Arduino printf Function
- Understanding the Arduino Environment
- Using sprintf for String Formatting
- Combining Serial.print for Output
- Advantages of Using sprintf and Serial.print
- Conclusion
- FAQ
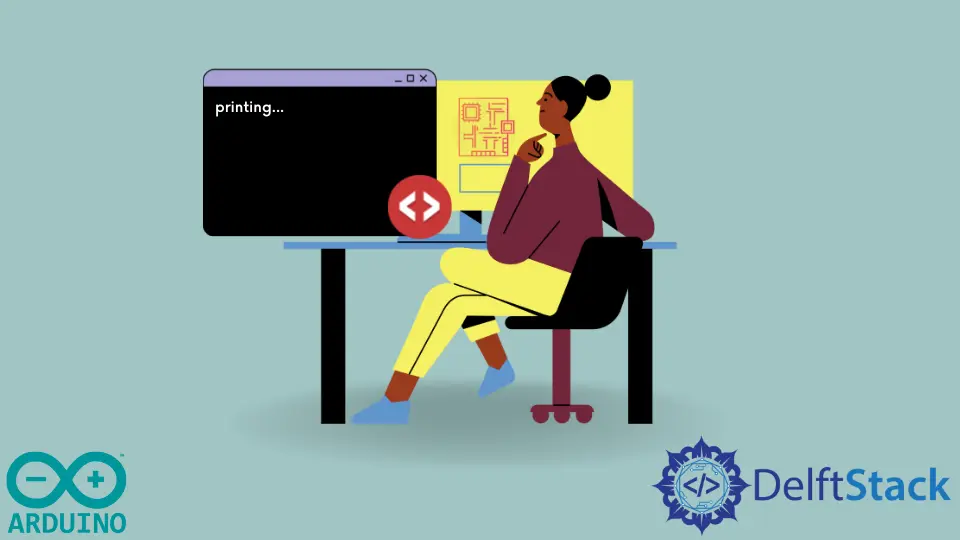
When working with Arduino, one might often miss the convenience of the printf function found in C/C++. This powerful function allows developers to format strings easily, making debugging and data output much more manageable. However, in the Arduino environment, the standard printf function is not directly available. Instead, you can achieve similar results using the sprintf function along with Serial.print.
This article will guide you through these methods, providing clear examples and explanations to help you utilize these functions effectively in your Arduino projects. Whether you’re a beginner or an experienced developer, understanding how to format output can significantly enhance your coding experience on the Arduino platform.
Understanding the Arduino Environment
Before diving into the specifics of the sprintf and Serial.print functions, it’s essential to grasp the Arduino environment’s limitations and capabilities. The Arduino platform is designed for simplicity and ease of use, which sometimes means sacrificing certain features found in standard C/C++ libraries. The printf function, while incredibly useful, isn’t included by default in the Arduino IDE. This limitation can be a hurdle for developers accustomed to the flexibility of printf for formatted output. However, the sprintf function, which formats strings and stores them in a character array, can be a powerful alternative when combined with Serial.print for output.
Using sprintf for String Formatting
One of the most effective ways to mimic the behavior of printf in Arduino is through the use of the sprintf function. This function allows you to create formatted strings, which can then be printed to the serial monitor. Here’s how to use it:
char buffer[50];
int value = 42;
sprintf(buffer, "The answer is: %d", value);
Serial.begin(9600);
Serial.println(buffer);
Output:
The answer is: 42
In this example, we first declare a character array named buffer
to hold our formatted string. The sprintf
function then formats the string, replacing the %d
placeholder with the value of the value
variable. After initializing the serial communication with Serial.begin(9600)
, we print the contents of buffer
to the serial monitor using Serial.println(buffer)
. This method not only provides a clear and formatted output but also allows for complex string manipulations, making it a valuable tool in your Arduino programming arsenal.
Combining Serial.print for Output
While sprintf
prepares the formatted string, the Serial.print
function is responsible for sending that string to the serial monitor. This combination is particularly useful for debugging or displaying information in a user-friendly format. Here’s a practical example:
int temperature = 25;
float humidity = 60.5;
char output[100];
sprintf(output, "Temperature: %d°C, Humidity: %.1f%%", temperature, humidity);
Serial.begin(9600);
Serial.println(output);
Output:
Temperature: 25°C, Humidity: 60.5%
In this code snippet, we define two variables, temperature
and humidity
, to hold our data. We then create a character array named output
to store the formatted string. The sprintf
function formats the string with both integer and floating-point values, replacing the placeholders with actual data. Finally, we print the formatted string to the serial monitor, allowing us to easily read and understand the output. This method is particularly effective for monitoring sensor data in real-time applications.
Advantages of Using sprintf and Serial.print
The combination of sprintf
and Serial.print
offers several advantages for Arduino developers. First, it allows for cleaner and more organized code, making it easier to read and maintain. Instead of concatenating strings manually, you can format them in a single line, reducing the chances of errors. Additionally, this method enhances the clarity of your output, especially when dealing with multiple variables or complex data types.
Moreover, using sprintf
can significantly reduce the amount of code you need to write, streamlining your development process. It also enables you to format strings in various ways, such as controlling the number of decimal places for floating-point numbers or padding integers with leading zeros. These features make it an invaluable tool for any Arduino programmer looking to improve their output formatting.
Conclusion
In summary, while the Arduino environment does not provide the printf function directly, you can achieve similar results using the sprintf and Serial.print functions. This combination allows for flexible and formatted output, making it easier to display data and debug your projects. By mastering these functions, you can enhance your coding skills and create more sophisticated Arduino applications. Whether you’re monitoring sensor data or debugging your code, understanding how to format strings effectively will undoubtedly improve your programming experience.
FAQ
-
What is the main purpose of the sprintf function in Arduino?
The sprintf function is used to format strings and store them in a character array, allowing for organized and readable output. -
Can I use printf directly in Arduino?
No, the printf function is not available in the standard Arduino environment, but you can achieve similar results with sprintf and Serial.print. -
How do I print floating-point numbers using sprintf?
You can use format specifiers like %.1f to control the number of decimal places when printing floating-point numbers. -
Is there a limit to the size of the character array used with sprintf?
Yes, you should allocate enough space in the character array to hold the formatted string; otherwise, it may lead to buffer overflow. -
How can I debug my Arduino code using Serial.print?
You can use Serial.print to output variable values and messages to the serial monitor, helping you identify issues in your code.