Arduino 2D Array
- 2D Arrays in Arduino
- Initialize 2D Arrays in Arduino
- Store Data in a 2D Array in Arduino
- Replace a Value in a Specific Position of a 2D Array in Arduino
- Retrieve a Value From a Specific Position in a 2D Array in Arduino
-
Arduino
MatrixMath
Library for 2D Arrays - Conclusion
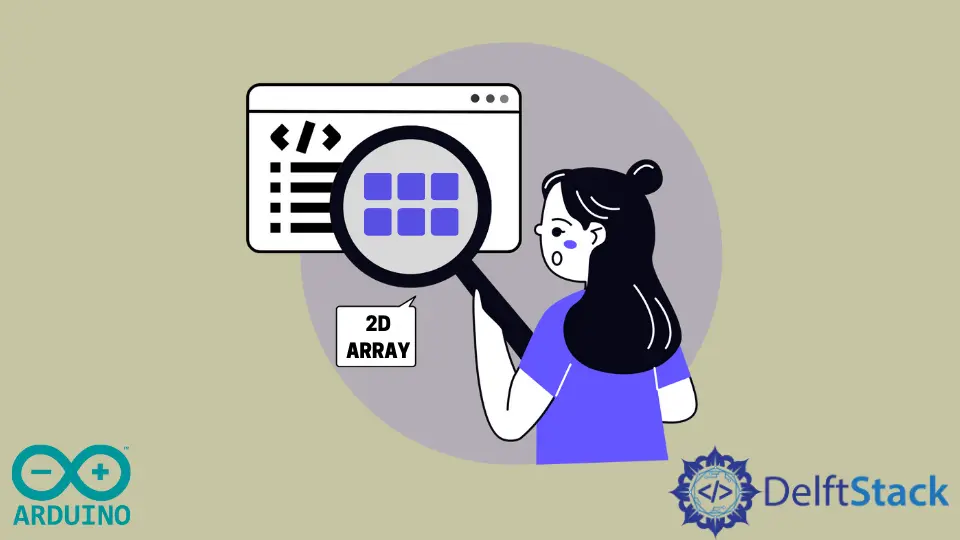
Arrays are fundamental data structures in programming that allow you to store and organize data efficiently. This guide will walk you through the process of initializing a 2D array in Arduino and demonstrate how to effectively use it to store and manipulate data.
2D Arrays in Arduino
In Arduino, a 2D array (two-dimensional array) is a data structure that allows you to store and organize data in a grid-like format with rows and columns. Think of it as a table with rows and columns, where each intersection point represents a unique piece of information.
This structure is an extension of the more common one-dimensional array, providing a way to represent and manipulate data in two dimensions.
In a 2D array, each element is identified by two indices: a row index and a column index. This dual indexing system allows you to access and manipulate data at a specific position within the grid.
The concept of a 2D array is especially useful when dealing with data that naturally fits into a tabular or grid structure, such as sensor readings, pixel values in an image, or game board states.
Initialize 2D Arrays in Arduino
In Arduino programming, the initialization of a 2D array follows a structure similar to that of a 1D array. However, due to the multidimensional nature of 2D arrays, an additional step involves specifying the number of rows and columns before populating the array with data.
Declare and Define the 2D Array
Imagine you have a grid like this:
| Column 0 | Column 1 | Column 2 |
----------------------------------------
Row 0 | 1 | 2 | 3 |
Row 1 | 4 | 5 | 6 |
Row 2 | 7 | 8 | 9 |
In Arduino, this grid can be represented using a 2D array. You will need to declare the array and specify the number of rows and columns.
Here’s how you might declare and initialize it:
void setup() {
int nRow = 3;
int nCol = 3;
int myArray[nRow][nCol] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
}
void loop() {
// main code goes here
}
In this example, nRow
and nCol
are integer variables indicating the number of rows and columns, respectively. The array myArray
is then initialized with specific integer values.
It is essential to note that the dimensions and data type of the array can be adjusted based on the specific requirements of the data you intend to store.
Variable Initialization and Modification
In the provided code snippet, nRow
and nCol
serve as variables, allowing for dynamic adjustments to the array dimensions. This flexibility is particularly useful when dealing with data of varying sizes or when you need to modify the array size during runtime.
You can modify these variables to match the dimensions of your specific use case.
int nRow = 3; // Change the number of rows to 3
int nCol = 5; // Change the number of columns to 5
This adaptability enables the seamless integration of 2D arrays into a wide range of Arduino applications.
While the example above uses an array of integers, it’s important to note that 2D arrays in Arduino can accommodate various data types. Whether dealing with integers, floating-point numbers, or even custom data structures, the versatility of 2D arrays makes them suitable for a diverse array of applications.
Store Data in a 2D Array in Arduino
Once a 2D array is initialized in Arduino, the next step is storing data within the array. Unlike 1D arrays, 2D arrays necessitate a more systematic approach due to their grid-like structure.
Use Loops for Data Storage
Given the multidimensional nature of a 2D array, manually assigning values to each position can be impractical and time-consuming. To streamline this process, loops are employed to systematically iterate through each row and column, storing data at every position.
The following example demonstrates this concept:
void setup() {
int data = 0;
int nRow = 2;
int nCol = 4;
int myArray[nRow][nCol];
for (int nr = 0; nr < nRow; nr++) {
for (int nc = 0; nc < nCol; nc++) {
myArray[nr][nc] = data++;
}
}
}
void loop() {
// main code goes here
}
In this code snippet, two loops, nr
for rows and nc
for columns, traverse each position within the 2D array. The variable data
is then systematically stored at every position, ensuring a sequential and efficient data storage process.
Dynamic Data Source
The value assigned to the array positions, represented by the variable data
in the example, can be dynamic and adapted based on the specific data source or requirements of your Arduino project. Whether the data source is sensor readings, user inputs, or any other dynamic information, the use of loops ensures a scalable and adaptable approach to populating the 2D array.
void setup() {
int data = 0;
int nRow = 2;
int nCol = 4;
int myArray[nRow][nCol];
int sensorReading = analogRead(A0); // Read sensor data dynamically
for (int nr = 0; nr < nRow; nr++) {
for (int nc = 0; nc < nCol; nc++) {
myArray[nr][nc] = sensorReading;
}
}
}
void loop() {
// main code goes here
}
The systematic use of loops not only enhances efficiency but also facilitates the organization of data within the 2D array. As the loops iterate through each position, the array becomes a structured repository for the collected information, making it readily accessible for subsequent operations or analysis.
Replace a Value in a Specific Position of a 2D Array in Arduino
In Arduino programming, scenarios may arise where it becomes necessary to replace or update the value at a specific position within a 2D array. Whether responding to user inputs or dynamic changes in the environment, efficiently modifying individual elements in a 2D array is a fundamental skill.
Using the Assignment Operator for Replacement
To replace a value at a specific position in a 2D array, the assignment operator (=
) is employed. The array indices for both the row and column are specified within square brackets, facilitating the precise identification of the element to be replaced.
Here’s an illustrative example:
void setup() {
int nRow = 2;
int nCol = 4;
int myArray[2][4] = {{1, 2, 3, 4}, {5, 6, 7, 8}};
// Replace the value at the specified position
myArray[1][2] =
42; // Replacing the value at the second row, third column with 42
}
void loop() {
// Your main code goes here
}
In this example, the value at the second row and third column of myArray
is replaced with the integer 42
. This targeted approach allows for precise updates within the 2D array without affecting other elements.
Dynamic Value Replacement
The value assigned for replacement need not be a constant; it can be dynamically determined based on real-time data or user inputs. This dynamic nature adds a layer of adaptability to your Arduino projects.
For instance:
void setup() {
int userInput = Serial.parseInt(); // Read user input dynamically
int targetRow = 1; // User-specified row index
int targetCol = 2; // User-specified column index
int myArray[2][4] = {{1, 2, 3, 4}, {5, 6, 7, 8}};
// Replace the value at the user-specified position
myArray[targetRow][targetCol] = userInput;
}
void loop() {
// Your main code goes here
}
In this scenario, the user can input a value, and based on the specified row and column indices, the corresponding element in the 2D array is replaced with the entered value.
Remember that when replacing values in a 2D array, it is important to ensure that the specified indices fall within the valid range of rows and columns. Accessing positions outside the array’s bounds may lead to unexpected behavior or errors.
Implementing appropriate checks, such as verifying user inputs or sensor data, enhances the robustness of your Arduino code.
Retrieve a Value From a Specific Position in a 2D Array in Arduino
The ability to retrieve a value from a specific position within a 2D array is also fundamental, particularly when analyzing or utilizing stored data.
To retrieve a value from a specific position in a 2D array, the assignment operator (=
) is again employed. Similar to the process of updating values, the row and column indices are specified within square brackets to pinpoint the desired element.
Here’s an example illustrating the retrieval process:
void setup() {
int nRow = 2;
int nCol = 4;
int myArray[nRow][nCol] = {{1, 2, 3, 4}, {5, 6, 7, 8}};
// Retrieve the value from the specified position
int retrievedValue =
myArray[1][2]; // Retrieving the value at the second row, third column
}
void loop() {
// Your main code goes here
}
In this example, the value at the second row and third column of myArray
is retrieved and stored in the variable retrievedValue
. This straightforward method allows for precise extraction of data from the 2D array.
Dynamic Retrieval Based on Conditions
The process of retrieving a value can also be dynamic, allowing for conditional retrieval based on certain criteria. For instance, you might want to retrieve a value only if it meets a specific condition.
Consider the following example:
void setup() {
int threshold = 5; // Define a threshold value
int targetRow = 0;
int targetCol = 2;
int nRow = 2;
int nCol = 4;
int myArray[2][4] = {{1, 2, 3, 4}, {5, 6, 7, 8}};
// Retrieve the value if it exceeds the defined threshold
int retrievedValue = (myArray[targetRow][targetCol] > threshold)
? myArray[targetRow][targetCol]
: -1;
// Print the retrieved value
Serial.begin(9600); // Initialize serial communication
Serial.print("Retrieved value at position (");
Serial.print(targetRow);
Serial.print(", ");
Serial.print(targetCol);
Serial.print("): ");
Serial.println(retrievedValue);
}
void loop() {
// Your main code goes here
}
Code Output:
Retrieved value at position (0, 2): 3
In this scenario, the value is retrieved only if it exceeds the specified threshold. If the condition is not met, a default value of -1
is assigned to the retrievedValue
.
Similar to value replacement, it is important to ensure that the specified row and column indices are within the valid range of the 2D array. Attempting to access positions outside the array’s bounds may result in unexpected behavior or errors.
Implementing proper bounds checking ensures the reliability of your Arduino code.
void setup() {
int nRow = 3;
int nCol = 3;
int myArray[3][3] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
int targetRow = 1;
int targetCol = 2;
if (targetRow >= 0 && targetRow < nRow && targetCol >= 0 &&
targetCol < nCol) {
// Valid indices, proceed with retrieval
int retrievedValue = myArray[targetRow][targetCol];
Serial.print("Retrieved value at position (");
Serial.print(targetRow);
Serial.print(", ");
Serial.print(targetCol);
Serial.print("): ");
Serial.println(retrievedValue);
} else {
// Invalid indices, handle the error, or provide default values
Serial.println("Invalid indices. Unable to retrieve value.");
}
}
void loop() {
// Main code goes here
}
Code Output:
Retrieved value at position (1, 2): 6
Here, the if
statement checks if the specified indices are within the valid range of the array (non-negative and less than the dimensions of the array). If the indices are valid, the code proceeds to retrieve the value at the specified position and prints the result to the Serial Monitor, including the indices.
In the event of invalid indices, an error message is printed to indicate that the retrieval is not possible. The code highlights the importance of checking index validity when working with arrays to prevent potential errors and improve code robustness.
Arduino MatrixMath
Library for 2D Arrays
The MatrixMath
library is designed to facilitate complex mathematical operations on matrices, which aligns seamlessly with the nature of 2D arrays. Matrices, in this context, refer to 2D arrays that can represent mathematical entities such as vectors or coordinate transformations.
The library simplifies the implementation of these operations, making it easier for Arduino developers to work with more advanced mathematical concepts. To use the MatrixMath
library in your Arduino project, you first need to include the library at the beginning of your sketch.
The following example demonstrates how to include the library and declare matrices for subsequent operations:
#include <MatrixMath.h>
#define N (3)
mtx_type X[N][N];
mtx_type Y[N][N];
mtx_type Z[N][N];
void setup() {
Serial.begin(9600);
// Initialize matrices
for (int i = 0; i < N; i++) {
for (int j = 0; j < N; j++) {
X[i][j] = i + j; // X is a matrix with sequential row and column sums
Y[i][j] = random(20); // Y is a random matrix with values up to 19
}
}
Serial.println("\nMatrix X:");
Matrix.Print((mtx_type*)X, N, N, "X");
Serial.println("\nMatrix Y:");
Matrix.Print((mtx_type*)Y, N, N, "Y");
// Matrix addition
Matrix.Add((mtx_type*)X, (mtx_type*)Y, N, N, (mtx_type*)Z);
Serial.println("\nZ = X + Y:");
Matrix.Print((mtx_type*)Z, N, N, "Z");
// Matrix subtraction
Matrix.Subtract((mtx_type*)X, (mtx_type*)Y, N, N, (mtx_type*)Z);
Serial.println("\nZ = X - Y:");
Matrix.Print((mtx_type*)Z, N, N, "Z");
}
void loop() {
// Main code goes here
}