, the next output will change.
As we can see, the output is the same as the output of the first example. We can also use other symbols for formatting the data, like %f
for floating-point numbers, %o
for octal numbers, %x
for hexadecimal numbers, and %u
for an unsigned integer.
Error Handling
It’s crucial to handle potential errors when using sscanf()
. If the input string does not match the specified format, sscanf()
might fail to extract data correctly.
Checking the return value of sscanf()
helps identify parsing errors.
int parsedItems = sscanf(input, format, arg1, arg2, ...);
if (parsedItems != expectedItems) {
// Handle parsing error
}
Here’s an example of how you can use sscanf
in Arduino to parse a string containing space-separated integers and handle parsing errors:
void setup() {
Serial.begin(9600);
// Simulated input string containing space-separated integers
char input[] = "10 20 30";
int num1, num2, num3;
// Using sscanf to parse the input string
int numParsed = sscanf(input, "%d %d %d", &num1, &num2, &num3);
if (numParsed == 3) {
Serial.print("Parsed values: ");
Serial.print(num1);
Serial.print(", ");
Serial.print(num2);
Serial.print(", ");
Serial.println(num3);
} else {
// Handle parsing error
Serial.println("Failed to parse the input string.");
}
}
void loop() {
// Nothing in the loop for this example
}
Output:
Parsed values: 10, 20, 30
This example demonstrates how to use sscanf
to parse a string containing three integers separated by spaces ("10 20 30"
).
The %d
format specifier is used to indicate integers and the values are stored in num1
, num2
, and num3
variables, respectively. Adjust the input string and format specifier according to your data format.
Benefits and Considerations
- Versatility:
sscanf()
enables parsing of complex data structures from strings. - Efficiency: It’s a powerful tool for extracting specific information without manually iterating through characters.
- Format String Precision: Correctly defining the format string is crucial for accurate data extraction.
Conclusion
Key points to remember about sscanf()
:
- Usage: It follows a specific syntax:
sscanf(inputString, format, arg1, arg2, ...)
, whereinputString
contains the data to parse,format
defines the expected structure, andarg1
,arg2
, etc., store the extracted data. - Format Specifiers: The
format
parameter utilizes format specifiers like%d
,%f
,%c
,%s
, and more, defining the data types and structure within the input string. - Parsing Examples:
sscanf()
is incredibly versatile. For instance, it can extract dates, separate delimited data, or even retrieve numeric values from sensor readings by carefully specifying the format string. - Error Handling: Properly handling parsing errors is essential. Mismatched format strings or unexpected data in the input string can lead to parsing errors, necessitating vigilant error handling.
- Benefits: Its versatility, efficiency, and ability to extract specific information without manual iteration through characters make
sscanf()
invaluable for structured data extraction in Arduino programming.
In essence, mastering the sscanf()
function elevates your ability to efficiently extract structured data from strings in Arduino projects, facilitating smoother communication between devices and enabling more robust data processing capabilities.