How to Print Char Array in Arduino
- Understanding Char Arrays in Arduino
- Method 1: Using a Simple Loop to Print Char Array
- Method 2: Using the Serial.print() Function Directly
- Method 3: Using the String Class for More Flexibility
- Conclusion
- FAQ
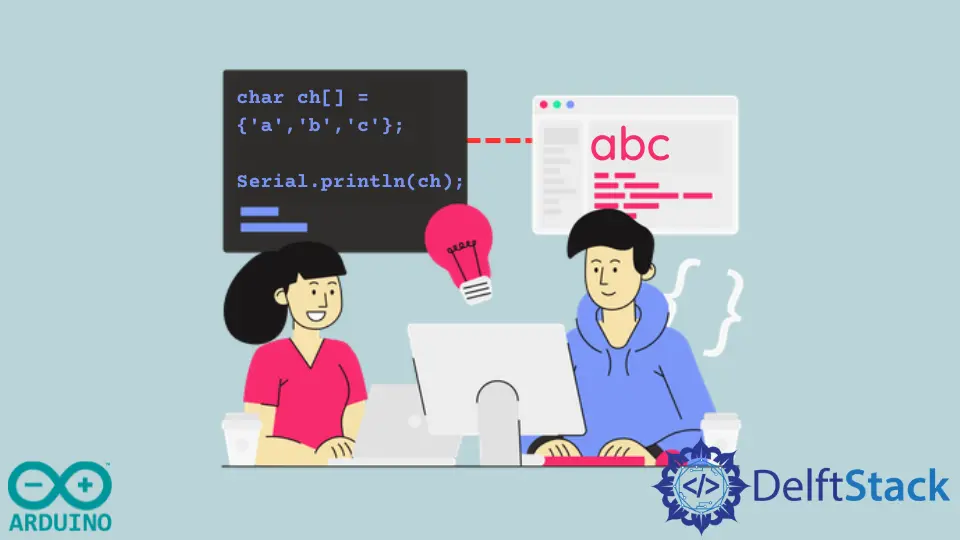
Printing a char array in Arduino can seem daunting for beginners, but it’s a straightforward task once you grasp the basics. Char arrays are essential for handling strings in Arduino programming. Whether you’re displaying a message on an LCD or sending data to the Serial Monitor, knowing how to print char arrays effectively is crucial.
In this article, we’ll explore different methods to print char arrays using loops in Arduino. We will provide clear examples and explanations to help you understand the process better. So, if you’re ready to enhance your Arduino skills, let’s dive into the world of char arrays!
Understanding Char Arrays in Arduino
Before we jump into printing char arrays, it’s essential to understand what they are. A char array is essentially a collection of characters stored in contiguous memory locations. In Arduino, they are often used to represent strings. For example, a char array can hold a word or a sentence, making it a versatile tool for various applications.
In Arduino, you can declare a char array like this:
char myArray[] = "Hello, Arduino!";
This line creates a char array named myArray
that contains the string “Hello, Arduino!”. The beauty of using char arrays lies in their ability to be manipulated easily. You can loop through the array to print each character, making it an excellent choice for displaying messages.
Method 1: Using a Simple Loop to Print Char Array
One of the most straightforward methods to print a char array in Arduino is by using a simple loop. This method iterates through each character in the array until it reaches the null terminator, which indicates the end of the string. Let’s take a look at the code.
char myArray[] = "Hello, Arduino!";
void setup() {
Serial.begin(9600);
for (int i = 0; myArray[i] != '\0'; i++) {
Serial.print(myArray[i]);
}
}
void loop() {
// Nothing here
}
Output:
Hello, Arduino!
In this code snippet, we first define a char array named myArray
containing the text “Hello, Arduino!”. In the setup()
function, we initialize the Serial communication at a baud rate of 9600. The for
loop iterates over each character in myArray
, printing each character to the Serial Monitor until it encounters the null terminator ('\0'
). This simple method effectively prints the entire char array as a string.
Method 2: Using the Serial.print() Function Directly
Another efficient way to print a char array is to use the Serial.print()
function directly. This method is particularly useful when you want to print the entire array without explicitly looping through each character. Here’s how you can do it:
char myArray[] = "Hello, Arduino!";
void setup() {
Serial.begin(9600);
Serial.print(myArray);
}
void loop() {
// Nothing here
}
Output:
Hello, Arduino!
In this example, we again declare our char array myArray
. Inside the setup()
function, we begin Serial communication and use Serial.print(myArray)
to print the entire array in one go. This method is cleaner and more efficient when you want to output the entire string without the need for a loop. It’s a great option for quick debugging or displaying messages.
Method 3: Using the String Class for More Flexibility
While char arrays are useful, the String class in Arduino provides more flexibility and functionality for handling strings. If you want to work with char arrays but need the additional features of the String class, you can easily convert a char array to a String object. Here’s an example:
char myArray[] = "Hello, Arduino!";
void setup() {
Serial.begin(9600);
String myString = String(myArray);
Serial.print(myString);
}
void loop() {
// Nothing here
}
Output:
Hello, Arduino!
In this code, we convert the char array myArray
into a String object named myString
. This conversion allows us to utilize the various methods available in the String class, such as concatenation, substring extraction, and more. After converting, we print myString
to the Serial Monitor. This method is particularly beneficial when you need to manipulate the string further after printing.
Conclusion
Printing a char array in Arduino is a fundamental skill that can significantly enhance your programming capabilities. Whether you choose to use a simple loop, the Serial.print()
function, or the String class, each method offers its unique advantages. As you continue to explore the world of Arduino, mastering these techniques will help you create more interactive and dynamic projects. So, grab your Arduino board, try out these methods, and watch your projects come to life!
FAQ
-
How do I declare a char array in Arduino?
You can declare a char array using the syntax:char myArray[] = "Your String";
-
What is the purpose of the null terminator in a char array?
The null terminator ('\0'
) indicates the end of the string, allowing functions to know when to stop reading the array. -
Can I modify the contents of a char array in Arduino?
Yes, you can modify the contents of a char array by accessing individual elements using their index. -
What is the advantage of using the String class over char arrays?
The String class provides more flexibility and built-in methods for string manipulation, making it easier to work with strings. -
How can I print multiple char arrays in Arduino?
You can print multiple char arrays by using separateSerial.print()
statements for each array.