Arduino memset() Function
-
Introduction to the
memset()
Function in Arduino -
Use the
memset()
Function in Arduino - Important Considerations
- Conclusion
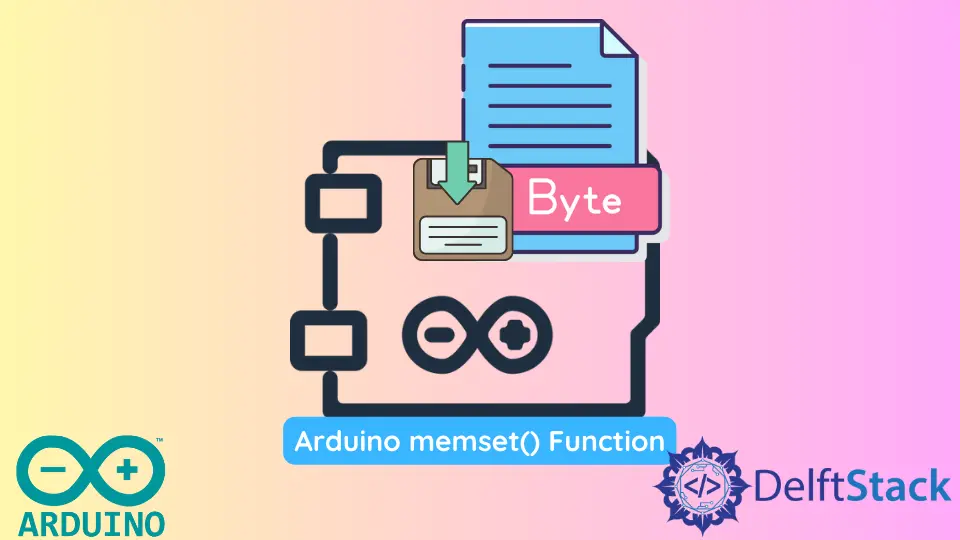
When it comes to working with microcontrollers like Arduino, memory management is crucial. The memset()
function is a powerful tool that allows you to set a block of memory to a specific value efficiently.
This tutorial will delve into the intricacies of the memset()
function in Arduino, providing you with a solid understanding of its usage and applications.
Introduction to the memset()
Function in Arduino
The memset()
function, short for “memory set”, is a standard C library function that allows you to set a block of memory to a specified value. It’s particularly useful for initializing arrays, buffers, and other data structures.
In Arduino, which is based on the C/C++ programming language, memset()
is a versatile tool for memory management.
The memset()
function sets the bytes of a value to the destination in Arduino. The function takes a specific number of bytes from a given value and stores it in the destination.
Syntax:
memset(destination, value, N_bytes);
In the above syntax, the memset()
function will take the first N_bytes
of the given value
and store it inside the destination
. If the value
is in integer or any other data type, it will be converted to byte data type.
Use the memset()
Function in Arduino
We’ll create an array of integers and change its values using the memset()
function.
int ch1[4] = {1, 2, 3, 4};
void setup() {
Serial.begin(9600);
memset(ch1, 2, 4 * sizeof(ch1[1]));
for (int i = 0; i < 4; i++) {
Serial.println(ch1[i]);
}
}
void loop() {}
Output:
514
514
514
514
We defined an array of integers and used the memset()
function to replace all four elements with the bytes of integer 2
. We used a loop to print the array’s values on the serial monitor window of Arduino IDE.
We can also convert the byte values to an integer using the int()
function. We must store each array value to a byte variable and then pass it inside the int()
function to convert it into an integer.
Let’s convert the above array value to an integer. Look at the code below.
int ch1[4] = {1, 2, 3, 4};
void setup() {
Serial.begin(9600);
memset(ch1, 2, 4 * sizeof(ch1[1]));
for (int i = 0; i < 4; i++) {
byte b = ch1[i];
int c = int(b);
Serial.println(c);
}
}
void loop() {}
Output:
2
2
2
2
The byte value has been converted to integers, but they are not stored inside the original array. We can also do the same operation with strings and characters using the memset()
function.
We can define an array of characters and replace them with another character using the memset()
function. After using the memset()
function, we will get an array that will contain the byte value of the characters, but we can convert the value back to a character using the char()
function.
We must store each byte value in a byte
variable and then pass it inside the char()
function to convert it into a character. Let’s define an array of integers, store character values, and then replace them with another.
int ch1[4] = {'a', 'b', 'c', 'd'};
void setup() {
Serial.begin(9600);
memset(ch1, '.', 4 * sizeof(ch1[1]));
for (int i = 0; i < 4; i++) {
byte b = ch1[i];
char c = char(b);
Serial.println(c);
}
}
void loop() {}
Output:
.
.
.
.
In the third argument, the integer that we multiply defines the number of values we want to replace inside the array. If we don’t want to replace all the values and we want to replace only some values of the array, we have to add an integer in the first argument of the memset()
function.
Suppose we only want to replace the second and third values of the given array. We must add 1
in the first argument and multiply it by 2
in the third argument, as shown in the code below.
int ch1[4] = {'a', 'b', 'c', 'd'};
void setup() {
Serial.begin(9600);
memset(ch1 + 1, '.', 2 * sizeof(ch1[1]));
for (int i = 0; i < 4; i++) {
byte b = ch1[i];
char c = char(b);
Serial.println(c);
}
}
void loop() {}
Output:
a
.
.
d
Only the middle elements of the array are replaced. We can also store values inside an array using a loop.
In the following example, let’s do the above operation using a loop in Arduino.
char ch1[4] = {'a', 'b', 'c', 'd'};
void setup() {
Serial.begin(9600);
for (int i = 1; i < 3; i++) {
ch1[i] = '.';
}
for (int i = 0; i < 4; i++) {
Serial.println(ch1[i]);
}
}
void loop() {}
Output:
a
.
.
d
In the above code, we defined the array as a char
data type, used a loop to replace the elements, and used another loop to print the array elements. If we use int
to declare the given array, the characters will be converted into ASCII representation.
We have to use a loop to print the values of an array, but we can print it without a loop in the case of a string. We can replace the characters present in a string using a loop.
We have to get the characters we want to replace using their index and then replace them using another character.
Let’s define a string and replace some of its characters with another. Look at the code below.
String ch1 = "hello world";
void setup() {
Serial.begin(9600);
for (int i = 1; i < 5; i++) {
ch1[i] = '.';
}
Serial.println(ch1);
}
void loop() {}
Output:
h.... world
Some characters of the given string are replaced with another character. In the case of the memset()
function, we defined the given array with the int
data type because the function does not work with other data type arrays.
Important Considerations
Use Carefully With Non-Pod Types
While memset()
is suitable for basic data types like integers and characters, it may not be suitable for more complex types or classes with constructors. In those cases, consider alternative initialization methods.
Beware of Overflows
Ensure that the length you specify does not exceed the actual size of the memory block. An overflow can lead to unintended consequences.
Mind the Data Type
Remember that memset()
works with bytes, so if you’re using it to set non-byte data types (like integers or floats), be aware that you’re setting the individual bytes.
Conclusion
In conclusion, mastering the memset()
function in Arduino provides a powerful tool for efficient memory management. This tutorial has provided a detailed understanding of its syntax, applications, and practical usage through various examples.
By applying memset()
, you can initialize arrays, clear buffers, and even set the initial state of data structures.
However, it’s important to be cautious with non-POD types and be mindful of potential overflows. Additionally, consider the data type you’re working with, as memset()
operates at the byte level.
With this knowledge, you’re well-equipped to leverage the capabilities of the memset()
function in your Arduino projects, enhancing your ability to manage memory effectively and create efficient microcontroller applications.