Arduino millis() Function
-
Understanding the
millis()
Function - Practical Applications of millis()
- Managing Multiple Timers with millis()
- Conclusion
- FAQ
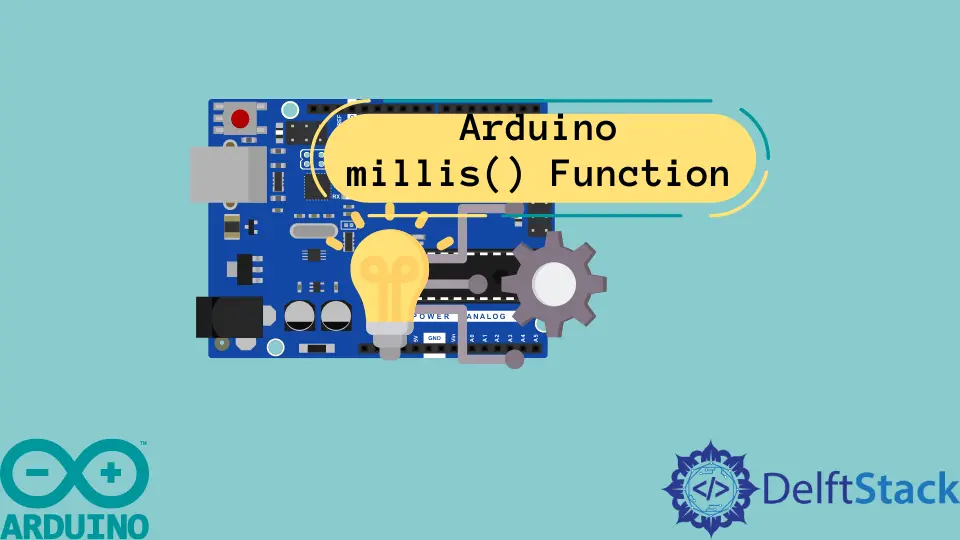
The Arduino millis() function is a powerful tool for anyone looking to track time in their projects. By using this function, you can easily determine how long it has been since your Arduino board began executing its code. This feature is particularly useful for creating non-blocking delays, managing time-based events, and enhancing the overall functionality of your projects.
In this article, we will dive deep into the millis()
function, explore its applications, and provide clear examples to help you understand how to implement it effectively. Whether you’re a beginner or a seasoned Arduino enthusiast, you’ll find valuable insights that can elevate your coding skills and project designs.
Understanding the millis()
Function
the millis()
function returns the number of milliseconds since the Arduino board began running the current program. This value is stored as an unsigned long, which means it can count up to about 50 days before it rolls over to zero. This characteristic makes millis() a reliable choice for timing events without blocking other processes, unlike the delay()
function, which halts all operations.
To use millis() effectively, you typically compare its current value to a previously recorded timestamp. This allows you to execute code based on elapsed time. For example, you can turn on an LED for a specific duration or trigger an event every few seconds without freezing your program. You can also learn about How to Make a Counter in Arduino to further enhance your projects by integrating time and counter functionalities.
Here’s a simple example of using the millis()
function to blink an LED:
const int ledPin = 13;
unsigned long previousMillis = 0;
const long interval = 1000;
void setup() {
pinMode(ledPin, OUTPUT);
}
void loop() {
unsigned long currentMillis = millis();
if (currentMillis - previousMillis >= interval) {
previousMillis = currentMillis;
digitalWrite(ledPin, !digitalRead(ledPin));
}
}
In this example, we set up an LED to blink every second. The previousMillis
variable stores the last time the LED state was changed. The currentMillis
variable captures the current time using millis(). If the difference between these two values exceeds the defined interval, the LED state toggles.
Output:
LED blinks every second
This simple yet effective approach demonstrates how you can leverage the millis()
function to create time-based actions without interrupting the flow of your program.
Practical Applications of millis()
the millis()
function is versatile and can be applied in various scenarios. One of the most common uses is for creating timers. For instance, you can use millis() to send data at regular intervals, control motors, or manage sensor readings without blocking the main loop of your Arduino sketch. You might find functions like the Arduino memset() Function useful in managing memory when working on these tasks.
Consider a scenario where you want to read a temperature sensor every two seconds and display the readings. Here’s how you can achieve this using millis():
const int sensorPin = A0;
unsigned long previousMillis = 0;
const long interval = 2000;
void setup() {
Serial.begin(9600);
}
void loop() {
unsigned long currentMillis = millis();
if (currentMillis - previousMillis >= interval) {
previousMillis = currentMillis;
int sensorValue = analogRead(sensorPin);
Serial.println(sensorValue);
}
}
In this sketch, we read an analog temperature sensor every two seconds. The previousMillis
variable tracks the last time we performed a reading. When the elapsed time exceeds the interval, we read the sensor and print the value to the Serial Monitor.
Output:
Sensor value output every 2 seconds
This method allows you to maintain a responsive program while continuously gathering data from the sensor, showcasing the efficiency of the millis()
function.
Managing Multiple Timers with millis()
As your projects grow in complexity, you may need to manage multiple timers simultaneously. the millis()
function allows you to do just that without the need for complex timing libraries. By using arrays or structures, you can keep track of various events and their corresponding intervals.
Here’s an example where we manage two LEDs blinking at different intervals:
const int ledPin1 = 13;
const int ledPin2 = 12;
unsigned long previousMillis1 = 0;
unsigned long previousMillis2 = 0;
const long interval1 = 1000;
const long interval2 = 500;
void setup() {
pinMode(ledPin1, OUTPUT);
pinMode(ledPin2, OUTPUT);
}
void loop() {
unsigned long currentMillis = millis();
if (currentMillis - previousMillis1 >= interval1) {
previousMillis1 = currentMillis;
digitalWrite(ledPin1, !digitalRead(ledPin1));
}
if (currentMillis - previousMillis2 >= interval2) {
previousMillis2 = currentMillis;
digitalWrite(ledPin2, !digitalRead(ledPin2));
}
}
In this code, we control two LEDs, one blinking every second and the other every half second. Each LED has its own timing logic, allowing them to operate independently. This showcases the flexibility of the millis()
function in managing multiple tasks concurrently. Understanding how to leverage functions like the Arduino printf Function can further assist in debugging and monitoring these concurrent tasks.
Output:
LED1 blinks every second, LED2 blinks every half second
By organizing your code this way, you can create intricate systems that respond to multiple events without sacrificing performance.
Conclusion
the millis()
function is an essential tool for every Arduino programmer. It opens up a world of possibilities for time tracking, event management, and creating responsive applications. By understanding how to use millis(), you can significantly enhance your projects, making them more efficient and versatile. Whether you’re blinking LEDs, reading sensors, or managing multiple tasks, millis() is your go-to function for non-blocking timing operations. Embrace this powerful feature and watch your Arduino projects flourish!
FAQ
-
What is the
millis()
function in Arduino?
themillis()
function returns the number of milliseconds since the Arduino board started running the current program. -
How does millis() differ from delay()?
Unlike delay(), which halts all operations, millis() allows your program to continue running while tracking time. -
Can millis() be used for multiple timers?
Yes, you can manage multiple timers simultaneously using millis() by storing different timestamps for each event. -
What happens when millis() reaches its maximum value?
When it reaches its maximum value (approximately 50 days), it rolls over to zero. -
Is millis() suitable for precise timing applications?
While millis() is useful for most applications, for very precise timing, consider using themicros()
function, which measures time in microseconds.